Question
21. Is there any thing wrong with the following code snippet? String[] data = { abc, def, ghi, jkl }; String searchedValue = ghi; int
21.
Is there any thing wrong with the following code snippet?
String[] data = { "abc", "def", "ghi", "jkl" };
String searchedValue = "ghi";
int pos = 0;
boolean found = false;
while (pos < data.length)
{
if (data[pos].equals(searchedValue))
{
found = true;
}
else
{
found = false;
}
pos++;
}
if (found)
{
System.out.println("Found at position: " + pos);
}
else
{
System.out.println("Not found");
}
Select one:
a. There is nothing wrong.
b. There is compile-time error.
c. There is a bounds error.
d. There is a logic error
22.
Characters that are grouped together between double quotes (quotation marks) in
Java are called
Select one:
a. reserved words
b. syntax
c. symbols
d. strings
23.
Assuming that a user enters 22 as the price of an object, which of the following
hand-trace tables is valid for the given code snippet?
int price = 0;
String status = "";
Scanner in = new Scanner(System.in);
System.out.print("Please enter objects price: ");
price = in.nextInt();
if (price >= 50)
{
status = "reasonable";
if (price >= 75)
{
status = "costly";
}
}
else
{
status = "inexpensive";
if (price <= 25)
{
status = "reasonable";
}
}
Select one:
a.
price
status
0
""
22
"inexpensive"
"reasonable"
b.
price
status
0
"inexpensive"
22
"reasonable"
c.
price
status
0
22
"reasonable"
"costly"
d.
price
status
0
"reasonable"
22
"costly"
24.
Consider the following code snippet. Assuming that the user inputs 75 as the age,
what is the output?
int age = 0;
Scanner in = new Scanner(System.in);
System.out.print("Please enter your age: ");
age = in.nextInt();
if (age < 10)
{
System.out.print("Child ");
}
if (age < 30)
{
System.out.print("Young adult ");
}
if (age < 70)
{
System.out.print("Old ");
}
if (age < 100)
{
System.out.print("Impressively old ");
}
a. Impressively old
b. Child Young adult Old
c. Young adult Old
d. Child Young adult Old Impressively old
25.
Given the following method, what do we need to fix?
public static String getPerformance(char grade)
{
switch (grade)
{
case 'A': return "Excellent"; break;
case 'B': return "Good"; break;
case 'C': return "Mediocre"; break;
case 'D': return "Weak"; break;
case 'F': return "Bad"; break;
}
}
Select one:
a. Remove all the break statements
b. Add a local boolean variable definition
c. Remove all the break statements and add a return statement before the
end of method
d. Remove the switch statement and use and if statement
Step by Step Solution
There are 3 Steps involved in it
Step: 1
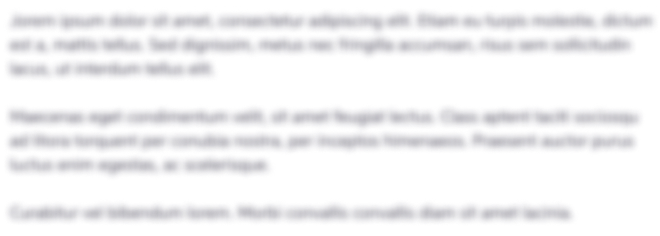
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started