Question
7.2 LAB: Bank accounts (implementing a class hierarchy) For this lab problem, you will be implementing a class hierarchy to represent bank accounts. Your hierarchy
7.2 LAB: Bank accounts (implementing a class hierarchy)
For this lab problem, you will be implementing a class hierarchy to represent bank accounts. Your hierarchy will consist of a class BankAccount and two subclasses of BankAccount: SavingsAccount and CheckingAccount.
Task 1: Create class BankAccount
- Create a class BankAccount.
- Its constructor should take in as parameters a persons name and a balance, stored as a double.
- Implement methods getName and getBalance
- Implement deposit and withdraw methods to add and subtract a specified amount from the balance.
- Furthermore, implement a transfer method, that takes another BankAccount instance and a specified amount, and transfers that amount from the original BankAccount to the one that is given.
- Finally, override the toString method to return a string containing the name and balance of the account.
Example output for toString:
BankAccount account = new BackAccount("Shrek", 5.0); System.out.println(accout.toString());
Should output
Shrek, $5.00
Task 2: Create derived class SavingsAccount
- Create another class named SavingsAccount.
- This should be a subclass of BankAccount.
- Implement a constructor for SavingsAccount that takes in an interest rate in addition to the name and balance. Example SavingsAccount constructor call with a balance of $200.00 and a 5% interest rate:
SavingsAccount savings = new SavingsAccount(Fiona, 200.0, 5.0);
- Finally, implement a method, addInterest, that deposits interest into account, based on the account balance and interest rate.
Task 3: Create derived class CheckingAccount
- Create another subclass of BankAccount named CheckingAccount.
- Create a field `transactionCount which keeps track of the number of transactions made (deposits and withdrawals).
- Create a static final field, TRANSACTION_FEE, and initialize it to 3.0.
- This checkings account charges a fee of $3.00 for every single transactino
- Finally, create a method, deductFees, that deducts fees from the account balance based on the number of transactions.
- This method should reset the transactionCount to zero.
Task 4
Finish implementing BankAccountTest as follows.
- Construct a SavingsAccount from savings parameters read from input.
- Construct a CheckingAccount from checkings parameters read from input.
- Deposit $1000 into the SavingsAccount.
- Withdraw $100 from the CheckingAccount.
- Now transfer $200 from the SavingsAccount into the CheckingAccount.
- Deduct the fees from the CheckingAccount.
- Add the interest to the SavingsAccount.
- Print out the SavingsAccount.
- Print out the CheckingAccount
Input:
Satoshi 8800000000.0 1.0 Maus 1000.0
Output:
Satoshi, $8888000808.00 Maus, $1094.00
GIVEN CODE
public class BankAccount {
/* Implement class BankAccount here. */
}
---------------------------------------------------------
/* Define class CheckingAccount here */
-----------------------------------------------------------
/* Define class SavingsAccount here. */
----------------------------------------------------------
import java.util.Scanner;
public class BankAccountTest { public static void main(String[] args) { Scanner scn = new Scanner(System.in); // Read in Savings Parameters String savingsName = scn.next(); double savingsBalance = scn.nextDouble(); double savingsInterest = scn.nextDouble(); // Read in Checkings Parameters String checkingsName = scn.next(); double checkingsBalance = scn.nextDouble(); /* Type your code here. */ } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
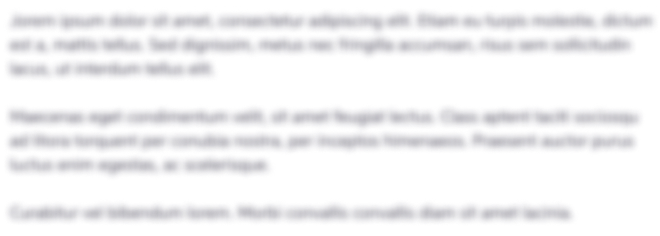
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started