Answered step by step
Verified Expert Solution
Question
1 Approved Answer
// A LinkedList object can be used to store a list of objects. public class LinkedList { private ListNode front; // node holding first value
// A LinkedList object can be used to store a list of objects. public class LinkedListO count Language/Type: Related Links: Author: Java generics implementing instance methods Linked List Linked List.java Marty Stepp (on 2012/02/29) Write a method count that accepts an element value as a parameter and returns the number of occurrences of that value in the list. For example, suppose a variable named list stores the following sequence of elements: [one, two, three, two, four, two, five, two, two, six] A call of list.count("two") should return 5 because there are five occurrences of that value in the list. If the list does not contain the value at all, return O. The example shown is a list of strings, but this is a generic linked list class that can store any type of objects. Assume that you are adding this method to the LinkedList{ private ListNode front; // node holding first value in list (null if empty) private String name = "front"; // string to print for front of list // Constructs an empty list. public LinkedList() { front = null; } // Constructs a list containing the given elements. // For quick initialization via Practice-It test cases. @SuppressWarnings("unchecked") public LinkedList(E... elements) { if (elements.length > 0) { front = new ListNode(elements[0]); ListNode current = front; for (int i = 1; i [" + current.data + "]"; if (current.cycle) { result += " (cycle!)"; cycle = true; break; } current = current.__gotoNext(); } if (!cycle) { result += " /"; } return result; } // Returns a text representation of the list. public String toString() { return toFormattedString(); } // ListNode is a class for storing a single node of a linked list. This // node class is for a list of values. // Most of the icky code is related to the task of figuring out // if the student has accidentally created a cycle by pointing a later part of the list back to an earlier part. private class ListNode { public E data; // data stored in this node public ListNode next; // link to next node in the list public boolean visited; // has this node been seen yet? public boolean cycle; // is there a cycle at this node? // post: constructs a node with data 0 and null link public ListNode() { this(null, null); } // post: constructs a node with given data and null link public ListNode(E data) { this(data, null); } // post: constructs a node with given data and given link public ListNode(E data, ListNode next) { ALL_NODES.add(this); this.data = data; this.next = next; this.visited = false; this.cycle = false; } public ListNode __gotoNext() { return __gotoNext(true); } public ListNode __gotoNext(boolean checkForCycle) { if (checkForCycle) { visited = true; if (next != null) { if (next.visited) { // throw new IllegalStateException("cycle detected in list"); next.cycle = true; } next.visited = true; } } return next; } } private final java.util.List ALL_NODES = new java.util.ArrayList (); public void clearCycleData() { for (ListNode node : ALL_NODES) { node.visited = false; node.cycle = false; } } // YOUR CODE GOES HERE }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
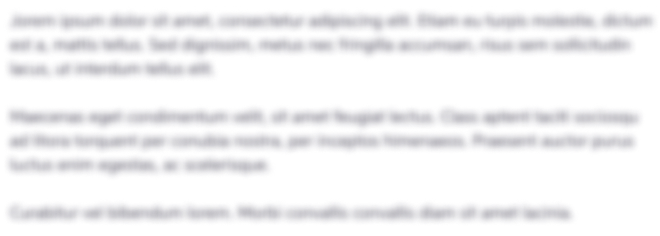
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started