Question
(2a) Model a physical entity as a Java Class (70 points) focus on Encapsulation : This is an Object Modeling/Programming exercise albeit around 1 (maybe
(2a) Model a physical entity as a Java Class (70 points) focus on Encapsulation:
This is an Object Modeling/Programming exercise albeit around 1 (maybe 2) Object(s). Using the Radio Class implementation provided as a point of reference, think of a common (or maybe, not so common) physical device or appliance thats worthy of modeling as an Object. Consider the following:
The basic operations/functionality of your device/appliance become public methods of the implementing Class (e.g., on, off, go, start, stop, )
The internal structure (or how you envision the internal structure) of your device/appliance become the data members of the implementing Class. This would include the internal piece-parts of the device and well as state components. For example, modeling a bread toaster might require a heat coil as well as a power on/off indicator.
In the spirit of modularity, reusability, etc., private/protected methods can and should be used to implement sub-tasks performed by public methods.
In addition to Class members supporting (a) (b) (c) above, you also need to implement:
Either a public void main(String [] ){} method or a second launch Class whose only job will be to:
Create an instance of your modeled Class
Invoke a sequence of methods on that instance that demonstrates the functionality and basic usefulness of what you built.
A String toString() method allowing you to display the state of the Object Instance.
What do we mean by the state of the Instance? The collective value of all data members at a moment in time is considered the instances state. Thats a valid definition of state, but for this lab, customize the Class toString() method to display values of data members that are considered significant to the device/applications operation.
Note: Refer to the Radio Class toString() and main() methods as a guide. Also refer to the Radios execution output below showing that a single instance has been created, and taken through a series of operations (method invocations). At critical points, a toString() representation of the instances state is displayed to the console using System.out.println()or the like.
Replace the output below with yours!
New Instance Radio Instance: [SerialNumber=1411918066216:43514652, powerState=false, selectedVolume=0, selectedStation=0.0, selectedBand=null, amPresets=null, fmPresets=null, firstTimeOn=null, lastTimeOn=null, selectedBalance=0, selectedBassLevel=0, selectedTrebleLevel=0] Turned On Radio Instance: [SerialNumber=1411918066216:43514652, powerState=true, selectedVolume=5, selectedStation=770.0, selectedBand=AM, amPresets=[563.0, 1080.0, 773.0, 730.0, 1192.0, 584.0, 608.0, 843.0], fmPresets=[99.0, 92.0, 101.0, 99.0, 88.0, 103.0, 92.0, 92.0, 92.0, 105.0, 99.0, 93.0], firstTimeOn=Sun Sep 28 11:27:46 EDT 2014, lastTimeOn=Sun Sep 28 11:27:46 EDT 2014, selectedBalance=0, selectedBassLevel=2, selectedTrebleLevel=3] Changed Station Radio Instance: [SerialNumber=1411918066216:43514652, powerState=true, selectedVolume=5, selectedStation=92.3, selectedBand=FM, amPresets=[563.0, 1080.0, 773.0, 730.0, 1192.0, 584.0, 608.0, 843.0], fmPresets=[99.0, 92.0, 101.0, 99.0, 88.0, 103.0, 92.0, 92.0, 92.0, 105.0, 99.0, 93.0], firstTimeOn=Sun Sep 28 11:27:46 EDT 2014, lastTimeOn=Sun Sep 28 11:27:46 EDT 2014, selectedBalance=0, selectedBassLevel=2, selectedTrebleLevel=3] Assign Preset [1] to + FM Radio Instance: [SerialNumber=1411918066216:43514652, powerState=true, selectedVolume=5, selectedStation=101.1, selectedBand=FM, amPresets=[563.0, 1080.0, 773.0, 730.0, 1192.0, 584.0, 608.0, 843.0], fmPresets=[101.1, 92.0, 101.0, 99.0, 88.0, 103.0, 92.0, 92.0, 92.0, 105.0, 99.0, 93.0], firstTimeOn=Sun Sep 28 11:27:46 EDT 2014, lastTimeOn=Sun Sep 28 11:27:46 EDT 2014, selectedBalance=0, selectedBassLevel=2, selectedTrebleLevel=3] Turned Off Radio Instance: [SerialNumber=1411918066216:43514652, powerState=false, selectedVolume=5, selectedStation=101.1, selectedBand=FM, amPresets=[563.0, 1080.0, 773.0, 730.0, 1192.0, 584.0, 608.0, 843.0], fmPresets=[101.1, 92.0, 101.0, 99.0, 88.0, 103.0, 92.0, 92.0, 92.0, 105.0, 99.0, 93.0], firstTimeOn=Sun Sep 28 11:27:46 EDT 2014, lastTimeOn=Sun Sep 28 11:27:46 EDT 2014, selectedBalance=0, selectedBassLevel=2, selectedTrebleLevel=3] |
/** the idea i am working on is a printer so please help me to finish its coding
//////Please edit the code and follow the lab instruction. thank you.
