Question
13.1: Multiplication Tables One of the principle applications of nested loops is to display tabular data (data organized into rows and columns). For this assignment,
13.1: Multiplication Tables
- One of the principle applications of nested loops is to display tabular data (data organized into rows and columns).
- For this assignment, let's practice the nested loop concept we discussed last class by creating a program to display the times tables.
- Name your Java project Multiplication
- First, the user should see a message like this one appear on the console:
***Time to Learn the Times Table!***
Enter the size of the times table: _
- Next, use a nested for loop to display the times table for the number entered from 1 to 12 (see example below)
- To help you get started, you can fill in the missing parts of the code here:
for (int row = ????; row <= 12; row++) { //printing times table 1 through 12 for (int col = ????; col <= ????; col++) { //for the numbers up to size System.out.print( col + " * " + row + "\t"); //add more to this print statement }
System.out.println();
}
- Now, as an intermediate step, run your code and verify that you get the following output:
***Time to Learn The Times Table!*** Enter the size of the times table: 5 1 * 1 2 * 1 3 * 1 4 * 1 5 * 1 1 * 2 2 * 2 3 * 2 4 * 2 5 * 2 1 * 3 2 * 3 3 * 3 4 * 3 5 * 3 1 * 4 2 * 4 3 * 4 4 * 4 5 * 4 1 * 5 2 * 5 3 * 5 4 * 5 5 * 5 1 * 6 2 * 6 3 * 6 4 * 6 5 * 6 1 * 7 2 * 7 3 * 7 4 * 7 5 * 7 1 * 8 2 * 8 3 * 8 4 * 8 5 * 8 1 * 9 2 * 9 3 * 9 4 * 9 5 * 9 1 * 10 2 * 10 3 * 10 4 * 10 5 * 10 1 * 11 2 * 11 3 * 11 4 * 11 5 * 11 1 * 12 2 * 12 3 * 12 4 * 12 5 * 12
- Finally, let's alter the print statement inside the for loop so that we print out the products, getting an output like the following:
***Time to Learn The Times Table!*** Enter the size of the times table: 5 1 * 1 = 1 2 * 1 = 2 3 * 1 = 3 4 * 1 = 4 5 * 1 = 5 1 * 2 = 2 2 * 2 = 4 3 * 2 = 6 4 * 2 = 8 5 * 2 = 10 1 * 3 = 3 2 * 3 = 6 3 * 3 = 9 4 * 3 = 12 5 * 3 = 15 1 * 4 = 4 2 * 4 = 8 3 * 4 = 12 4 * 4 = 16 5 * 4 = 20 1 * 5 = 5 2 * 5 = 10 3 * 5 = 15 4 * 5 = 20 5 * 5 = 25 1 * 6 = 6 2 * 6 = 12 3 * 6 = 18 4 * 6 = 24 5 * 6 = 30 1 * 7 = 7 2 * 7 = 14 3 * 7 = 21 4 * 7 = 28 5 * 7 = 35 1 * 8 = 8 2 * 8 = 16 3 * 8 = 24 4 * 8 = 32 5 * 8 = 40 1 * 9 = 9 2 * 9 = 18 3 * 9 = 27 4 * 9 = 36 5 * 9 = 45 1 * 10 = 10 2 * 10 = 20 3 * 10 = 30 4 * 10 = 40 5 * 10 = 50 1 * 11 = 11 2 * 11 = 22 3 * 11 = 33 4 * 11 = 44 5 * 11 = 55 1 * 12 = 12 2 * 12 = 24 3 * 12 = 36 4 * 12 = 48 5 * 12 = 60
- Note: Part of the output may get cut off if the user enters too large of a number or the numbers might not line up perfectly. Both are okay.
- When you are finished, submit your assignment to Canvas.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
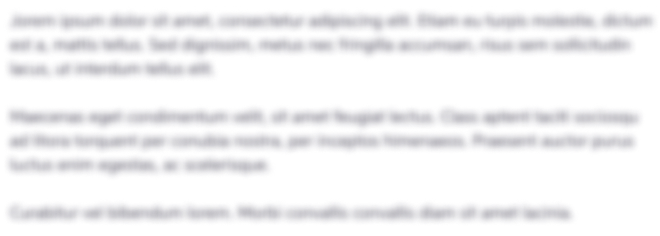
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started