Question
2. Define a class named Student in your listDriver package. Every Student has a name, which is a String, and every Student has an id
2. Define a class named Student in your listDriver package. Every Student has a name, which is a String, and
every Student has an id number, which is an int. Two Students are considered equal if they have the same id
number, even if they have different names. Also include methods getName() and getID() in that class. In the
attached driver, LabDeleteListIterator, there is a method which needs to be completed. Its name
is deleteFirstOfDup(). It is supposed to search a List named roster for the first pair of neighboring Students which
are equal; then it should delete the first Student of that pair from the List.
You may give the main method a parameter, but do not make other changes to the driver.
Hint: Use a ListIterator. List Interface
public interface List
/** * Clear this List * */ void clear();
/** * @return true iff this List is empty * */ boolean isEmpty(); /** * @return the position of the first occurrence of the given Object in this List, * or -1 if it is not in this List */ int indexOf(Object obj); /** * @return true only if the given Object is in this List */ boolean contains (Object obj);
/** * @return this List as a String */ public String toString(); /** * Remove the first occurrence of the given object from this List. * @return true iff it was removed */ boolean remove (Object obj); /** * @return an Iterator for this List */ Iterator
package list;
public interface Iterator
LabDeleteListIterator Class:
package listDriver; import list.*;
/** * Lab Problem. Method to search a list for the first pair of equal neighbors. * Delete the first member of that pair. * */ public class LabDeleteListIterator { static List roster; public static void main() { roster = new ArrayList(); test(); roster = new LinkedList(); test(); } private static void init() { deleteFirstOfDup(); roster.add (new Student ("jim", 2222)); deleteFirstOfDup(); roster.add (new Student ("joseph", 2345)); roster.add (new Student ("joe", 2345)); roster.add (new Student ("mary", 3333)); roster.add (new Student ("maryLou", 3333)); System.out.println("Before deletions " + roster); } private static void test() { init(); deleteFirstOfDup(); if (roster.size() != 4) System.err.println ("Deletion is incorrect"); System.out.println ("Roster is " + roster); } /** Search the roster for the first pair of neighbors which are * equal. Delete the first member of that pair. */ private static void deleteFirstOfDup() { /////////// PUT YOUR CODE HERE /////////////// } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
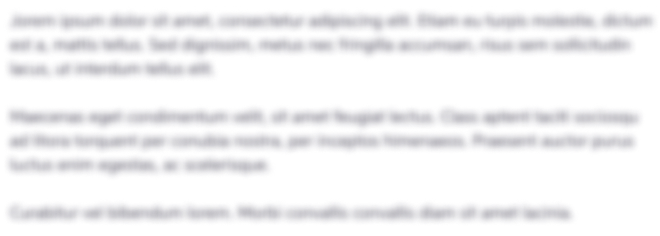
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started