Question
2.4 Text Generator Write a client program TextWriter that takes two command-line integers k and L, reads the input text from standard input, and builds
2.4 Text Generator Write a client program TextWriter that takes two command-line integers k and L, reads the input text from standard input, and builds a Markov model of order k from the input text. Then, starting with the k -gram consisting of the rst k characters of the input text, print out L characters generated by simulating a trajectory through the corresponding Markov chain. You may assume that the text has length at least k and that L k. ./TextWriter 2 11 < input17.txt 3 Debugging and Testing You should write a test.cpp le that uses the Boost functions BOOST_REQUIRE_THROW and BOOST_REQUIRE_NO_THROW to verify that your code properly throws the specied exceptions when appropriate (and does not throw an exception when it shouldn't). As usual, use BOOST_REQUIRE to exercise all methods of the class. Make sure you throw std::runtime_errors in the methods per the spec. Use Boost unit testing to test your implementation by using the next sequence "gagggagaggcgagaaa" Your test le must exercise all methods of your RandWriter class I will provide my codes so far. RandWriter.cpp // Copyright Jiho Lee 2023
#include
#include
#include
#include "RandWriter.hpp"
RandWriter::RandWriter(std::string txt, size_t k) : text(txt), k_order(k) {
if (text.length() < k_order) {
throw std::invalid_argument("Text length should be at least the order k.");
}
buildModel();
}
size_t RandWriter::orderK() const {
return k_order;
}
size_t RandWriter::freq(std::string kgram) const {
if (kgram.length() != k_order) {
throw std::invalid_argument("kgram should be of length k.");
}
return kgram_freq.count(kgram) ? kgram_freq.at(kgram) : 0;
}
size_t RandWriter::freq(std::string kgram, char c) const {
if (kgram.length() != k_order) {
throw std::invalid_argument("kgram should be of length k.");
}
if (kgram_next_char.count(kgram) && kgram_next_char.at(kgram).count(c)) {
return kgram_next_char.at(kgram).at(c);
}
return 0;
}
char RandWriter::kRand(std::string kgram) {
if (kgram.length() != k_order) {
throw std::invalid_argument("kgram should be of length k.");
}
std::vector
for (char c = ' '; c <= '~'; ++c) {
size_t frequency = freq(kgram, c);
for (size_t i = 0; i < frequency; ++i) {
possible_chars.push_back(c);
}
}
if (possible_chars.empty()) {
throw std::runtime_error("No such kgram found.");
}
std::mt19937 gen(std::chrono::system_clock::now().time_since_epoch().count());
std::uniform_int_distribution<> dis(0, static_cast
return possible_chars[dis(gen)];
}
std::string RandWriter::generate(std::string kgram, int L) {
if (kgram.length() != k_order) {
throw std::invalid_argument("kgram should be of length k.");
}
std::string result = kgram;
for (int i = k_order; i < L; ++i) {
char nextChar = kRand(kgram);
result += nextChar;
result = result.substr(result.length() - k_order);
kgram = result.substr(result.length() - k_order);
}
return result;
}
void RandWriter::buildModel() {
for (size_t i = 0; i <= text.length() - k_order; ++i) {
std::string kgram = text.substr(i, k_order);
char nextChar = text[i + k_order];
// Update kgram_freq
kgram_freq[kgram]++;
// Update kgram_next_char
kgram_next_char[kgram][nextChar]++;
}
}
std::ostream& operator<<(std::ostream& os, const RandWriter& rw) {
os << "Order: " << rw.k_order << std::endl;
// Print out k-gram and k+1-gram frequencies
for (const auto& entry : rw.kgram_freq) {
os << "k-gram: " << entry.first << ", Frequency: " << entry.second << std::endl;
}
for (const auto& entry : rw.kgram_next_char) {
for (const auto& innerEntry : entry.second) {
os << "k+1-gram: " << entry.first << innerEntry.first << ", Frequency: " << innerEntry.second << std::endl;
}
}
