Question
9. In an array-based implementation of a queue, a possible solution to dealing with the full condition is to a. maintain a count of queue
9. In an array-based implementation of a queue, a possible solution to dealing with the full condition
is to
a. maintain a count of queue items
b. check for frontIndex equal to backIndex
c. wait for an arrayFullExcetion to be thrown
d. all of the above
10. Given a stack variable stk which provides a push operation that places an integer on the top of the stack, and a pop operation which removes and returns the integer from the
top of the stack, what would the stack look like, after the following code executed?
for(int k = 1; k < 10; k++)
{
if (k % 3 == 0)
stk.push( k+ stk.pop( ) );
else
stk.push( k );
}
Answer:
11. Given a queue variable que which provides an add operation which places an integer at the back of the queue, and a get operation which removes and returns the integer from
the front of the queue, what would the queue look like after the following code executed?
for(int k = 1; k < 10; k++)
{
if (k % 3 == 0)
que.add( k+ que.get( ) );
else
que.add( k );
}
Answer:
14. What is the order of complexity of the following function? Why?
int func(unsigned n)
{
int sum = 0;
int p, m;
int q = 100;
p = n;
m = n;
while ( q > 0)
{
while (m > 0) {
n = p;
while ( n > 0 )
{
sum += n;
n = n/2;
}
m = m -1;
}
q = q 1;
}
return sum;
}
Answer:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
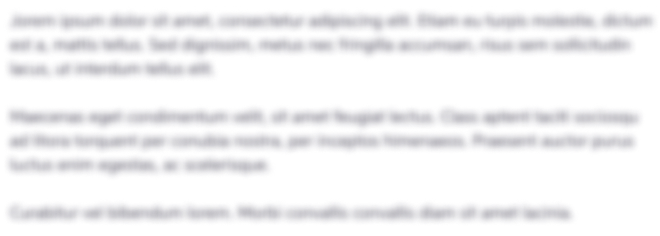
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started