Question
(+20) Write a python program that: Ask the user for a string and creates the following dictionary: The values are the letters in the string,
(+20) Write a python program that:
Ask the user for a string and creates the following dictionary: The values are the letters in the string, with the corresponding key being the place in the string. For example if the user entered the string CSC120 then create the dictionary d
d = {0:'C', 1:'S', 2:'C', 3:'1', 4:'2', 5:'0'}
Display the length of the dictionary d In this case it is 6
Ask the user for a key(integer). If the key is in the dictionary display the corresponding value. As an example if the user entered 2 display C if the user entered a key that was not in the dictionary display Error key not found
Ask the user for a value (one of the characters). If the value is in the dictionary display Value found otherwise display Value not found. As an example if the user entered C display Value found
Ask the user for a string of digits, separated by a comma. Print out the corresponding values for those digits.
For example if the user enters the string 0,1, 4 then print out C S 2 (or CS2 ) At issue is how to handle out of range digits for example, in our case, any digits in the range 05 should generate a corresponding value but any digit(s) > 5 OR < 0 has to handled appropriately -- I leave that to you to decide ( generate an error message is a suggestion )
Tutorial on sets and dictionary can be found at : (left side in the Table of Contents 5.4 and 5.5 https://docs.python.org/2/tutorial/datastructures.html
Tutorial on strings can be found at
Try your code on the string CSC 120ComputingFundamentals
See the Lab09Template.py in our Source code tab for hints on the lab Duplicated here
#lab09Template.py
names = { 10:"Euclid", 20:"Archimedes", 30:"Newton", 40:"Descartes", 50:"Fermat", 60:"Turing", 70:"Euler", 80:"Einstein", 90:"Boole", 100:"Fibonacci", 110:"Nash" } print("1 ",names.keys()) # print keys print("2 ", names.values() ) # insert names[120] = "Hopper" print("names == ", names) #use in to search for keys as well as non-existance keys key = 110 if key in names.keys(): print("4 ",names[key]) else: print("error key == 110") s = "Euler" if s in names.values(): print(" 3 YES") else: print("NO Euler") for key in names: print(key, names[key]) print("====VALUES====") for values in names.values(): print(values) print("====string manipulation====") s = (input("Enter integers seperated by a comma ")) # NOTE cannot convert 110,20,3 into an integer print('s ==' , s) s = s.split(',') # now split using , as a seperator, into individual words print('1',s, len(s) ) # still a string but separated into words x = int(s[0]) # now convert to a string print('x == ', x) # now print it print('value for the key == ',x, ' is ', names[x]) for i in s: print('i == ', i) print ("=================SET THEORY=============") a = set('ABCD') b = set('CDEFF') print(" a == ", a) # unique letters in a print(" b == ", b) # unique letters in b print(" a - b ",a - b) # letters in a but not in b print( "a | b ", a | b) # letters in a or b print( "a & b ", a & b) # letters in both a and b print( "a ^ b ", a ^ b) # letters in a or b but not both
names =={100: 'Fibonacci', 70: 'Euler', 40: 'Descartes', 10: 'Euclid', 110: 'Nash', 80: 'Einstein', 50: 'Fermat', 20: 'Archimedes', 120: 'Hopper', 90: 'Boole', 60: 'Turing', 30: 'Newton'}
3 YES
100 Fibonacci
70 Euler
40 Descartes
10 Euclid
110 Nash
80 Einstein
50 Fermat
20 Archimedes
120 Hopper
90 Boole
60 Turing
30 Newton
====string manipulation====
Enter integers separated by a comma 10,20,3
s == 10,20,3
1 ['10', '20', '3'] 3
x ==10
value for the key ==10isEuclid
i ==10
i ==20
i ==3
=================SET THEORY=============
a =={'D', 'A', 'C', 'B'}
b =={'D', 'F', 'C', 'E'}
a - b {'A', 'B'}
a | b{'F', 'A', 'B', 'D', 'C', 'E'}
a & b{'D', 'C'}
a ^ b{'F', 'A', 'B', 'E'}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
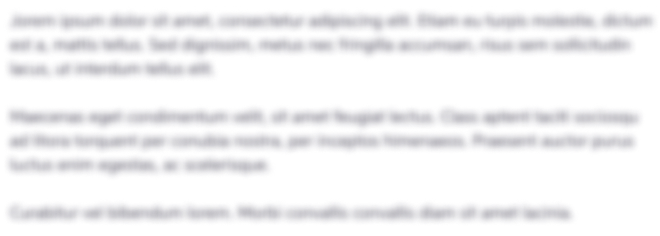
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started