Question
12.8 (LAB) Fun With Branching - FINAL For this project, you will write a Java program that will run a simple math quiz. Your program
12.8 (LAB) Fun With Branching - FINAL
For this project, you will write a Java program that will run a simple math quiz. Your program will generate two random integers between 1 and 20 and then ask a series of math questions. Each question will be evaluated as to whether it is the right or wrong answer. In the end, a final score should be reported for the user. (See below for how to generate random numbers).
Sample Output This is a sample transcript of what your program should do. Values in the transcript in BOLD show the user inputs. So in this transcript the user inputs 33, Jeremy, 24, -16, and 80 the rest is output from the program.
Enter a random number seed: 33 Enter your name: Jeremy Hello Jeremy! Please answer the following questions: 4 + 20 = 24 Correct! 4 - 20 = -16 Correct! 4 * 20 = 80 Correct! You got 3 correct answers! That's 100.0%!
Your code will behave differently based on the random number seed you use and the answers provided by the user. Here is a second possible execution of this code. As before, values in BOLD are provided by the user - in this case 54, Bob, 8, 8, and 8 - the rest is the output of the program when you run it.
Enter a random number seed: 54 Enter your name: Bob Hello Bob! Please answer the following questions: 20 + 12 = 8 Wrong! The correct answer is: 32 20 - 12 = 8 Correct! 20 * 12 = 8 Wrong! The correct answer is: 240 You got 1 correct answers! That's 33.33333333333333%!
Random numbers: Random numbers in a computer are rarely random. Instead, computers use something known as a pseudorandom number generator. This is a process that gives the appearance of generating a random number sequence, but in fact, is entirely predictable if you know the value used to seed (i.e. start) the process. The upshot of this is that we can get predictable behavior out of a random number generator for testing by using a known seed that we use as input as here in this program. Then when we move it to actual use, we can use a different seed to give more random behavior - typically by using the system clock or other input that will be different from one execution to the next as our seed value instead of prompting for it.
To code this, we don't want to write our own pseudorandom generator. Not only would that be a fairly complicated thing to do with the Java knowledge we have currently, there are already pseudorandom generators built into the Java library for our use. For this project, you will need to use the generator implemented in the Java Random class. You will need to create a new Random object and then draw numbers from it using the nextInt method of the Random class.
The Random object's instance method nextInt will provide a random number between 0 and an upper bound (not including the upper bound) for example, the code below will provide an integer value for the variable x between 0 and 9 and a value for y between 1 and 10:
int seed = 1024; // For your project, use a value entered by the user as your seed. Random rnd = new Random(seed); int x = rnd.nextInt(10); // x will be an integer between 0 and 9 int y = rnd.nextInt(10) + 1; // y will be an integer between 1 and 10
How would you modify the code above so that Random uses the seed provided by the user and x will be assigned a value between 1 and 20? That's what you'll need to do for this project.
Note that you only ever want to create a single Random object in your code, even if you are generating multiple random numbers! Just make multiple calls to nextInt to get different random numbers, as the code snippet above does.
Displaying percentages: To display the percentage of answers correct you need to convert your integer count of the number of correct answers to a double percentage value. The following piece of Java code will display the decimal value of the fraction 1/4. Think about how you would modify this code to get the percentage of correct answers as required by this assignment:
int numerator = 1; double denominator = 4.0; double quotient = numerator/denominator; System.out.println(quotient); // will display a value of 0.25
------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
MUST USE THE CODE BELOW AND CONTINUE FROM THERE
/** * YOUR DESCRIPTION HERE! **/ import java.util.Random; import java.util.Scanner;
public class FunWithBranching {
public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.print("Enter a random number seed: "); // TODO: Your code here } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
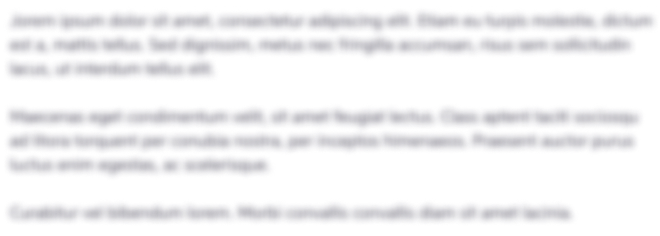
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started