Question
2. Download and open the Nave Ticket Machine project Ive posted -- adapted from the project in Chapter 2 of the BlueJ textbook used in
2. Download and open the Nave Ticket Machine project Ive posted -- adapted from the project in Chapter 2 of the BlueJ textbook used in IOOP. a. In the main() method in class SingletonTesting, note added line of code to instantiate a second TicketMachine object with, say, a different price than that in the first, and demonstrate functionality. b. Revise class TicketMachine to make it a Singleton. In the line that creates the initial [single] instance, call the TicketMachine constructor using 500 as the cost of a ticket. c. Try to re-run your demo code that creates a new TicketMachine(...) [wont compile!] d. Comment out the lines in your demo to create a second TicketMachine, and revise the original line to work with the Singleton object and demonstrate functionality. [Hint: In your test driver, youll need to get the instance, not create a new Ticket Machine.]
Deliverables: Zip of TicketMachine project plus your WORD doc with screen shots and results
Heres the code for the 2 java files:
**** TicketMachine: ****
/**
* TicketMachine models a naive ticket machine that issues
* flat-fare tickets.
* The price of a ticket is specified via the constructor.
* It is a naive machine in the sense that it trusts its users
* to insert enough money before trying to print a ticket.
* It also assumes that users enter sensible amounts.
*
*
*/
public class TicketMachine
{
// The price of a ticket from this machine.
private int price;
// The amount of money entered by a customer so far.
private int balance;
// The total amount of money collected by this machine.
private int total;
/**
* Create a machine that issues tickets of the given price.
* Note that the price must be greater than zero, and there
* are no checks to ensure this.
*/
public TicketMachine(int cost)
{
price = cost;
balance = 0;
total = 0;
}
/**
* Return the price of a ticket.
*/
public int getPrice()
{
return price;
}
/**
* Return the amount of money already inserted for the
* next ticket.
*/
public int getBalance()
{
return balance;
}
/**
* Receive an amount of money from a customer.
*/
public void insertMoney(int amount)
{
balance = balance + amount;
}
/**
* Print a ticket.
* Update the total collected and
* reduce the balance to zero.
*/
public void printTicket()
{
// Simulate the printing of a ticket.
System.out.println("##################");
System.out.println("# The BlueJ Line");
System.out.println("# Ticket");
System.out.println("# " + price + " cents.");
System.out.println("##################");
System.out.println();
// Update the total collected with the balance.
total = total + balance;
// Clear the balance.
balance = 0;
}
}
**** SingletonTesting: ****
public class SingletonTesting
{
public static void main(String[] args)
{
TicketMachine tm1 = new TicketMachine(500);
TicketMachine tm2 = new TicketMachine(200);
System.out.println("Tm1 Price = " + tm1.getPrice());
System.out.println("Tm2 Price = " + tm2.getPrice());
}
}
2. Download and open the Nave Ticket Machine project I've posted -- adapted from the project in Chapter 2 of the BlueJ textbook used in IOOP. a. In the main() method in class SingletonTesting, note added line of code to instantiate a second TicketMachine object with, say, a different price than that in the first, and demonstrate functionality. b. Revise class TicketMachine to make it a Singleton. In the line that creates the initial [single] instance, call the TicketMachine constructor using 500 as the cost of a ticket. c. Try to re-run your demo code that creates a new TicketMachine(...) [won't compile!] d. Comment out the lines in your demo to create a second TicketMachine, and revise the original line to work with the Singleton object - and demonstrate functionality. [Hint: In your test driver, you'll need to get the instance, not create a new Ticket Machine.] Peliverables: Zip of TicketMachine project plus your WORD doc with screen shots and results
Step by Step Solution
There are 3 Steps involved in it
Step: 1
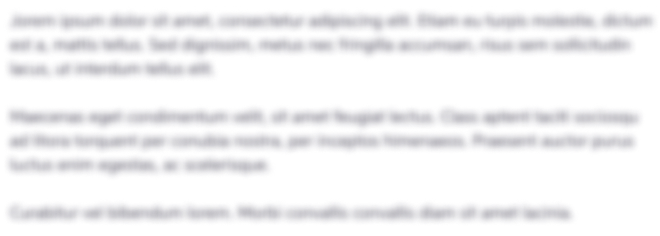
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started