Question
2. Consider the classes shown below: public class Parent { public void doSomething(){/* Implementation not shown */} } public class Child extends Parent { public
2. Consider the classes shown below:
public class Parent
{
public void doSomething(){/* Implementation not shown */}
}
public class Child extends Parent
{
public void doAnotherThing(){/* Implementation not shown */}
}
Which lines in the following code will compile without error?
Child kid = new Child();
kid.doSomething(); // line 1
kid.doAnotherThing(); // line 2
a) Line 1 only
b) Line 2 only
c) Lines 1 and 2
d) Neither line will compile without error
3. Consider the classes shown below:
public class Parent
{
private int value = 100;
public int getValue()
{
return value;
}
}
public class Child extends Parent
{
private int value;
public Child(int number)
{
value = number;
}
}
What is the output of the following lines of code?
Child kid = new Child(-14);
Parent adult = new Parent();
System.out.println(kid.getValue() + " "
+ adult.getValue());
a) 100 100
b) -14 100
c) -14 -14
d) 100 -14
4. Suppose the class Value is partially defined below
public class Value
{
private int number;
public int getValue()
{
return number;
}
}
A subclass of Value, LargerValue, is defined with a getValue method that returns twice the value of the parent. Which line is the body of LargerValue's getValue method?
a) return getValue() * 2;
b) return super.getValue() * 2;
c) return number * 2;
d) return super.number * 2;
5. You are creating a Motorcycle class which is supposed to inherit from the Vehicle class. Which of the following class declaration statements will accomplish this?
a) public class Motorcycle inherits Vehicle
b) public class Motorcycle implements Vehicle
c) public class Motorcycle interfaces Vehicle
d) public class Motorcycle extends Vehicle
6. Consider the following class hierarchy:
public class Vehicle
{
private String type;
public Vehicle(String type)
{
this.type = type;
}
public String displayInfo()
{
return type;
}
}
public class LandVehicle extends Vehicle
{
public LandVehicle(String type)
{
super(type);
}
}
public class Auto extends LandVehicle
{
public Auto(String type)
{
super(type);
}
}
You have written a program to use these classes, as shown in the following code snippet:
public class VehicleTester
{
public static void main(String[] args)
{
Auto myAuto = new Auto("sedan");
System.out.println("MyAuto type = " + ______);
}
}
Complete the code in this program snippet to correctly display the auto's type.
a) myAuto.displayInfo()
b) myAuto.super.displayInfo()
c) myAuto.super.super.displayInfo()
d) This cannot be done unless the Auto class overrides the displayInfo method.
7. Consider the following class hierarchy:
public class Vehicle
{
private String type;
public Vehicle(String type)
{
this.type = type;
}
public String displayInfo()
{
return type;
}
}
public class LandVehicle extends Vehicle
{
public LandVehicle(String type)
{
. . .
}
}
public class Auto extends LandVehicle
{
public Auto(String type)
{
. . .
}
public String displayAutoType()
{
return _____;
}
}
Complete the code in the Auto class method named displayAutoType to return the type data.
a) super(type);
b) super.type;
c) super.super.type;
d) super.displayInfo()
8. Consider the following code snippet:
Employee programmer = new Employee(10254, "exempt");
String s = programmer.toString();
Assume that the Employee class has not implemented its own toString() method. What value will s contain when this code is executed?
a) s will contain the values of the instance variables in programmer.
b) s will contain only the class name of the programmer object.
c) s will contain the class name of the programmer object followed by a hash code.
d) This code will not compile.
9. Consider the following code snippet:
class MyListener implements ActionListener
{
public void actionPerformed(ActionEvent event)
{
System.out.println(event);
}
}
Timer t = new Timer(interval, listener);
t.start();
What is wrong with this code?
a) The Timer object should be declared before the MyListener class.
b) The listener has not been attached to the Timer object.
c) The Timer object must be declared as final.
d) There is nothing wrong with the code.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
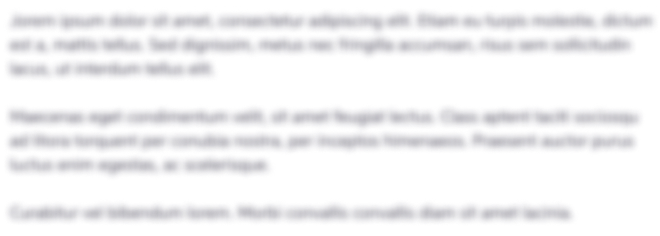
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started