Question
2. Create a command shell based on commandServer2.c that recognizes the following commands and calls appropriately named stub routines for each of them. Hand in
2. Create a command shell based on commandServer2.c that recognizes the following commands and calls appropriately named stub routines for each of them. Hand in a separate listing and set of tests that shows that the appropriate routine is called . Create the stubs in a separate file that is included with your main and interpret routine. DO NOT IMPLEMENT THE FUNCTIONALITY OF ANY OF THESE METHODS AT THIS STAGE. The only thing a stub routine should do is return a string This functionName is not yet implemented in order to identify which function was called. (4) Use a lookup table to find each command. If the command isnt in the list respond with commandname not found). a. export var=value unset var b. chdir dirname (3) c. access file1 file2 file3 (2) d. chmod octalPermission file1 file2 file3 . (3). e. path file1 file2 file3 . (3) f. touch -m time1 -a time2 file1 file2 file3 (3). g. ln -s -f file1 file2 (3) ==================================== Here is the commandServer2.c for the above question:
#include#include #include #include #include #include #include #include #include #include #include #include void ctrlCHandler(int signum) { fprintf(stderr,"Command server terminated using C "); exit(1); } //Examples of stub routines - the purpose here is to test commmand and control char * f1() { return "Command 'one' was received"; } char * f2(char *cmd) { return "Command 'two' was received"; } char * f3() { return "Command 'three' was received"; } char * f4() { return "Command four was received"; } //2 parallel arrays. commands is a list of commands to recognize // methods is an array of function pointers to call. char *commands[10]={"one","two","three","four", "alias"} ; char *(*methods[10])()={f1,f2,f3,f4,f2}; //Alternate declaration struct CMDSTRUCT { char *cmd; char *(*method)(); } cmdStruct[]={{"fred",f1},{"mary",f2},{"clark",f3},{"sonia",f4},{NULL,NULL}} ; char *interpret(char *cmdline) { char **tokens; char *cmd; int i; char *result; tokens=history_tokenize(cmdline); //Split cmdline into individual words. if(!tokens) return "no response needed"; cmd=tokens[0]; //Detecting commands: table lookup: 2 techniques //Using the parallel arrays to look up function calls for(i=0;commands[i];i++) { if(strcasecmp(cmd,commands[i])==0) return (methods[i])(cmd,&tokens[1]); } //Using struct CMDSTRUCT as an alternative lookup method. Pick either technique, not both //Note that its possible to create multiple aliases for the same command using either method. for(i=0;cmdStruct[i].cmd;i++) if(strcasecmp(cmd,cmdStruct[i].cmd)==0) return (cmdStruct[i].method)(cmd,&tokens[1]); return "command not found"; } int main(int argc, char * argv[],char * envp[]) { char cmd[100]; char *cmdLine; char *expansion; time_t now=time(NULL); int nBytes; //size of msg rec'd signal(SIGINT,ctrlCHandler); read_history("shell.log"); add_history(ctime(&now)); fprintf(stdout,"Starting the shell at: %s ",ctime(&now)); while(true) { cmdLine=readline("Enter a command: "); if(!cmdLine) break; history_expand(cmdLine,&expansion); add_history(expansion); if(strcasecmp(cmdLine,"bye")==0) break; char *response=interpret(cmdLine); fprintf(stdout,"%s ",response); } write_history("shell.log"); system("echo Your session history is; cat -n shell.log"); fprintf(stdout,"Server is now terminated "); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
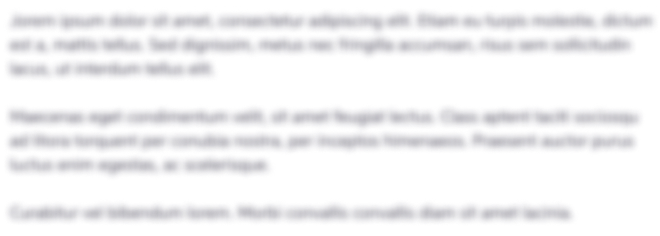
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started