Question
2A) Add a constructor for class KWArrayList that accepts an integer argument that represents the initial array capacity. Then, create an array list with capacity
2A) Add a constructor for class KWArrayList that accepts an integer argument that represents the initial array capacity. Then, create an array list with capacity 1 in class App_KWArrayList (already available):
KWArrayList myList = new KWArrayList(1);
Run the project, you will get output. Please complete the following tasks:
1) The reallocate() method was called how many times? Why reallocate() was called?
2) Add methods getSize() and getCapacity() to the KWArrayList class, and output the size and capacity of myList in the main method of the App_KWArrayList class through uncommenting the code block.
2B)Implement the indexOf method of the KWArrayList class. Then use this method to search Happy and return the index number. Output this index number in the main method of the App_KWArrayList class (The function of the method indexOf was described in TABLE 2.4 of the textbook).
App_KWArrayList Class
package A02_Q2;
public class App_KWArrayList {
public static void main(String[] args) {
//Uncomment the following code to test questions 2(a) and 2(b).
/* //2(a)
KWArrayList
myList.add("Bashful");
myList.add("Awful");
myList.add("Jumpy");
myList.add("Rain");
myList.add("Sun");
myList.add("Happy");
myList.add("Wind");
myList.add("Math");
myList.add("Java");
myList.add(2, "Doc1");
myList.add(3, "Doc2");
myList.set(2, "Doc3");
myList.add("Dopey");
myList.remove(3);
for (int i = 0; i < myList.getSize(); i++) {
System.out.printf("The item of index %d is %s. ", i, myList.get(i));
}
System.out.printf(" The size myList is %d.", myList.getSize());
System.out.printf(" The capacity myList is %d.", myList.getCapacity());
//2(b)
System.out.printf(" The array index of the item Happy is %d", myList.indexOf("Happy"));*/
}
}
KWArrayList
package A02_Q2;
import java.util.*;
/** This class implements some of the methods of the Java ArrayList class. It
does not implement the List interface.
*/
public class KWArrayList
// Data fields
/** The default initial capacity */
private static final int INITIAL_CAPACITY = 10;
/** The underlying data array */
private E[] theData;
/** The current size */
private int size = 0;
/** The current capacity */
private int capacity = 0;
@SuppressWarnings("unchecked")
public KWArrayList() {
capacity = INITIAL_CAPACITY;
theData = (E[]) new Object[capacity];
}
//Appends an item to the end of a KWArrayList
public boolean add(E anEntry) {
if (size == capacity) {
reallocate();
}
theData[size] = anEntry;
size++;
return true;
}
// Insert an item at a specified position of index, i.e., theData[index]
// Need to shift data in elements from index to size - 1
public void add(int index, E anEntry) {
if (index < 0 || index > size) {
throw new ArrayIndexOutOfBoundsException(index);
}
if (size == capacity) {
reallocate();
}
// Shift data in elements from index to size 1
for (int i = size; i > index; i--) {
theData[i] = theData[i - 1];
}
// Insert the new item.
theData[index] = anEntry;
size++;
}
public E get(int index) {
if (index < 0 || index >= size) {
throw new ArrayIndexOutOfBoundsException(index);
}
return theData[index];
}
public E set(int index, E newValue) {
if (index < 0 || index >= size) {
throw new ArrayIndexOutOfBoundsException(index);
}
E oldValue = theData[index];
theData[index] = newValue;
return oldValue;
}
public E remove(int index) {
if (index < 0 || index >= size) {
throw new ArrayIndexOutOfBoundsException(index);
}
E returnValue = theData[index];
for (int i = index + 1; i < size; i++) {
theData[i - 1] = theData[i];
}
size--;
return returnValue;
}
//Double the capacity of the array list
private void reallocate() {
capacity = 2 * capacity;
theData = Arrays.copyOf(theData, capacity);
}
//////////////////////////////////////////////////////////////////////////////
//Complete the following three methods for questions of a(2) and 3 of the assignment 2.
//You may don't need the statement "return -1" when you complete the methods.
//A new required constructor should be added here
//////////////////////////////////////////////////////////////////////////////
public int getSize() {
return -1;
}
public int getCapacity() {
return -1;
}
//int indexOf(E value)
//Searches for value and returns the position of the first occurrence, or 1
//if it is not in the List
public int indexOf(E value) {
return -1;
}
//////////////////////////////////////////////////////////////////////////////
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
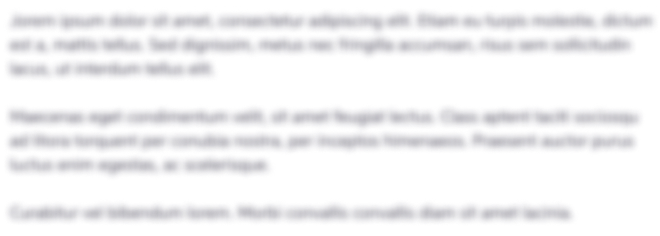
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started