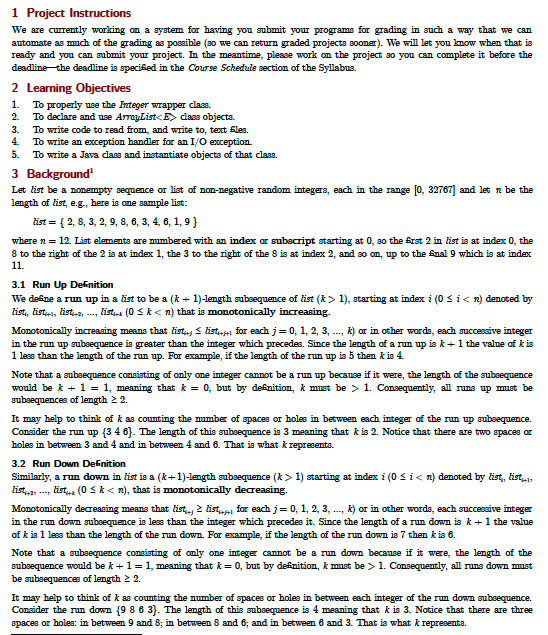
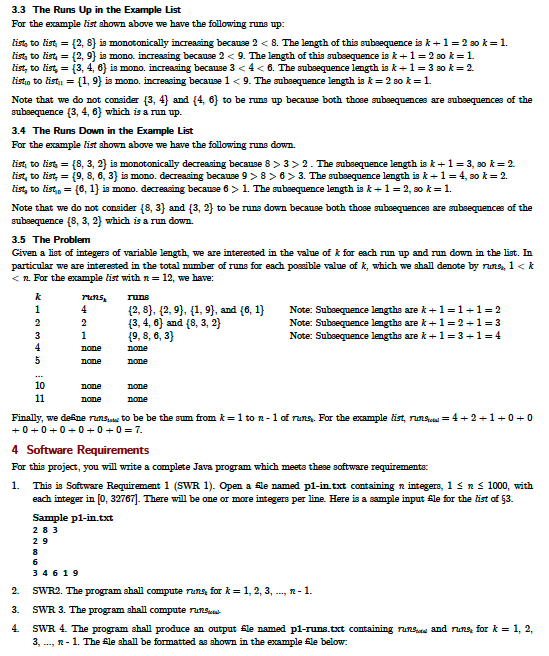
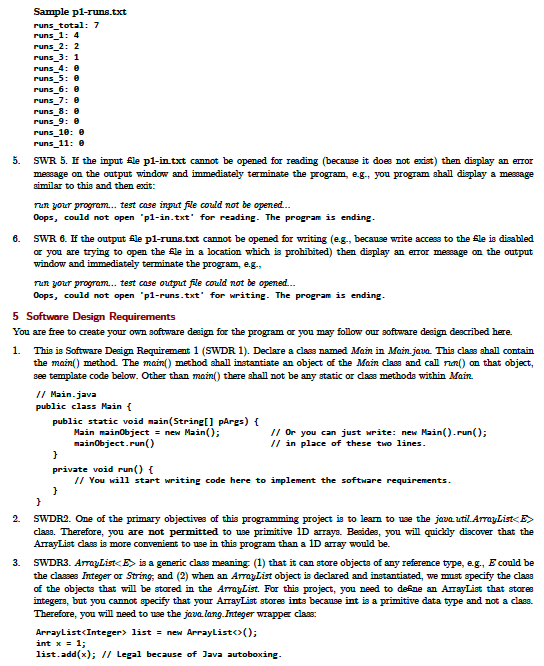
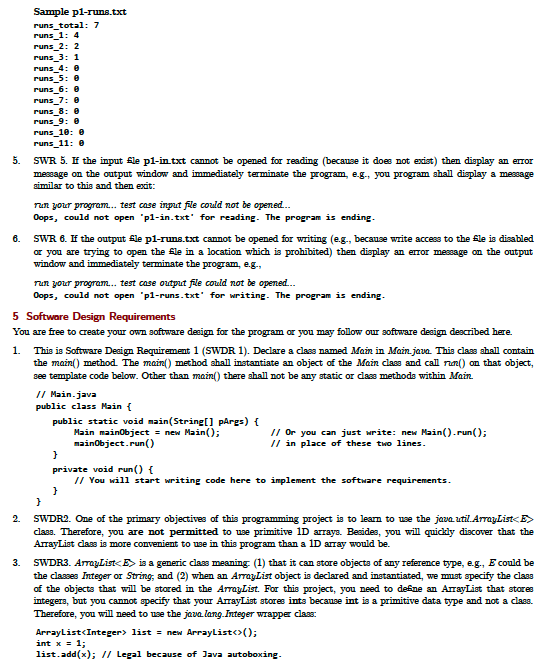
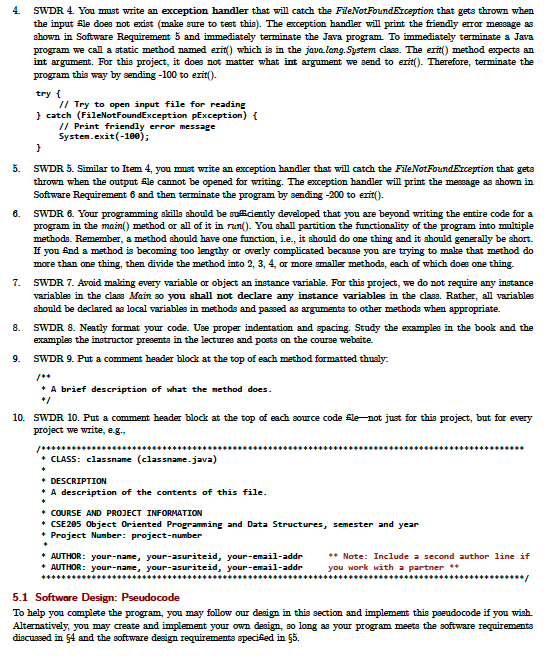
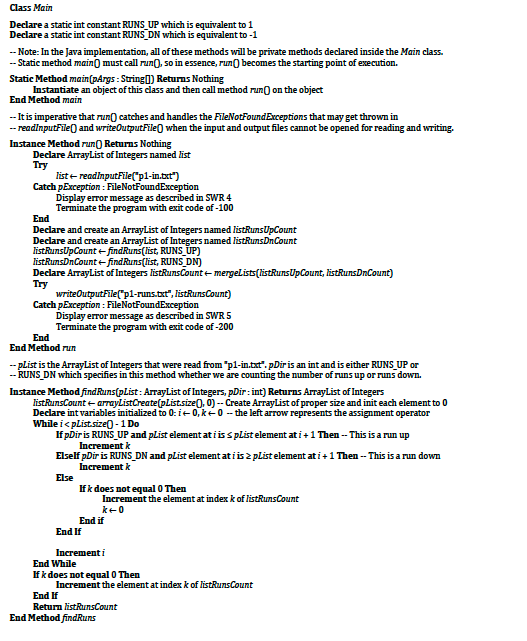
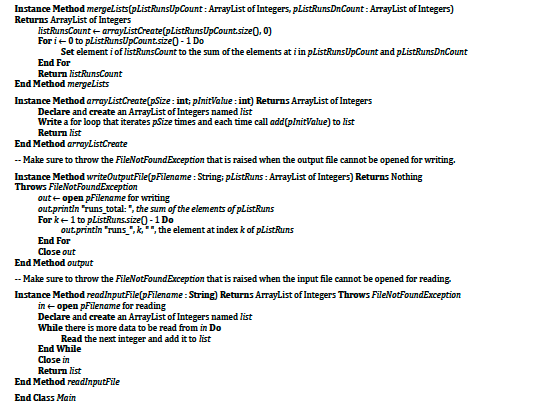
9 1 Project Instructions We are currently working on a system for having you submit your programs for grading in such a way that we can automate as much of the grading as possible (30 we can return graded projects sooner). We will let you know when that is ready and you can submit your project. In the meantime, please work on the project 30 you can complete it before the deadline the deadline is specifed in the Course Schedule section of the Syllabus. 2 Learning Objectives 1. To properly use the Integer wrapper class. 2. To declare and we ArrayList
dass objecta. 3. To write code to read from, and write to, text Sles. To write an exception handler for an I/O exception. 5. To write a Java case and instantiate objects of that class. 3 Background" Let list be a nonempty sequence or list of non-negative random integers, each in the range (0, 32767] and let n be the length of list, e.g, here is one sample list: list = { 2, 8, 3, 2, 9, 8, 6, 3, 4, 6, 1,9} where n = 12. List elements are numbered with an index or subscript starting at 0, so the Erst 2 in list is at index 0, the 8 to the right of the 2 is at index 1, the 3 to the right of the 8 is at index 2, and so on, up to the Enal 9 which is at index 11. 3.1 Run Up Definition We deene a run up in a list to be a (x + 1)-length subsequence of fist (k> 1), starting at index i (0 Si 1. Consequently, all runs up must be subsequences of length 22. It may help to think of k as counting the number of spaces or hole in between each integer of the run up subsequence. Consider the run up (3 4 6). The length of this subsequence is 3 meaning that kis 2. Notice that there are two spaces a holes in between 3 and 4 and in between 4 and 6. That is what k represents. 3.2 Run Down Definition Similarly, a run down in list is a (x+1)-length subsequence (x > 1) starting at index i (0 Si 1. Consequently, all runs down must be subsequences of length 2 2. It may help to think of k as counting the number of spaces or holes in between each integer of the run down subsequence. Consider the run down 19 8 6 3). The length of this subsequence is 4 meaning that kis 3. Notice that there are three spaces or holes: in between 9 and 8; in between 8 and 6, and in between 6 and 3. That is what k representa. 3.3 The Runs Up in the Example List For the example list shown above we have the following runs up: list, to tiste = {2, 8) is monotonically increasing because 2 3>2. The subsequence length is k+1=3, 20 k = 2. list, to list, = {9, 8, 6, 3} is mono. decreasing because 9 >8>6> 3. The subsequence length is k +1 = 4,30 k = 2. list, to listo = {6, 1} is mono. decreasing because 6 > 1. The subsequence length is k=1=2, 30 k=1. Note that we do not consider {8, 3} and (3, 2) to be