return os;
} RandWriter.hpp // Copyright Jiho Lee 2023
#pragma once
#include
#include
#include
class RandWriter {
public:
RandWriter(std::string text, size_t k);
size_t orderK() const;
size_t freq(std::string kgram) const;
size_t freq(std::string kgram, char c) const;
char kRand(std::string kgram);
std::string generate(std::string kgram, int L);
friend std::ostream& operator<<(std::ostream& os, const RandWriter& rw);
private:
std::string text;
size_t k_order;
std::map
std::map
void buildModel();
};
TextWriter.cpp // Copyright Jiho Lee 2023
#include
#include
#include
#include "RandWriter.hpp"
int main(int argc, char* argv[]) {
if (argc != 3) {
std::cerr << "Usage: " << argv[0] << " k L < input.txt" << std::endl;
return 1;
}
int k = std::stoi(argv[1]);
int L = std::stoi(argv[2]);
std::stringstream buffer;
buffer << std::cin.rdbuf();
std::string text = buffer.str();
try {
RandWriter writer(text, k);
std::string generatedText = writer.generate(text.substr(0, k), L);
std::cout << generatedText << std::endl;
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
return 1;
}
return 0;
}
I am generating new texts by using markov chain with c++. when I submit my code to autograder, I pass half of the tests, but fail other halfs. please implement my full codes to pass all the tests and provide full codes back. 1.0) Your Tests (0/4)Test Failed: 0 not greater than or equal to 4 : Usage: ./test k L < input.txt
- 1.1) TestOrderK (1/1)
- 1.2) TestOrderKFail (1/1)
- 2.1) TestFreq1 (1/1)
- 2.2) TestFreq3 (0/1)
- 2.3) TestFreqSpec1 (0/1)
- 2.4) TestFreqSpec3 (1/1)
- 2.5) TestFreqFail (1/1)
- 2.6) TestFreqSpecFail (1/1)
- 3.1) TestKRand1 (2/2)
- 3.2) TestKRand3 (2/2)
- 3.3) TestKRandText1 (1/1)
- 3.4) TestKRandText3 (1/1)
- 3.5) TestKRand1Freq (1/1)
- 3.6) TestKRand3Freq (0/1)
- 4.1) TestGenerate1Len (0/1)
- 4.2) TestGenerate3Len (0/1)
- 4.3) TestGenerate1Composition (0/1)
- 4.4) TestGenerate3Composition (0/1)
- 5.1) TextWriter 1 100 (0/1)Test Failed: 2 != 100 : expected len: 100, actual len: 2 h
- 5.2) TextWriter 3 300 (0/1)Test Failed: 4 != 300 : expected len: 300,
Running 1 test case... unknown location(0): fatal error: in "TestGenerate3Composition": std::runtime_error: No such kgram found. TestGenerate3Composition.cpp(18): last checkpoint: "TestGenerate3Composition" test entry *** 1 failure is detected in the t
Running 1 test case... TestGenerate1Composition.cpp(25): fatal error: in "TestGenerate1Composition": critical check s.size() == len has failed [1 != 10] *** 1 failure is detected in the test module "TextWriter Tests"
Running 1 test case... unknown location(0): fatal error: in "TestGenerate3Len": std::runtime_error: No such kgram found. TestGenerate3Len.cpp(18): last checkpoint: "TestGenerate3Len" test entry *** 1 failure is detected in the test module "TextWriter Tests"
Running 1 test case... TestGenerate1Len.cpp(25): fatal error: in "TestGenerate1Len": critical check s.size() == len has failed [1 != 10] *** 1 failure is detected in the test module "TextWriter Tests"
Running 1 test case... TestKRand3Freq.cpp(36): fatal error: in "TestKRand3Freq": critical check numA < trials * 2 / 5 has failed [485 >= 400] *** 1 failure is detected in the test module "TextWriter Tests"
Running 1 test case... TestFreqSpec1.cpp(26): fatal error: in "TestFreq3Plus": critical check writer.freq("a", 'g') == 5 has failed [4 != 5] *** 1 failure is detected in the test module "TextWriter Tests"
Running 1 test case... TestFreq3.cpp(23): fatal error: in "TestFreq3": critical check writer.freq("aga") == 3 has failed [2 != 3] *** 1 failure is detected in the test module "TextWriter Tests"
Step by Step Solution
There are 3 Steps involved in it
Step: 1
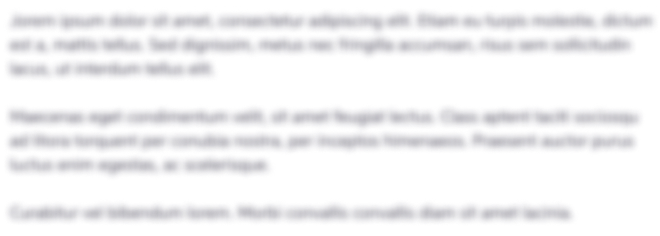
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started