Question
3I.3 The Client sends the radius to server Java Chapter 31 LISTING 31.1 Server.java 1 import java.io.*; 2 import java.net.*; 3 import java.util.Date; 4 import
3I.3 The Client sends the radius to server Java Chapter 31
LISTING 31.1 Server.java 1 import java.io.*; 2 import java.net.*; 3 import java.util.Date;
4 import javafx.application.Application; 5 import javafx.application.Platform; 6 import javafx.scene.Scene; 7 import javafx.scene.control.ScrollPane; 8 import javafx.scene.control.TextArea; 9 import javafx.stage.Stage; 10 11 public class Server extends Application { 12 @Override // Override the start method in the Application class 13 public void start(Stage primaryStage) { 14 // Text area for displaying contents 15 TextArea ta = new TextArea(); 16 17 // Create a scene and place it in the stage 18 Scene scene = new Scene(new ScrollPane(ta), 450, 200); 19 primaryStage.setTitle("Server"); // Set the stage title 20 primaryStage.setScene(scene); // Place the scene in the stage 21 primaryStage.show(); // Display the stage 22 23 new Thread(() -> { 24 try { 25 // Create a server socket 26 ServerSocket serverSocket = new ServerSocket(8000); 27 Platform.runLater(() -> 28 ta.appendText("Server started at " + new Date() + ' ')); 29 30 // Listen for a connection request 31 Socket socket = serverSocket.accept(); 32 33 // Create data input and output streams 34 DataInputStream inputFromClient = new DataInputStream( 35 socket.getInputStream()); 36 DataOutputStream outputToClient = new DataOutputStream( 37 socket.getOutputStream()); 38 39 while (true) { 40 // Receive radius from the client 41 double radius = inputFromClient.readDouble(); 42 43 // Compute area 44 double area = radius * radius * Math.PI; 45 46 // Send area back to the client 47 outputToClient.writeDouble(area); 48 49 Platform.runLater(() -> { 50 ta.appendText("Radius received from client: " 51 + radius + ' '); 52 ta.appendText("Area is: " + area + ' '); 53 }); 54 } 55 } 56 catch(IOException ex) { 57 ex.printStackTrace(); 58 } 59 }).start(); 60 } 61 }
1 import java.io.*; 2 import java.net.*; 3 import javafx.application.Application; 4 import javafx.geometry.Insets; 5 import javafx.geometry.Pos; 6 import javafx.scene.Scene; 7 import javafx.scene.control.Label; 8 import javafx.scene.control.ScrollPane; 9 import javafx.scene.control.TextArea; 10 import javafx.scene.control.TextField; 11 import javafx.scene.layout.BorderPane; 12 import javafx.stage.Stage; 13 14 public class Client extends Application { 15 // IO streams 16 DataOutputStream toServer = null; 17 DataInputStream fromServer = null; 18 19 @Override // Override the start method in the Application class 20 public void start(Stage primaryStage) { 21 // Panel p to hold the label and text field 22 BorderPane paneForTextField = new BorderPane(); 23 paneForTextField.setPadding(new Insets(5, 5, 5, 5)); 24 paneForTextField.setStyle("-fx-border-color: green"); 25 paneForTextField.setLeft(new Label("Enter a radius: ")); 26 27 TextField tf = new TextField(); 28 tf.setAlignment(Pos.BOTTOM_RIGHT); 29 paneForTextField.setCenter(tf); 30 31 BorderPane mainPane = new BorderPane(); 32 // Text area to display contents 33 TextArea ta = new TextArea(); 34 mainPane.setCenter(new ScrollPane(ta)); 35 mainPane.setTop(paneForTextField); 36 37 // Create a scene and place it in the stage 38 Scene scene = new Scene(mainPane, 450, 200); 39 primaryStage.setTitle("Client"); // Set the stage title 40 primaryStage.setScene(scene); // Place the scene in the stage 41 primaryStage.show(); // Display the stage 42 43 tf.setOnAction(e -> { 44 try { 45 // Get the radius from the text field 46 double radius = Double.parseDouble(tf.getText().trim()); 47 48 // Send the radius to the server 49 toServer.writeDouble(radius); 50 toServer.flush(); 51 52 // Get area from the server 53 double area = fromServer.readDouble(); 54 55 // Display to the text area 56 ta.appendText("Radius is " + radius + " "); 57 ta.appendText("Area received from the server is " 58 + area + ' ');