runs down because both those subsequences are subsequences of the subsequence (8, 3, 2) which is a run down. 3.5 The Problem Given a list of integers of variable length we are interested in the value of k for each run up and run down in the list. In particular we are interested in the total number of runs for each possible value of k, which we shall denote by rus, 1 class. Therefore, you are not permitted to use primitive 1D arrays. Besides, you will quickly discover that the ArrayList dass is more convenient to use in this program than a 1D array would be. 3. SWDR3. ArrayList is a generic class meaning: (1) that it can store objects of any reference type, e.g., E could be the classes Integer or String, and (2) when an ArrayList object is declared and instantiated, we must specify the clasa of the objects that will be stored in the ArrayList. For this project, you need to dene an ArrayList that stores integers, but you cannot specify that you ArrayList stores ints because int is a primitive data type and not a class. Therefore, you will need to use the java.lang. Integer wrapper class: ArrayList list = new ArrayList(); int x = 1; list.add(x); // Legal because of Java autoboxing. 2 Sample p1-runs.txt runs_total: 7 runs 1: 4 runs_2: 2 runs 3: 1 runs_4: runs 5: @ runs 6: @ runs_7: runs_8: runs 9: runs_19: runs_11: SWR 5. F the input Ele pl-in txt cannot be opened for reading (because it does not exist) then display an error message on the output window and immediately terminate the program, e.g-, you program shall display a message similar to this and then exat: run your program... test case input file could not be opened.. Oops, could not open 'p1-in.txt' for reading. The program is ending. 5. 6. SWR 6. If the output sle p1-runs.txt cannot be opened for writing (e.g., because write access to the fle is disabled or you are trying to open the fle in a location which is prohibited) then display an error message on the output window and immediately terminate the program, e.g., run your program... test case output file could not be opened... Oops, could not open 'pl-runs.txt' for writing. The program is ending. 5 Software Design Requirements You are free to create your own software design for the program or you may follow our software design described here. 1. This is Software Design Requirement 1 (SWDR 1). Declare a class named Main in Main.java. This class shall contain the main() method. The main() method shall instantiate an object of the Main class and call run on that object, see template code below. Other than main() there shall not be any static or dae methods within Main. // Main.java public class Main { public static void main(String[] pArgs) { Main mainObject = new Main(); 1/ Or you can just write: new Main().run(); main object.run() // in place of these two lines. } private void run() { // You will start writing code here to implement the software requirements. } } SWDR2. One of the primary objectives of this programming project is to learn to we the java