Question
A CACHE CLASS: SHORT TERM MEMORY You will have a lot of freedom in this assignment in your design. You could manage your own heap
A CACHE CLASS: SHORT TERM MEMORY
You will have a lot of freedom in this assignment in your design. You could manage your own heap memory directly (more painful, but good practice), use different container classes (also good practice so you get familiar with the C++ Standard Library), etc. The main skills being learned in this assignment are work with templates.
This cache class should be templated based on the data type it stores, the number of items it can remember, i.e., store, and a means of comparing two elements. The idea is that it can be told to store items, but if it needs to remember something and it is already full then it will have to forget the oldest item. It can be told to remove items, in which case the oldest item will be removed.
There is one caveat here: it will store the high and low values of whatever data it stores. So even if those values are forgotten by the short term memory, they may still be remembered long term.
The class has iterators that can go from the most recent value back as far as there are values stored.
For example, suppose the class can store 3 int values and we sort them based on > so that 4 is considered higher than 7 (instead of sorting based on
Telling the structure to store: 2, 4, 6, 8, 10 will mean that it can only remember the numbers 10, 8, 6 (from most recent to most distant - only 3 numbers stored); in addition the high value would be 2 and the low value would be 10. If you are confused, read the instructions again about the data structure having its own comparison system.
Here are the specific requirements; everything should be in the pic10b namespace:
cache should be a templated class with...
three template parameters: a data type T, a number of elements it can store N, and a means of defining ordering ord that is by default std::less;
a default constructor that can also accept a value of type ord and set the member comparison with that;
a destructor (unless the compiler generated destructor works);
a copy constructor (unless the default copy mechanism generated by the compiler works);
a move constructor (unless the default move mechanism generated by the compiler works);
assignment operators (unless the default assignments generated by the compiler work);
an insert member function that accepts something of type T and remembers it, possibly at the cost of forgetting the most distant memory;
a templated emplace function that behaves as remember but can accept an arbitrary number of arguments of arbitrary type to construct an object and remember it;
a pop member function that forgets the most distant memory;
a begin member function returning an iterator to the most recent memory;
an end member function returning an iterator beyond the most distant memory (the usual past-the-end idea);
a size member function to count the number of memories (not counting the longterm storage); a get low member function to retrieve the lowest value; and a get high member function to retrieve the highest value.
You should also throw exceptions with appropriate messages should the user do something logically incorrect like calling pop when there are no memories, etc.
The iterator class should...
allow for prefix ++ and postfix ++;
include the dereferencing operator;
and include the arrow operator.
There should be a print that prints a cache object of any type, printing all the elements separated by spaces to the console. This function should not be variadic: it should accept a single memory object and print all of its elements. Period
You may assume that the objects T have default constructors (but if you worked more directly with memory like with allocators this would not be necessary).
An example of the desired output for the code below is provided.
#include "Cache.h" #include#include bool length_comp(const std::string& left, const std::string& right) { return left.size() intCache; // can store up to 3 memories of int plus high and low try { // can we pop with nothing there? intCache.pop(); } catch (const std::exception& e) { // apparently not... std::cerr stringCache(length_comp); stringCache.emplace(); // emtpy string added stringCache.insert("hey"); stringCache.insert("howdy"); stringCache.insert("salutations"); stringCache.insert("greetings"); stringCache.pop(); // now only stores "greetings" stringCache.emplace(4, '$'); // also stores "$$$$" std::cout size()
Some initial guidance to get you on your way...
1. Write the class in a more concrete ordering first: just use operator
2. If you use the C++ Standard data structures, you should not have to write a destructor, a copy constructor, a move constructor, or even assignment operators!
3. Be sure to draw diagrams! No matter what implementation you take, youll want nice diagrams!
4. Be very careful if a size t value is 0 and you decrease its value... you want to avoid that happening; if your logic requires something like that, treat the 0 case separately.
5. You can save yourself some grief and define everything within the namespace and everything within the first interface where it appears, i.e. fully define cache::iterator inside of the cache interface, dont define it outside, etc. You are still responsible to know how to define template nested classes/functions outside the interface, however.
6. Define the operator!= as a member within the memory::iterator class! When you work with templates, the compiler and linker will take any chance they can to attack you and you are less vulnerable to attack if things are tightly organized and kept together (even if the code itself looks worse).
7. You are allowed to make use of the C++ Standard containers if you want. Recall that many of those data structure are themselves built upon simpler data structures.
exception thrown forget nothing current: high and low: 33 4 3 print all the numbers: 37 27 4 After a forget: 37 27 recall highs and lows 373 element count 2 element count: 2 element count 2 printout of strings: $$$$ greetings size of a string 4 highs and lows of the strings lowest is emptysalutations exception thrown forget nothing current: high and low: 33 4 3 print all the numbers: 37 27 4 After a forget: 37 27 recall highs and lows 373 element count 2 element count: 2 element count 2 printout of strings: $$$$ greetings size of a string 4 highs and lows of the strings lowest is emptysalutations
Step by Step Solution
There are 3 Steps involved in it
Step: 1
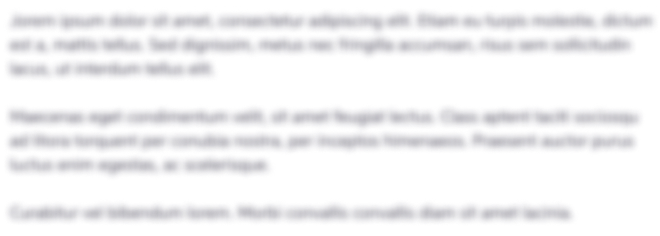
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started