Question
2. (50 marks) A string in C++ is simply an array of characters with the null character(0) used to mark the end of the string.
2. (50 marks) A string in C++ is simply an array of characters with the null character(\0)
used to mark the end of the string. C++ provides a set of string handling function in
Template Library), C++ now provides a string class.
But for this assignment, you are to develop your own string class. This will give you a
chance to develop and work with a C++ class, define constructors and destructors, define
member functions outside of the class body, develop a copy constructor and assignment
operator (and understand why!), work with C-Style strings and pointers, dynamically
allocate memory and free it when done.
Of course, you must also do suitable testing which implies writing a main function that
uses your new string class. Submit your assignment under URCourses.The following is the skeleton of the Mystring class declaration. Mystring.h file is
provided. You must produce the Mystring.cpp and main.cpp files.
class Mystring
{
private:
char *pData;
//pointer to simple C-style representation of the string
//(i.e., sequence of characters terminated by null)
//pData is only a pointer. You must allocate space for
//the actual character data
int length;
//length of the string
//
//possibly other private data
public:
MyString();
//constructor --- create empty string
MyString(char *cString); //constructor --- create a string whose data is a copy of
//cString
~MyString();
//destructor -- don't forget to free space allocated by the constructor
//i.e., the space allocated for the character data
MyString(MyString const& s); //override the default copy constructor --- why?
//important -- think about it -- possible test question
MyString operator = (MyString const& s); //override default assignment operator
void Put();
//output string
void Reverse();
//reverse the string
MyString operator + (MyString const& s); //concatenation operator
//
//other useful member functions
//as you wish
};
In addition, prepare a graphical explanation of each of your member functions. (Pseudo
code or flowchart or some diagram to show your design.)#ifndef MYSTRING_H
#define MYSTRING_H
class MyString
{
private:
char *pData;
//pointer to simple C-style representation of the string
//(i.e., sequence of characters terminated by null)
//pData is only a pointer. You must allocate space for
//the actual character data
int length;
//length of the string
//
//possible other private data
public:
MyString();
//constructor --- create empty string
MyString(char *cString); //constructor --- create a string whose data is a copy of
//cString
~MyString();
//destructor -- don't forget to free space allocated by the constructor
//i.e., the space allocated for the character data
MyString(MyString const& s); //override the default copy constructor --- why?
//important -- think about it -- possible test question
MyString operator = (MyString const& s); //override default assignment operator
void Put();
//output string
void Reverse();
//reverse the string
MyString operator + (MyString const& s); //concatenation operator
//
//other useful member functions
//as you wish
};
#endif // MYSTRING_H
Step by Step Solution
There are 3 Steps involved in it
Step: 1
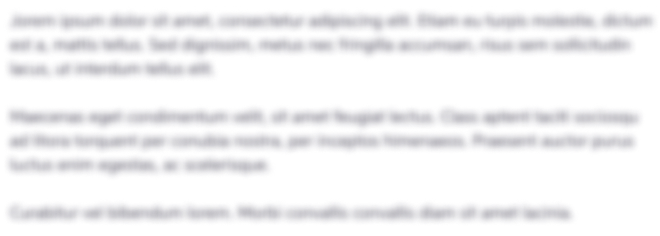
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started