util.ArrayList class. Therefore, you are not permitted to use primitive 1D arrays. Besides, you will quickly discover that the ArrayList dass is more convenient to use in this program than a 1D array would be. 3. SWDR3. ArrayList is a generic class meaning: (1) that it can store objects of any reference type, e.g., E could be the classes Integer or String, and (2) when an ArrayList object is declared and instantiated, we must specify the clasa of the objects that will be stored in the ArrayList. For this project, you need to dene an ArrayList that stores integers, but you cannot specify that you ArrayList stores ints because int is a primitive data type and not a class. Therefore, you will need to use the java.lang. Integer wrapper class: ArrayList list = new ArrayList(); int x = 1; list.add(x); // Legal because of Java autoboxing. 2 4. SWDR 4. You must write an exception handler that will catch the FileNotFoundException that gets thrown when the input sle does not exist (make sure to test this). The exception handler will print the friendly error message 23 shown in Software Requirement 5 and immediately terminate the Java program To immediately terminate a Java program we call a static method named eritl) which is in the java.lang. System class. The erit() method expects an int argument. For this project, it does not matter what int argument we send to exit(). Therefore, terminate the program this way by sending -100 to exit(). try { 5. 6. // Try to open input file for reading } catch (FileNotFoundException pException) { // Print friendly error message System.exit(-100); } SWDR 5. Similar to Item 4, you must write an exception handler that will catch the FileNotFoundException that gets thrown when the output sle cannot be opened for writing. The exception handler will print the message as shown in Software Requirement 6 and then terminate the program by sending -200 to exit(). SWDR 6. Your programming skills should be recently developed that you are beyond writing the entire code for a program in the main() method or all of it in rund). You shall partition the functionality of the program into multiple methods. Remember, a method should have one function, i.e., it should do one thing and it should generally be short. If you and a method is becoming too lengthy or overly complicated because you are trying to make that method do more than one thing, then divide the method into 2, 3, 4, or more smaller methods, each of which does one thing. SWDR 7. Avoid making every variable or object an instance variable. For this project, we do not require any instance variable in the class Main 30 you shall not declare any instance variables in the class. Rather, all variables should be declared a local variables in methods and passed 22 arguments to other methods when appropriate. SWDR 8. Neatly format you code. Use proper indentation and spacing. Study the examples in the book and the examples the instructor presents in the lectures and posts on the course website. SWDR 9. Put a comment header block at the top of each method formatted thusly. 