Question
5.1a Use either the Insertion Sort or Bubble Sort to sort an array/vector of Accounts by account name. This can be a local function in
5.1a Use either the Insertion Sort or Bubble Sort to sort an array/vector of Accounts by account name. This can be a local function in main.cpp, but will need the Account header and cpp files to use the Account type.
5.1b Test the sort function and print out the array/vector after sorting to verify that the names are in order.
5.2a Use a binary search in the findTicket member function of the MovieTheater class to find a ticket number in the soldTickets array.
5.2b Test the binary search function. Try to find a ticket that you know exists, then try to find one that you know does not exist. Submission: main.cpp and MovieTheater.cpp file Due: Thursday, March 7, 2019 by end of day
_______________
Main.cpp
_______________
#include "Account.h"
#include
#include
#include
#include
#include
#include
using namespace std;
https://repl.it/@jholst/6
void printArray(int arr[], int size){
int count = 1;
for (int i = 0; i < size; i++){
cout << setw(3) << arr[i];
if (count % 10 == 0){
cout << " ";
}
else{
cout << " ";
}
count++;
}
cout << " ";
}
void printAccounts(vector
for (int i = 0; i < accts.size(); i++){
cout << accts[i]->getName() << "\t"
<< accts[i]->getBalance() << endl;
}
}
int binarySearch(const int numbers[], const int size, const int searchvalue){
int position = -1; // initialize to invalid
int low = 0;
int high = size - 1;
int middle = (low + high + 1)/2;
do{
if (searchvalue == numbers[middle])
position = middle;
else if (searchvalue < numbers[middle])
high = middle - 1;
else
low = middle + 1;
middle = (low + high + 1) / 2;
} while (low <= high && position == -1);
return position;
}
int linearSearch(const int numbers[], const int size, const int searchvalue){
for (int i = 0; i < size; i++){
if (searchvalue == numbers[i]){
return i;
}
}
return -1;
}
void insertionSort(int numbers[], const int size){
for (int i = 0; i < size; i++){
int insert = numbers[i]; // save value
int moveTo = i; // location to move value to
while ((moveTo > 0) && (numbers[moveTo - 1] > insert)){
numbers[moveTo] = numbers[moveTo - 1];
moveTo--;
}
numbers[moveTo] = insert;
}
}
void insertionSort(vector
for (int i = 0; i < accts.size(); i++){
Account* insert = accts[i];
int moveTo = i;
while ((moveTo > 0) && (accts[moveTo - 1]->getBalance() > insert->getBalance())){
accts[moveTo] = accts[moveTo - 1];
moveTo--;
}
accts[moveTo] = insert;
}
}
void swap(int* a, int* b){
int temp = *a;
*a = *b;
*b = temp;
}
void bubbleSort(int arr[], int size){
bool swapped;
do {
swapped = false;
for (int i = 0; i < size - 1; i++){
if (arr[i] > arr[i + 1]){
swap(&arr[i], &arr[i+1]);
swapped = true;
} // end if
}// end for
} while (swapped == true);
}
int main() {
srand(time(0));
const int size = 10;
int myArray[size];
int userArraySize;
cout << "Enter array size: ";
cin >> userArraySize;
int * numbers = new int[userArraySize];
for (int i = 0; i < userArraySize; i++){
numbers[i] = rand() % 1000;
}
printArray(numbers, userArraySize);
bubbleSort(numbers, userArraySize);
printArray(numbers, userArraySize);
// test linear search
int search = 500;
int position = linearSearch(numbers, userArraySize, search);
if (position != -1){
cout << search << " found at position " << position << " ";
}
else{
cout << search << " not found in linear search. ";
}
position = binarySearch(numbers, userArraySize, search);
if (position != -1){
cout << search << " found at position " << position << " ";
}
else{
cout << search << " not found in binary search. ";
}
delete[] numbers;
vector
char accountID = 'E';
for (int i = 1; i <= 5; i++){
int startBal = rand() % 1000;
string name{accountID, 1};
name = name + "_Account";
Account* temp = new Account{name, startBal};
accounts.push_back(temp);
accountID -= 1;
}
printAccounts(accounts);
insertionSort(accounts);
printAccounts(accounts);
}
_____________
Account.cpp
____________
#include "Account.h"
Account::Account() {}
Account::Account(std::string accountName, int startingBalance)
: name{accountName}
{
if (startingBalance > 0)
balance = startingBalance;
}
void Account::deposit(int depositAmount){
if (depositAmount > 0)
balance += depositAmount;
}
void Account::withdraw(int withdrawAmount){
if (withdrawAmount > 0 && withdrawAmount <= balance)
balance -= withdrawAmount;
}
int Account::getBalance() const {
return balance;
}
void Account::setName(std::string accountName){
name = accountName;
}
std::string Account::getName() const {
return name;
}
______________
Account.h
______________
#include
#ifndef ACCOUNT_H
#define ACCOUNT_H
class Account {
public:
Account(std::string, int);
Account();
void deposit(int);
void withdraw(int);
int getBalance() const;
void setName(std::string);
std::string getName() const;
private:
std::string name;
int balance{0};
};
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
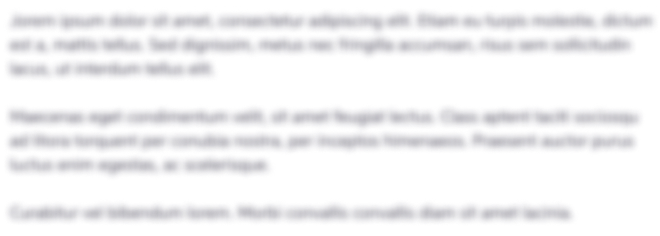
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started