Question
A bank operate savings account as per following condition: Savings accounts have a customer id, balance, and overdraft amount. A customer can withdraw an amount
A bank operate savings account as per following condition:
Savings accounts have a customer id, balance, and overdraft amount. A customer can withdraw an amount of money from the account, but only if they don't go over the overdraft amount. For example, if the balance is $100 and the overdraft is $200, the customer can withdraw no more than $300. The customer can also deposit an amount of money to the account. Please check the sample run of program to design your classes.
Following is the simple structure of abstract class for the program.
You can add more data member and methods in abstract class to accomplish the requirement of program.
pubic abstract class Accounts
{
private String customerID;
private double balance;
public Accounts(String customerID, double balance)
{
this.customerID = customerID;
this.balance = balance;
}
public abstract void deposit(double amount);
pubic abstract void withdraw(double amount);
OR
public abstract double deposit();
public abstract double withdraw();
OR
public abstract double deposit(double amount);
public abstract double withdraw(double amount);
You can use any format of abstract method to do deposit and withdraw.
}
Saving class is a subclasses of Account class.
Write a TestProgram to create object of saving class.
Use scanner class to enter value of customerID, balance, overdraft amount, value to be deposit and value to be withdrawn.
Print total balance after withdraw and deposit for saving account.
Use menu driven structure for saving account as per following.
Following is the sample run of program:
Please enter a customerID for the savings account: 101
Please enter a starting balance for the savings account: 100
Please enter the overdraft limit for the savings account: 100
1. Deposit Amount to Savings Account
2. Withdraw Amount from Savings Account
3. Exit
Choose a menu option for the savings account: 1
Please enter an amount to deposit to your savings account: 50
Amount of $50.0 deposited to account
Current account balance after deposit is $150.0
1. Deposit Amount to Savings Account
2. Withdraw Amount from Savings Account
3. Exit
Choose a menu option for the savings account: 2
Please enter an amount to withdraw from your savings account: 80
Amount of $80.0 successfully withdrawn
Current account balance after withdraw is $70.0
Current overdraft balance is $100.0
1. Deposit Amount to Savings Account
2. Withdraw Amount from Savings Account
3. Exit
Choose a menu option for the savings account: 2
Please enter an amount to withdraw from your savings account: 100
Overdraft funds required for transaction.
Amount of $100.0 successfully withdrawn
Current account balance is $0.0
Current overdraft balance is $70.0 and your original overdraft limit was: $100.0
1. Deposit Amount to Savings Account
2. Withdraw Amount from Savings Account
3. Exit
Choose a menu option for the savings account: 2
Please enter an amount to withdraw from your savings account: 100
Insufficient funds to complete transaction
Current account balance is $0.0
Current overdraft balance is 70.0
1. Deposit Amount to Savings Account
2. Withdraw Amount from Savings Account
3. Exit
Choose a menu option for the savings account: 3
Step by Step Solution
There are 3 Steps involved in it
Step: 1
Heres an example implementation of the Accounts abstract class and the Savings subclass as well as a Test Program that demonstrates the stated functionality import javautilScanner public abstract clas...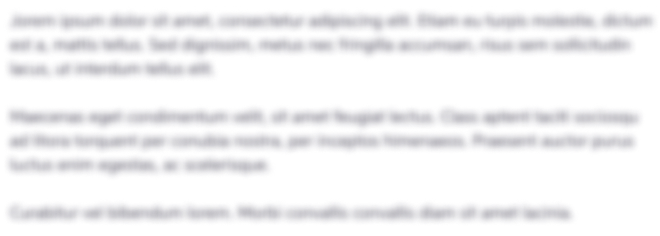
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started