Question
A collection of Interfaces that define Behavior Interfaces have many uses - (1) to prescribe certain behaviors, (2) to commonly identify all classes that implement
A collection of Interfaces that define Behavior
Interfaces have many uses - (1) to prescribe certain behaviors, (2) to commonly identify all classes that implement an interface, (3) to act as a functional interface that can be passed as a parameter.
In the next lesson, we will explore use #3. But we will use interfaces for the first two purposes in this assignment.
You will be building an application that combines different media collections from old style TV shows. Each TV show's collection will only be of one specific file type. You will build a class specific to each media collection. Each class will have an instance variable of type Sting called filename plus instance variables for the clip's metadata.
A collection of Interfaces that define Behavior Interfaces have many uses - (1) to prescribe certain behaviors, (2) to commonly identify all classes that implement an interface, (3) to act as a functional interface that can be passed as a parameter. In the next lesson, we will explore use #3. But we will use interfaces for the first two purposes in this assignment. You will be building an application that combines different media collections from old style TV shows. Each TV show's collection will only be of one specific file type. You will build a class specific to each media collection. Each class will have an instance variable of type Sting called filename plus instance variables for the clip's metadata.
Here are the clips you will build an instance of:
You will create all twelve instances of various clips and place them into an ArrayList. You will not use superclasses; rather you will use interfaces. The interfaces should define the play method according to the current types of media. At the moment, there are only collections of animated gifs and wav files, but you want to make sure that your ArrayList could cover other media forms, such as mp3 files or mp4 files in the future. Here is the code you will need to use to play wav files: System.out.println("Playing " + filename); AudioInputStream audioStream; try { Clip clip = AudioSystem.getClip(); clip.open(AudioSystem.getAudioInputStream(new File(filename))); clip.start(); Thread.sleep(clip.getMicrosecondLength() / 1000); clip.close(); } catch (Exception e) { e.printStackTrace(); } And here is the code you will need to play animated gifs (using Java Swing) System.out.println("Playing " + filename); JFrame frame = new JFrame(); ImageIcon icon = new ImageIcon(filename); JLabel label = new JLabel(icon); frame.add(label); frame.pack(); frame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE); frame.setLocation(filename.length()* 10, filename.length() * 10); frame.setVisible(true); Your driver will build the various twelve media clips in the table above, add them to an ArrayList, then iterate through the ArrayList and play each one. | |||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
Here are the clips you will build an instance of:
filename | Media Collection | Properties |
Dark-Jonas.gif | DarkClip | scene = Jonas Walking Into Cave |
Dark-TheStranger.gif | DarkClip | scene = Stranger Leaving Cave |
DarkShadows-Angelique.wav | DarkShadowsClip | speaker = Angelique, episode=606 |
DarkShadows-DoctorHoffman.wav | DarkShadowsClip | speaker = Doctor Hoffman, episode=613 |
DarkShadows-ProfessorStokes.wav | DarkShadowsClip | speaker = Professor Stokes, episode=506 |
DarkShadows-Zombie.wav | DarkShadowsClip | speaker = Zombie Sheriff Davenport, episode=942 |
GameOfThrones-Joffrey.gif | GameOfThronesClip | character = Joffrey Baratheon |
GameOfThrones-Jon.gif | GameOfThronesClip | character = Jon Snow |
RockyAndBullwinkle-Natasha.wav | RockyAndBullwinkleClip | speakers = [Natasha] |
RockyAndBullwinkle-RockyAndBullwinkle.wav | RockyAndBullwinkleClip | speakers = [Rocky, Bullwinkle] |
Seinfeld-Jerry.wav | SeinfeldClip | speaker = Jerry, year = 1997 |
Seinfeld-Kramer.wav | SeinfeldClip | speaker = Kramer, year = 1993 |
You will create all twelve instances of various clips and place them into an ArrayList. You will not use superclasses; rather you will use interfaces. The interfaces should define the play method according to the current types of media. At the moment, there are only collections of animated gifs and wav files, but you want to make sure that your ArrayList could cover other media forms, such as mp3 files or mp4 files in the future.
Here is the code you will need to use to play wav files:
System.out.println("Playing " + filename); AudioInputStream audioStream; try { Clip clip = AudioSystem.getClip(); clip.open(AudioSystem.getAudioInputStream(new File(filename))); clip.start(); Thread.sleep(clip.getMicrosecondLength() / 1000); clip.close(); } catch (Exception e) { e.printStackTrace(); }
And here is the code you will need to play animated gifs (using Java Swing)
System.out.println("Playing " + filename); JFrame frame = new JFrame(); ImageIcon icon = new ImageIcon(filename); JLabel label = new JLabel(icon); frame.add(label); frame.pack(); frame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE); frame.setLocation(filename.length()* 10, filename.length() * 10); frame.setVisible(true);
Your driver will build the various twelve media clips in the table above, add them to an ArrayList, then iterate through the ArrayList and play each one.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
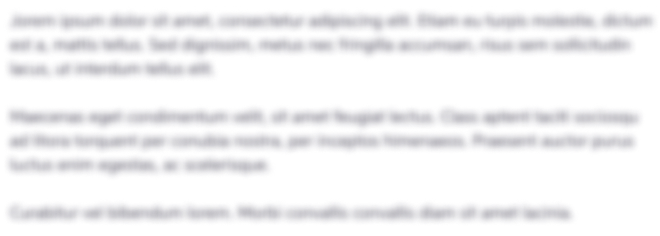
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started