Question
21. How many columns are in the array? (1 point) int[][] array1 = new int[15][10]; 0 2 10 15 22. Suppose you write a subclass
21. How many columns are in the array? (1 point)
int[][] array1 = new int[15][10];
0 2 10 15
22. Suppose you write a subclass of Insect named Ant. You add a new method named doSomething to the Ant class. You write a client class that instantiates an Ant object and invokes the doSomething method. Which of the following Ant declarations will NOT permit this? (2 points)
Insect a = new Ant(); Ant a = new Ant(); Ant a = new Insect();
I only II only III only I and II only I and III only
23. Consider the following code:
String[] fruits = {"apple", "pear", "mango", "peach"}; int i = 3; String str = "p";
for(String item : fruits) { i = item.indexOf("p") + 2; str += item.substring(i); }
System.out.println(str);
Assume that the loop accesses array elements in order. What is output by this code? (2 points)
learangoach peearmangoeach plearangoach ppplepearpeach A StringIndexOutOfBoundsException occurs.
24. Consider the following code:
int[] arr = new int[ < some positive integer > ]; int index;
// assume arr is filled with values here // assume the user enters a value for index here
if((index >= 0) && (index <= arr.length)) { System.out.println(arr[index]); }
When could this code throw an exception? (2 points)
When index is less than 0. When index is equal to 0. When index is greater than the arr.length. When index is equal to the arr.length. This code cannot throw an exception because the if statement prevents it.
25. Consider a method that compares the elements of two ArrayLists and returns the value true if the elements in corresponding positions are equal, and returns false otherwise.
Which of the following code will accomplish this? (2 points)
boolean temp = false; int i; for(i = 0; i < a.size(); i++) { if(a.get(i) == b.get(i)) { temp = true; } } return temp;
int i; for(i = 0; i < a.size(); i++) { if(a.get(i) != b.get(i)) { return false; } else { return true; } }
int i = 0; while(i < a.size() && a.get(i) == b.get(i)) { i++; } return (i == a.size());
boolean temp = true; int i; for(i = 0; i < a.size(); i++) { temp = (a.get(i) == b.get(i)); } return temp;
int i = 0; while(i < a.size() && a.get(i) == b.get(i)) { i++; } if(i > a.size()) { return true; } return false;
26. Assume table has been declared and initialized as a two-dimensional integer array with 9 rows and 5 columns. Which segment of code will correctly find the sum of the elements in the fifth column? (2 points)
int sum = 0; for(int i = 0; i < table.length; i++) sum += table[4][i];
int sum = 0; for(int i = 0; i < table.length; i++) sum += table[i][4];
int sum = 0; for(int i = 0; i < table[0].length; i++) sum += table[i][4];
int sum = 0; for(int outer = 0; outer < table[0].length; outer++) for(int inner = 0; inner < table.length; inner++) sum += table[outer][4];
int sum = 0; for(int outer = 0; outer < table.length; outer++) for(int inner = 0; inner < table[0].length; inner++) sum += table[outer][4];
27. Suppose a class named SportsCar is a subclass of a class called Automobile. Automobile has only two methods: accelerate and addGas. Which statement is true? (2 points)
A SportsCar object has the methods accelerate and addGas only. No other methods are allowed, since no other methods are inherited. A SportsCar object has at least the methods accelerate and addGas. It might add its own methods. A SportsCar object has the methods accelerate and addGas only if it is cast to Automobile. A SportsCar object has the methods accelerate and addGas only if they are static. A SportsCar object has the methods accelerate and addGas only if they are abstract.
28. Consider an integer array, vals, which has been declared with one or more integer values. Which of the code segments updates vals so that each element contains the sum of the original value with the current index? (2 points)
for (int v: vals) { v = v + v; }
for (int m = 1; m < = vals.length; m++ ) { vals[m] = vals[m] + m; }
int m = 0; while (m < vals.length) { vals[m] = vals[m] + m; m++; }
II only III only I and III II and III I, II, and III
29. Consider the following code block.
String str = "Good Evening. How are you?"; String newString = str.substring(0, str.indexOf("."));
Which of the following BEST describes the result of the code above? (2 points)
"How are you?" is assigned to newString "Good Evening" is assigned to newString "Good Evening." is assigned to newString "12" is assigned to newString There is a syntax error in the code
30. Consider the following code segment. What would print when the code executes? (2 points)
int num1 = 12; int num2 = 2; while (num1 < num2) { System.out.print(num1 % num2 + " "); num2++; }
0 0 0 2 0 5 0 3 2 1 0 0 0 2 0 5 4 3 2 0 0 2 0 5 4 3 2 1 0 0 0 2 0 5 4 3 2 1 The loop never executes
Step by Step Solution
There are 3 Steps involved in it
Step: 1
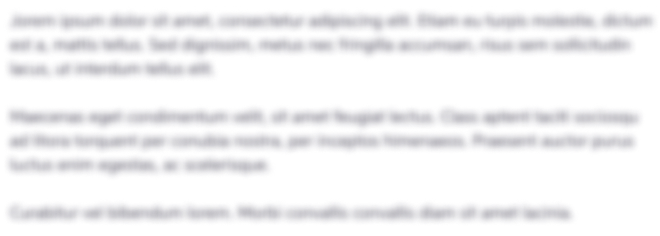
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started