Question
2. Consider the problem of replacing all straight quotes like this with curly quotes like this in a string. Before embarking on this task, lets
2. Consider the problem of replacing all straight quotes "like this" with curly quotes like this in a string. Before embarking on this task, lets think of a reusable method. Suppose we know where the straight quote is. Then we want to replace it. That would be useful in other situations too.
Your task is to complete the function replace_at that replaces the character at a given position with an arbitrary string.
#include using namespace std; /** Replaces a character of a string at a given position. @param str the string where the replacement takes place @param position the position of the character to be replaced @param replacement the replacement string @return str with the character at the position changed to the replacement string, or the original string if position was not valid. */ string replace_at(string str, int position, string replacement) { . . . }
3. Consider again the problem of replacing all straight quotes "like this" with curly quotes like this in a string. We need to know the position of the first quotation mark, then the second quotation mark. We could write functions for these tasks, but we can do better. In general, we may want to look for a character and find the nth occurrence.
Your task is to complete the function find_occurrence that returns the nth occurrence of a character within a string.
#include using namespace std; /** Finds the nth occurrence of a given character in a string. @param str the string @param ch the character to search @param n the occurrence count @return the position of the nth occurrence of ch in str, or -1 if ch doesn't occur n times. */ int find_occurrence(string str, string ch, int n) { . . . return -1; }
4. Now we are ready to tackle the problem of replacing all pairs of straight quotes "like this" with curly quotes like this in a string. Using the helper functions, replace_at and find_occurrence, from the two preceding exercises, implement the replace_quotesfunction below.
For example, to find the first quotation mark, call
int first = find_occurrence(result, "\"", 1);
To replace it with an opening curly quote, call
result = replace_at(result, first, left_quote);
If there is an odd number of straight quotes, don't replace the last one.
Note that the left and right curly quotes each use two bytes of memory, so the length of the string changes when you replace a straight quote. This will be explained more fully in Chapter 8.
#include using namespace std; int find_occurrence(string str, string ch, int n); string replace_at(string str, int position, string replacement); /** Replaces all pairs of straight quotes with curly quotes. @param str the string to process @return str with adjacent pairs of straight quotes changed to curly quotes */ string smart_quotes(string str) { string result = str; string left_quote = ""; string right_quote = ""; . . . return result; } /** Finds the nth occurrence of a given character in a string. @param str the string @param ch the character to search @param n the occurrence count @return the position of the nth occurrence of ch in str, or -1 if ch doesn't occur n times. */ int find_occurrence(string str, string ch, int n) { int count = 0; for (int i = 0; i < str.length(); i++) { if (str.substr(i, 1) == ch) { count++; if (count == n) { return i; } } } return -1; } /** Replaces a character of a string at a given position. @param str the string where the replacement takes place @param position the position of the character to be replaced @param replacement the replacement string @return str with the character at the position changed to the replacement string, or the original string if position was not valid. */ string replace_at(string str, int position, string replacement) { if (0 <= position && position < str.length()) { return str.substr(0, position) + replacement + str.substr(position + 1); } else { return str; } }
5.
Wiki markup is a simple convention indicating which parts of text should be bold, italic, computer code, and so on. The symbols used for each feature vary. We will use these simple rules:
Bold is indicated as *Bold*
Italic is indicated as !Italic!
Code is indicated as `Code`
Superscript is indicated with ^, such as 10^23^ for 1023
Subscript is indicated with _, such as H_2_O for H2O
To include one of these symbols in the text, precede it by a backslash\, such as \!Hello\!.
In order to see the text, we would like to translate the symbols into HTML tags. For example, the `main` function should turn into the main
function.
Rearrange the following lines of pseudocode that decomposes this problem into simpler tasks (finding, replacing, and removing characters).
Assume that find position of ... at or after ... returns -1 if there is no match.
Replace the symbol at the end first. (If you replace the start tag, the end position shifts.)
Press start to begin.
Start
while not done
else
start = find position of *!`^_ at or after start
symbol = character at position start
if start == -1
end = find position of symbol at or after start + 1
tag = tag for symbol
done = true
done = false
Replace character at start with "<" + tag + ">"
if end -1
Replace character at end with "">"
Remove all backslashes
start = 0
In the preceding exercise, you started the process of stepwise refinement for the task of translating Wiki markup to HTML. Let us continue the process.
One of the steps was to find position of next *!`^_ at or after start. We want to turn this into a function call next_symbol(message, symbols, start) where symbols is a string of the symbols that we are looking for (such as "*!`^_"). We want to return the position of the first of these symbols in the message at position start.
Rearrange the following lines to produce pseudocode for this function.
Press start to begin.
Start
return -1
while pos < length of message
else if symbols contains ch
return pos
pos = pos + 2
if ch is a backslash
pos++
else
ch = message.substr(pos, 1)
pos = start
In the preceding exercises, you saw how one can use stepwise refinement to solve the problem of translating Wiki markup to HTML. Now turn the pseudocode into code. Complete the convert_to_HTML and next_symbol functions.
#include#include using namespace std; // Functions defined below string convert_to_HTML(string message); int next_symbol(string message, string symbols, int start); string replace_at(string str, int position, string replacement); string tag_for_symbol(string symbol); string replace_escapes(string str); /** Converts a message with Wiki markup to HTML. @param message the message with markup @return the message with Wiki notation converted to HTML */ string convert_to_HTML(string message) { string result = message; . . . return replace_escapes(result); } /** Finds the next unescaped symbol. @param message a message with Wiki markup @param symbols the symbols to search for @param start the starting position for the search @return the position of the next markup symbol at or after start */ int next_symbol(string message, string symbols, int start) { . . . } int main() { string message; getline(cin, message); cout << convert_to_HTML(message) << endl; return 0; } /** Replaces a character of a string at a given position. @param str the string where the replacement takes place @param position the position of the character to be replaced @param replacement the replacement string, or the original string if position was not valid. */ string replace_at(string str, int position, string replacement) { if (0 <= position && position < str.length()) { return str.substr(0, position) + replacement + str.substr(position + 1); } else { return str; } } /** Gets the HTML tag for a markup symbol. @param symbol the markup symbol @return the corresponding HTML tag, or "" if none found */ string tag_for_symbol(string symbol) { if (symbol == "!") { return "em"; } else if (symbol == "*") { return "strong"; } else if (symbol == "`") { return "code"; } else if (symbol == "^") { return "super"; } else if (symbol == "_") { return "sub"; } else { return ""; } } /** Removes escape sequences from a string. @param str the string to process. @return the string with all \\ characters removed. */ string replace_escapes(string str) { string result; for (int i = 0; i < str.length(); i++) { string ch = str.substr(i, 1); if (ch != "\\") { result = result + ch; } } return result; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
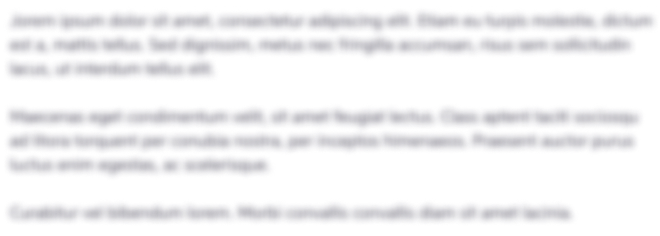
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started