7. 8. 9. /** * A brief description of what the method does. +/ 10. SWDR 10. Put a comment header block at the top of each source code flenot just for this project, but for every project we write, e.g. /********************************** + CLASS: classname (classname.java) + + DESCRIPTION A description of the contents of this file. + + COURSE AND PROJECT INFORMATION CSE 205 Object Oriented Programming and Data Structures, semester and year Project Number: project-number AUTHOR: your-name, your-asuriteid, your-email-addr ** Note: Include a second author line if AUTHOR: your-name, your-asuriteid, your-email-addr you work with a partner ** / 5.1 Software Design: Pseudocode To help you complete the program, you may follow our design in this section and implement this peeudocode if you wish Alternatively, you may create and implement your own design, so long as your program meets the software requirements discussed in 54 and the software design requirements specifed in 55. Class Main Declare a static int constant RUNS_UP which is equivalent to 1 Declare a static int constant RUNS DN which is equivalent to-1 -- Note: In the Java implementation, all of these methods will be private methods declared inside the Main class. -- Static method main() must call run, so in essence, run becomes the starting point of execution. Static Method main(pArgs : String) Returns Nothing Instantiate an object of this class and then call method run on the object End Method main -- It is imperative that runcatches and handles the FileNotFoundExceptions that may get thrown in -- readinputfile() and writeOutputFile when the input and output files cannot be opened for reading and writing, Instance Method run Returns Nothing Declare ArrayList of Integers named list Try list readinputFile("p1-in.txt") Catch pException : FileNotFoundException Display error message as described in SWR 4 Terminate the program with exit code of -100 End Declare and create an ArrayList of Integers named listRunsUpCount Declare and create an ArrayList of Integers named listRunsDnCount list RunsUpCount-findRuns(list, RUNS_UP) listRunsDnCount=find Runs (list, RUNS_DN) Declare ArrayList of Integers listRunscount-mergelists(listRunsUpCount, listRunsDnCount) Try writeOutputFile("pl-runs.txt", list unscount) Catch pException: FileNotFoundException Display error message as described in SWR 5 Terminate the program with exit code of -200 End End Method run --plist is the ArrayList of Integers that were read from "p1-in.txt.pDir is an int and is either RUNS_UP or -- RUNS_DN which specifies in this method whether we are counting the number of runs up or runs down. Instance Method find Runs(pList: ArrayList of Integers, pDir: int) Returns ArrayList of Integers listRunsCount = arrayListCreate(pList.size0.0) -- Create ArrayList of proper size and init each element to 0 Declare int variables initialized to 0:i0,k0 -- the left arrow represents the assignment operator While i<><>