Question
a.) Create the expression_tree class ( expressionTree.h ) according to the textbook requirements using a binary tree approach. Include a private data member and public
a.) Create the expression_tree class (expressionTree.h) according to the textbook requirements using a binary tree approach. Include a private data member and public accessor method (get_root) for the root pointer. The expression_tree class should hava default constructor to create an empty binary tree and a parmeterized constructor to create the binary expression tree form an input stream containing a valid postfix expression: expression_tree(istream&);
**********Textbook requirements*************
This project deals with a simple kind of expression tree, where there are two kinds of nodes:
(a) Leaf nodes, which contain a real number as their entry;
(b) Nonleaf nodes, which contain either the character + or the character * as their entry, and have exactly two children.
For this project, implement a class for expression trees, including operations for building expression trees. Also include a recursive function to evaluate a non-empty expression tree using these rules:
(a) If the tree has only one node (which must be a leaf), then the evaluation of the tree returns the real number that is the nodes entry;
(b) If the tree has more than one node, and the root contains +, then first evaluate the left subtree, then evaluate the right subtree, and add the results. If the root contains *, then evaluate the two subtrees and multiply the results.
For example, consider the small expression tree shown to the right. The left subtree evaluates to 3+7, which is 10. The right subtree evaluates to 14. So the entire tree evaluates to 10*14, which is 140.
***********End of Textbook Requirements*************
b) Each node of the binary expression_tree can contain either an integral numberic or character value in addition to the left and right subtree pointers. Use the following structure definition to represent this node:
struct exp_node {
int number;
char op;
exp_node* left_field;
exp_node* right_field;
};
c) Remember to properly allocate and release memory as needed to populate the nodes of hte binary expression_tree. Thus, an expression_tree destructor should release all memory that has been allocated.
d) Include a recursive function to evaluate and return the value of a binary expression_tree as described in the textbook.
e) Use expressionTree_test.cpp file to test our expression_tree
************expressionTree_tester.cpp*****************
#include "expression_tree.h" #include#include #include #include using namespace std; // displays binary expression tree void pretty_print(const exp_node* node_ptr, int depth = 5) // Library facilities used: iomanip, iostream { cout << setw(4 * depth) << ""; // indentation if (node_ptr == nullptr) { // fallen off the tree cout << "[Empty]" << std::endl; } else if (node_ptr->left_field == nullptr) { // a leaf with numeric data cout << node_ptr->number; cout << " [leaf]" << std::endl; } else { // a nonleaf with operator cout << node_ptr->op << std::endl; pretty_print(node_ptr->right_field, depth + 1); pretty_print(node_ptr->left_field, depth + 1); } } // main interactive testing function int main() { cout << "Enter the postfix expression: "; while (cin && cin.peek() != ' ') { // allocate memory expression_tree *expTree = new expression_tree(cin); // display binary tree pretty_print(expTree->get_root()); cout << " \t==> evaluates to " << evaluate(expTree->get_root()) << endl; // release memory delete expTree; // clear input stream cin.ignore(); cout << "Enter another postfix expression or to end: "; } cout << "This concludes the Binary Tree Math Postfix Expression program! "; return EXIT_SUCCESS; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
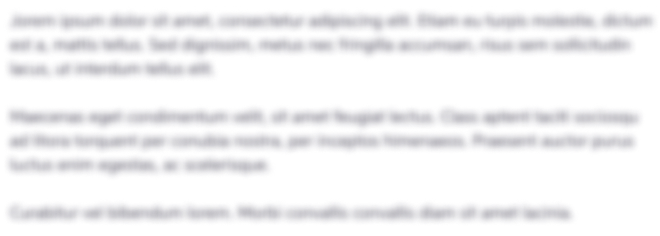
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started