Question
12) How many recursive calls to the fib method shown below would be made from an original call to fib(4)? (Do not count the original
12) How many recursive calls to the fib method shown below would be made from an original call to fib(4)? (Do not count the original call)
public int fib(int n)
{ // assumes n >= 0
if (n <= 1)
{
return n
}
else
{
return (fib(n - 1) + fib(n - 2));
}
}
a) 1
b) 2
c) 4
d) 8
13) Consider the method below, which implements the exponentiation operation recursively. Select the statement that should be used to complete the method, so that it handles the special case correctly.
public static double power(int base, int exponent)
{
if (exponent == 0)
{
_______________
}
else
{
reurn base * power(base, exponent 1);
}
}
a) return 1;
b) return base;
c) return 0;
d) return 1 * power(base, exponent 1);
14) Consider the code for the recursive method printSum shown in this code snippet, which is intended to return the sum of digits from 1 to n:
public static int printSum(int n)
{
if (n <= 0) // line #1
{
return 0; // line #
}
else
{
return (n + printSum(n)); //line #3
}
}
Which of the following statements is correct?
a) line #1 is incorrect, and should be changed to if (n <= 1)
b) line #3 is incorrect, and should be changed to return (printSum (n - 1));
c) line #3 is incorrect, and should be changed to return (n + printSum (n + 1));
d) line #3 is incorrect, and should be changed to return (n + printSum (n - 1));
Step by Step Solution
There are 3 Steps involved in it
Step: 1
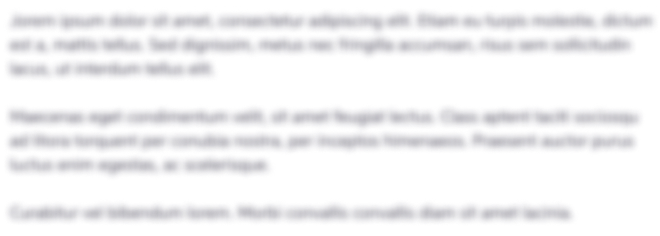
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started