59 } 60 catch (IOException ex) { 61 System.err.println(ex); 62 } 63 }); 64 65 try { 66 // Create a socket to connect to the server 67 Socket socket = new Socket("localhost", 8000); 68 // Socket socket = new Socket("130.254.204.36", 8000); 69 // Socket socket = new Socket("drake.Armstrong.edu", 8000); 70 71 // Create an input stream to receive data from the server 72 fromServer = new DataInputStream(socket.getInputStream()); 73 74 // Create an output stream to send data to the server 75 toServer = new DataOutputStream(socket.getOutputStream()); 76 } 77 catch (IOException ex) { 78 ta.appendText(ex.toString() + ' '); 79 } 80 } 81 }
FIGURE 31.3 The client sends the radius to the server; the server computes the area and sends it to the client. The client sends the radius through a DataoutputStream on the output stream socket and the server receives the radius through the DataInputstream on the input stream socket, as shown in Figure 31.4a. The server computes the area and sends it to the client through a DataoutputStream on the output stream socket, and the client receives the area through a DataInputStream on the input stream socket, as shown in Figure 31.4b. The server and client programs are given in Listings 31.1 and 31.2. Figure 31.5 contains a sample run of the server and the client Server Client Server Client radius radius area area DataInputstream DataInputstream Dataoutputstream Dataoutputstream socket. getInputStream socket.getoutput Stream socket. getoutputStream socket.getInputStream socket socket socket socket Network t------- Network (a) (b) FIGURE 31.4 (a) The client sends the radius to the server. (b) The server sends the area to the client. Server started at Tue Ap r 16 17:34:02 EDT 2013 Enter a radius: 5.5 Radius received from client: 4.5 Area is: 63.61725123519331 Radius is -1.5 Radius received from client: 5.5 Area received from the server is 63.61725123519331 Area is: 95.03317777109125 Radius is 5.5 Area received from the server is 95.03317777109125 FIGURE 31.3 The client sends the radius to the server; the server computes the area and sends it to the client. The client sends the radius through a DataoutputStream on the output stream socket and the server receives the radius through the DataInputstream on the input stream socket, as shown in Figure 31.4a. The server computes the area and sends it to the client through a DataoutputStream on the output stream socket, and the client receives the area through a DataInputStream on the input stream socket, as shown in Figure 31.4b. The server and client programs are given in Listings 31.1 and 31.2. Figure 31.5 contains a sample run of the server and the client Server Client Server Client radius radius area area DataInputstream DataInputstream Dataoutputstream Dataoutputstream socket. getInputStream socket.getoutput Stream socket. getoutputStream socket.getInputStream socket socket socket socket Network t------- Network (a) (b) FIGURE 31.4 (a) The client sends the radius to the server. (b) The server sends the area to the client. Server started at Tue Ap r 16 17:34:02 EDT 2013 Enter a radius: 5.5 Radius received from client: 4.5 Area is: 63.61725123519331 Radius is -1.5 Radius received from client: 5.5 Area received from the server is 63.61725123519331 Area is: 95.03317777109125 Radius is 5.5 Area received from the server is 95.03317777109125Step by Step Solution
There are 3 Steps involved in it
Step: 1
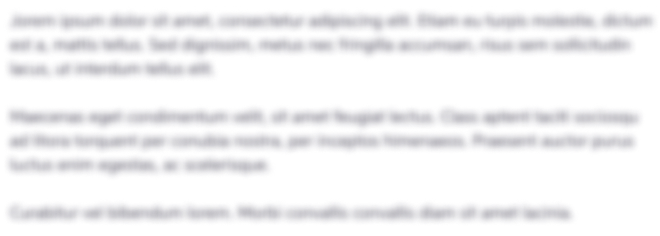
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started