////////////////////////////////////
package edu.cuny.csi.csc330.lab4;
import java.text.Format;
import java.text.SimpleDateFormat;
import java.util.*;
import java.util.Scanner;
import edu.cuny.csi.csc330.lab4.Radio.Bands;
import edu.cuny.csi.csc330.lib.Randomizer;
public class Printer {
protected static final int MIN_PAPER = 0;
protected static final int MAX_PAPER = 500;
protected static final int MIN_INK = 0;
protected static final int MAX_INK = 100;
protected static final int MIN_PRINTED_PAPER = 0;
protected static final int MAX_PRINTED_PAPER = 50;
private Scanner input;
private Randomizer randomizer;
private boolean powerState;
private boolean staple = false;
private String serialNumber;
private int ink;
private int paper;
private int numComands;
private int selectedPapers[];
private Date firstTimeOn;
private Date lastTimeOn;
public Printer() {
init();
}
private void init() {
// serial number
Integer irand = randomizer.generateInt(11111111, 99999999);
this.serialNumber = new Date().getTime() + ":" + irand.toString();
}
private void on(boolean status) {
Date now = new Date();
// all the things we need to do the 1st time we turn on the instance ...
if(firstTimeOn == null) {
firstTimeOn = now;
// selectedVolume & station
setInk();
setPaper();
selectedPapers[numComands] = 0;
// tone and balance
selectedBalance = DEFAULT_BALANCE;
selectedTrebleLevel = DEFAULT_TREBLE;
selectedBassLevel = DEFAULT_BASS;
}
powerState = true;
lastTimeOn = now;
}
public Date getFirstTimeOn() {
return firstTimeOn;
}
public void off() {
powerState = false;
}
public boolean isOn() {
return powerState == true;
}
public void staple() {
staple = input.nextstring();
}
public void displayStatus() {
Format formatter=new SimpleDateFormat("EEE MMM dd yyyy HH:mm:ss z");
System.out.println(formatter.format(new Date()));
}
public int setInk() {
this.ink = randomizer.generateInt(MIN_INK, MAX_INK);
return ink;
}
public int setPaper() {
this.paper = randomizer.generateInt(MIN_PAPER, MAX_PAPER);
return paper;
}
public int[] getSelectedPapers() {
return selectedPapers;
}
public double getNumberComandsn() {
input = new Scanner(System.in);
numComands = input.nextInt();
return numComands;
}
public String toString() {
return "Printer Instance: "+", powerState=" + powerState + ", AmountInk="
+ ink + ", NumberOfPaper=" + paper
+ ", Numberofcommands =" + getNumberComandsn() + ", NumberOfpapers=" + getSelectedPapers()
+", firstTimeOn=" + firstTimeOn
+"Do you want your documents to be printed?(Y/N)"+staple
+ ", lastTimeOn=" + lastTimeOn
+ ", RemainingPapers= " + paper
+ ", RemainingInk=" + ink + " "+"";
}
public static void main(String[] args) {
// Create instance of - power up & down ... other ops, and
// display the powerState of the Radio instance
Printer printer = new Printer();
System.out.println("New Instance " + printer + " ");
// turn it on
printer.on();
System.out.println("Turned On " + printer + " ");
System.out.println("Changed Station " + printer + " ");
System.out.println("Assign Preset [1] to + " + printer.getSelectedBand() + " " + printer + " ");
// Turn off radio
printer.off();
System.out.println("Turned Off " + printer + " ");
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
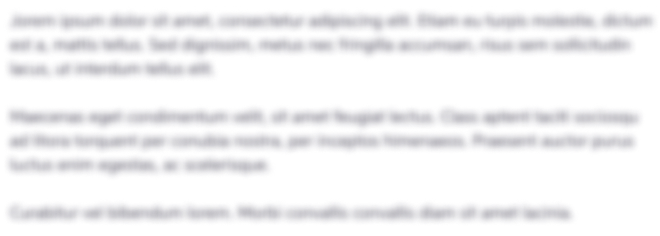
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started