Answered step by step
Verified Expert Solution
Question
1 Approved Answer
4 . 1 2 LAB 3 : Classes ( Rational ) Rational Numbers It may come as a bit of a surprise when the C
LAB : Classes Rational
Rational Numbers
It may come as a bit of a surprise when the C floatingpoint types float double fail to capture a particular value accurately. Certainly double, which is usually stored as a bit value, is far better than the old float, which is only bits, but problems do arise. For example:
float n ;
float d ;
cout precision;
cout n d endl;
produces which is accurate to only decimal places a bit dirty for a discipline that prides itself on precision!
A solution that is often used when precision is of greatest importance and all of the numbers involved are going to be "rational" that is expressible as a 'ratio' of two integers ie a fraction is to use a custom data type ie a class that implements fractions, or "rational numbers". This is what you will do in this lab assignment.
Class Specification
Write a C program that performs the rational number operations addition, subtraction, multiplication and division on two fractions. The program should be written in a single file. You will need to design a "rational number" class named Rational whose value will be a fraction eg or with appropriate constructors and member functions. A fraction will be specified as a numerator and a denominator eg the pair represents the fraction The member variables should be private and accessed using the accessor and mutator functions.
Constructors
Create constructors:
a constructor with two parameters numerator and denominator
a constructor with one parameter denominator set to
a constructor with no parameters
Accessor Functions
add
subtract
multiply
divide
display
Mutator Function
simplify
The following are a list of the rules of arithmetic for fractions:
abcdad bcbd
abcdad bcbd
abcdacbd
abcdadcb
Note that for this lab, when you perform an operation, you do not need to simplify the resulting fraction, ie You should not simplify this to at this point.
The display function should output the Rational object in the format:
n d
#include
using namespace std;
Rational Class declaration
class Rational
private:
int numerator;
int denominator;
public:
Rational;
explicit Rationalint;
Rationalint int;
Rational addconst Rational & const;
Rational subtractconst Rational & const;
Rational multiplyconst Rational & const;
Rational divideconst Rational & const;
void simplify;
void display const;
private:
int gcdint int const;
;
Implement Rational class member functions here
Do not change any of the code below this line!!
Rational getRational;
void displayResultconst string & const Rational & const Rational& const Rational&;
int main
Rational A B result;
char choice;
cout "Enter Rational A: endl;
A getRational;
cout endl;
cout "Enter Rational B: endl;
B getRational;
cout endl;
cout "Enter Operation:" endl
a Addition A B endl
s Subtraction A B endl
m Multiplication A B endl
d Division A B endl
y Simplify A endl;
cin choice;
cout endl;
if choice a
result AaddB;
displayResult A B result;
else if choice s
result AsubtractB;
displayResult A B result;
else if choice m
result AmultiplyB;
displayResult A B result;
else if choice d
result AdivideB;
displayResult A B result;
else if choice y
Asimplify;
Adisplay;
else
cout "Unknown Operation";
cout endl;
return ;
Rational getRational
int choice;
int numer, denom;
cout "Which Rational constructor? Enter or endl
parameters numerator & denominator endl
parameter numerator endl
parameters default endl;
cin choice;
cout endl;
if choice
cout "numerator? ;
cin numer;
cout endl;
cout "denominator? ;
cin denom;
cout endl;
return Rationalnumer denom;
else if choice
cout "numerator? ;
cin numer;
cout endl;
return Rationalnumer;
else
return Rational;
void displayResultconst string &op const Rational &lhs const Rational&rhs const Rational &result
cout ;
lhsdisplay;
cout op ;
rhsdisplay
Step by Step Solution
There are 3 Steps involved in it
Step: 1
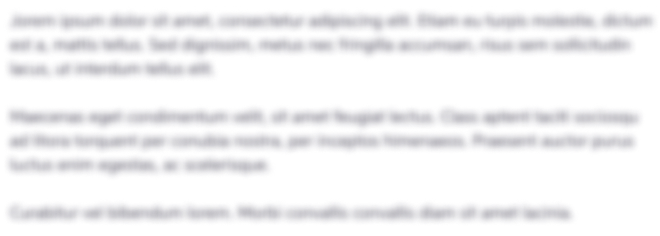
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started