Answered step by step
Verified Expert Solution
Question
1 Approved Answer
98. Do not modify constructor and any methods except in main method write your name in line 98. 99. Add bubble sort, insertion sort and
98. Do not modify constructor and any methods except in main method write your name in line 98.
99. Add bubble sort, insertion sort and selection sort methods to above program.
100. Modify each sort method that computes and prints no. of comparison and swaps after sorting. Add the following statement at the end of each sort method:
prt.printf(" \tComparisons = %d, Swaps = %d", ncomps, nswaps);
A sample input can be as follow:
50 15
200 184 125 175 151 129 106 152 163 168 106 166 105 162 107
171 740 176 501 194 174 115 108 445 154 139 140 150 193 128
159 157 186 315 190 142 191 108 151 113 148 164 150 171 144
176 191 114 124 171
1 import java.io.*;
2 import java.util.*;
3
4 // CSUN COMP 182 Sp 21, Homework-2
5 // Implementing bubble, insertion and selection sort algorithms
6 // and printing no. of comparisons and swaps.
7 // Author: xx
8 public class xxxxxH2{
9 // class variables
10 int arr[], barr[], n, k;
11 //varibles to count no. of comparisons and swaps
12 int nswap, ncomp;
13
14 //use a short word for System.out to save typing
15 PrintStream prt = System.out;
16
17 // class constructor
18 xxxxxH2 (){
19 int i;
20 prt.print("\tThis Java program implements bubble, insertion and selection sort \t" +
21 "algorithms and prints no. of comparisons and swaps. \t" +
22 "The program first reads n, k and next reads n integers and stores \t" +
23 "them in array barr[]. \tApplies bubble, insertion and selection sort to the same \t" +
24 "data and print no. of comparisons and swaps after sorting." +
25 " \t\tTo compile: javac xxxxxH2.java" +
26 " \t\tTo execute: java xxxxxH2 < any data file");
27
28 try{
29 // open input file inf
30 Scanner inf = new Scanner(System.in);
31 // read from input file
32 n = inf.nextInt();//read n, no. of data
33 k = inf.nextInt();//read k, how many per line
34
35 // Its a good practice to print input in program output!
36 prt.printf(" \tn = %3d, k = %4d ", n, k);
37
38 // Allocate space for arr
39 arr = new int[n];
40 barr = new int[n];
41 //loop to read n integers
42 for (i = 0; i < n ; i ++)
43 barr[i] = inf.nextInt();
44 // close input file inf
45 inf.close();
46 } catch (Exception e){ prt.printf(" Ooops! Read Exception: %s", e);}
47
48 } // end constructor
49
50 //Method to print formatted array,
51 //k elements per line
52 private void printarr(){
53 int j;
54 for (j = 0; j < n ; j ++){
55 prt.printf("%4d ", arr[j]);
56 if ((j+1) % k == 0)
57 prt.printf(" \t");
58 } // end for
59 } // end printarr
60
61 //swap arr[j] and arr[k]
62 private void swap(int j, int k){
63 int tmp = arr[j];
64 arr[j] = arr[k];
65 arr[k]= tmp;
66 } // end swap
67
68 // main program
69 public static void main(String args[]) throws Exception{
70 // Create an instance of xxxxxH2 class
71 xxxxxH2 h = new xxxxxH2();
72
73 //copy barr to arr for bubble sort,
74 h.arr = h.barr.clone();
75 System.out.printf(" \tInput before bubble sort \t");
76 h.printarr();
77 h.bubble();
78 System.out.printf(" \tInput after bubble sort \t");
79 h.printarr();
80
81 //copy barr to arr for insertion sort,
82 h.arr = h.barr.clone();
83 System.out.printf(" \tInput before insertion sort \t");
84 h.printarr();
85 h.insertion();
86 System.out.printf(" \tInput after insertion sort \t");
87 h.printarr();
88
89 //copy barr to arr for selection sort,
90 h.arr = h.barr.clone();
91 System.out.printf(" \tInput before selection sort \t");
92 h.printarr();
93 h.selection();
94 System.out.printf(" \tInput after selection sort \t");
95 h.printarr();
96
97 //MAKE SURE TO WRITE YOUR NAME IN NEXT LINE
98 System.out.printf(" \tAuthor: xxx Date: " +
99 java.time.LocalDate.now());
100 } // end main
101 } // end class xxxxxH2
Step by Step Solution
There are 3 Steps involved in it
Step: 1
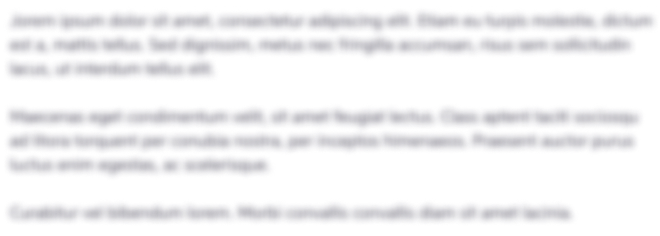
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started