Answered step by step
Verified Expert Solution
Question
1 Approved Answer
A circular doubly linked list is a linked list where every node has a next pointer and a previous pointer. The new thing that differentiates
A circular doubly linked list is a linked list where every node has a next pointer and a previous pointer. The new thing that differentiates this from a regular doubly linked list is that the header points to both the first and last elements of the List, making the references at the end of the list circle back to the beginning of the list.
Given the base code, create an implementation of the CircularDoublyLinkedList class with dummy header with the knowledge obtained from lectures and previous lab exercises. This includes the constructor and all the member methods.
Note: This List will have only a dummy header. Consider the case the list is empty, what would the header's next and previous point
import java.util.Iterator;
import java.util.NoSuchElementException;
public class ImplementCircularDoublyWrapper
public static interface List extends Iterable
public void addE elm;
public void addE elm, int index;
public boolean removeint index;
public boolean removeE elm;
public E getint index;
public E setE elm, int index;
public int size;
public int removeAllE elm;
public void clear;
public boolean containsE elm;
public int firstIndexE elm;
public int lastIndexE elm;
public boolean isEmpty;
public static class CircularDoublyLinkedList implements List
private class Node
private E value;
private Node next, prev;
public NodeE value, Node next, Node prev
this.value value;
this.next next;
this.prev prev;
public NodeE value
thisvalue null, null; Delegate to other constructor
public Node
thisnull null, null; Delegate to other constructor
public E getValue
return value;
public void setValueE value
this.value value;
public Node getNext
return next;
public void setNextNode next
this.next next;
public Node getPrev
return prev;
public void setPrevNode prev
this.prev prev;
public void clear
value null;
next prev null;
End of Node class
private class ListIterator implements Iterator
private Node nextNode;
public ListIterator
nextNode header.getNext;
@Override
public boolean hasNext
return nextNode header;
@Override
public E next
if hasNext
E val nextNode.getValue;
nextNode nextNode.getNext;
return val;
else
throw new NoSuchElementException;
End of ListIterator class, DO NOT REMOVE, TEST WILL FAIL
private fields
private Node header; "dummy" node
private int currentSize;
public CircularDoublyLinkedList
header new Nodenull;
TODO
What should the header point to
Remember the List is supposed to act like a circle
currentSize ;
@Override
public void addE obj
TODO ADD YOUR CODE HERE
@Override
public void addE elm, int index
TODO ADD YOUR CODE HERE
@Override
public boolean removeE obj
TODO ADD YOUR CODE HERE
return false;
@Override
public boolean removeint index
TODO ADD YOUR CODE HERE
return false;
@Override
public int removeAllE obj
TODO ADD YOUR CODE HERE
return ;
@Override
public E getint index
TODO ADD YOUR CODE HERE
return null;
@Override
public E setE elm, int index
TODO ADD YOUR CODE HERE
return null;
@Override
public int firstIndexE obj
TODO ADD YOUR CODE HERE
return ;
@Override
public int lastIndexE obj
TODO ADD YOUR CODE HERE
return ;
@Override
public int size
TODO ADD YOUR CODE HERE
return ;
@Override
public boolean isEmpty
TODO ADD YOUR CODE HERE
return false;
@Override
public boolean containsE obj
TODO ADD YOUR CODE HERE
return false;
@Override
public void clear
TODO ADD YOUR CODE HERE
@Override
public Iterator iterator
return new ListIterator;
DO NOT DELETE, TESTS WILL FAIL
public String toString
Node temp this.header.getNext;
String r ;
whiletemp header
r temp.getValue;
temp temp.getNext;
r rsubstring rlength;
return r;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
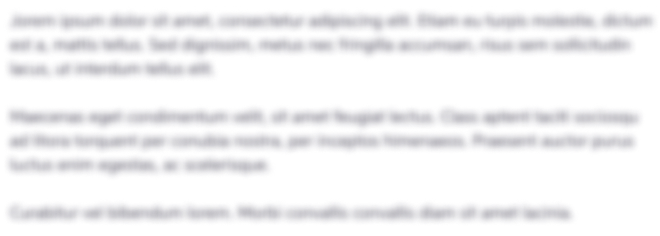
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started