Question
A new feature has been added to the Give Music platform, achievements . Only some users have achievements, and those that do are stored between
A new feature has been added to the Give Music platform, achievements. Only some users have achievements, and those that do are stored between the tags <achievements> and </achievements>. Each achievement stores a date and a name separated by a comma.
Here is an example section for testfile9_extra.txt:
2016-06-14,Classic's Fan 2016-11-16,Top Donor 2018-02-04,Top Donor 2019-05-02,Music Fan
If a file has an achievements block, insert a new achievements section into the program's report with a new a heading 'ACHIEVEMENTS' below the rest of the report. Print each achievement out in alphabetical order. If the user has gained the achievement more than once then add an asterisk and a count of the number of times it was earned to the end of that line. For example:
---------------------------------------- Achievement summary ---------------------------------------- Classic's Fan Music Fan Top Donor * 2 ----------------------------------------
The example above shows that the user has gained the following achievements: Classic's Fan, Music Fan a single time, and has earned the Top Donor achievement 2 times.
This extra functionality should be easy to add if you have refactored the initial program in a logical way such that each function does one thing effectively, and is then reused.
Notes:
- You can see examples of the new file format here.
- Your code still needs to work the same way as the original program if the achievement block is absent
- Whitespace at the start/end of achievement lines should be removed.
- This question is most easily done using dictionaries, but if you don't understand them yet you can do it without dictionaries. It's only worth 5%; don't stress if you can't do it.
For example:
Input | Result |
---|---|
testfile0_extra.txt | Enter a file name: testfile0_extra.txt ---------------------------------------- Donation Summary ---------------------------------------- User name: Coretta Timko Account Status: active ---------------------------------------- Calle 13 (59.00) Earth; Wind & Fire (86.00) Green Day (36.00) Kool and the Gang (31.25) Talking Heads (900.50) The Beatles (900.15) The Mamas and the Papas (62.50) The Mills Brothers (8.50) Toots and the Maytals (27.50) ---------------------------------------- Count: 9 Total: 2111.40 ---------------------------------------- Achievement summary ---------------------------------------- Classic's Fan ---------------------------------------- |
testfile1 testfile1.doc testfile.txt testfile1.txt | Enter a file name: testfile1 testfile1 DOES NOT EXIST! Enter a file name: testfile1.doc testfile1.doc DOES NOT EXIST! Enter a file name: testfile.txt testfile.txt DOES NOT EXIST! Enter a file name: testfile1.txt ---------------------------------------- Donation Summary ---------------------------------------- User name: Donette Sommers Account Status: suspended *** WARNING *** *** User can't access their account *** ---------------------------------------- Earth; Wind & Fire (77.00) Fleetwood Mac (99.15) Fugazi (72.50) Hsker D (38.25) Joy Division (88.50) Pavement (18.25) Pearl Jam (11.00) Puffy AmiYumi (92.15) Run-D.M.C. (64.50) The Bee Gees (300.50) The Clash (25.50) The Isley Brothers (26.25) The Mamas and the Papas (45.75) The Moody Blues (44.50) The Shadows (44.25) The Soul Stirrers (42.50) Yes (76.00) ---------------------------------------- Count: 17 Total: 1166.55 ---------------------------------------- |
testfile7_extra.txt | Enter a file name: testfile7_extra.txt ---------------------------------------- Donation Summary ---------------------------------------- User name: Nancie Borg Account Status: active ---------------------------------------- Fugazi (15.00) Jonas Brothers (84.00) Led Zeppelin (88.00) Smashing Pumpkins (98.00) Sonic Youth (76.15) The Beach Boys (78.15) The Four Tops (100.75) The Grateful Dead (40.75) The Hollies (60.50) The Moody Blues (32.00) The White Stripes (94.00) ---------------------------------------- Count: 11 Total: 767.30 ---------------------------------------- Achievement summary ---------------------------------------- Music Fan * 3 ---------------------------------------- |
HERE IS THE PROGRAM THAT NEEDS EDITING:-
"""h""" import os.path def tag_position(tag, lines): """k""" i = 0 found = False line = -1 while i < len(lines) and not found: line = lines[i] if line.strip().startswith(tag): line = i # line index found = True else: line = -1 i += 1 return line def tagged_contents(tag_name, lines): """g""" # pull out the eader data h_lines = {} start_tag = '<' + tag_name + '>'# Start tag end_tag = ''>' # End tag start_line = tag_position(start_tag, lines) end_line = tag_position(end_tag, lines) h_lines = lines[start_line + 1 : end_line] return h_lines def get_file_name(): """k""" filename = None while filename is None: filename = input("Enter a file name: ") if not os.path.isfile(filename): print(filename, "DOES NOT EXIST!") filename = None return filename def get_file_content(filename): """h""" file = open(filename, encoding="utf-8") lines = file.readlines() file.close() return lines def process_data(lines): """d""" h_lines = tagged_contents('head', lines) _, name = h_lines[0].strip().split(':') _, status = h_lines[1].strip().split(':') data_lines = tagged_contents('data', lines) data = [] for line in data_lines: _, band_name, amount = line.strip().split(',') amount = float(amount) data.append((band_name, amount)) return data, name, status def sum_donations(data): """g""" total = 0 for band_name, amount in data: total += amount return total def print_title(name, status): """g""" print("-" * 40) print("Donation Summary") print("-" * 40) print("User name: {}".format(name.title())) print("Account Status: {}".format(status)) if status in ["suspended", "deleted"]: print("*** WARNING ***") print("*** User can't access their account ***") print("-" * 40) def print_body(data, total): """g""" for band_name, amount in sorted(data): print("{} ({:.2f})".format(band_name, amount)) print("-" * 40) print("Count: {}".format(len(data))) print("Total: {:.2f}".format(total)) print("-" * 40) def main(): """k""" filename = get_file_name() lines = get_file_content(filename) data, name, status = process_data(lines) total = sum_donations(data) print_title(name, status) print_body(data, total) main()
THE TESTFILES ARE PROVIDED AS FOLLOWS:
testfile0_extra.txt
# mindless comment name:Coretta Timko account status:active 2016-01-19,The Mills Brothers,8.5 2016-06-13,The Mamas and the Papas,62.5 2016-10-15,Kool and the Gang,31.25 2016-12-29,Talking Heads,900.5 2017-08-11,The Beatles,900.15 2017-08-13,Calle 13,59.0 2018-08-27,Toots and the Maytals,27.5 2018-09-28,Earth; Wind & Fire,86.0 2019-03-28,Green Day,36.0
testfile1_extra.txt
name:Hermila Vento account status:suspended 2016-02-11,The Shirelles,28.25 2016-03-19,Rage Against the Machine,39.5 2016-07-31,The Mamas and the Papas,84.5 2016-09-17,The Four Seasons,88.5 2016-12-28,Coldplay,13.0 2016-12-29,Sam and Dave,85.0 2017-01-13,One Direction,92.25 2017-08-20,Dire Straits,93.25 2017-09-03,Run-D.M.C.,57.0 2017-10-08,The Flamingos,900.0 2018-01-11,The Police,54.15 2018-03-31,The Monkees,87.5 2018-05-19,Black Sabbath,67.0 2018-09-03,The Velvet Underground,100.25 2018-11-09,OutKast,15.15 2018-12-13,Red Hot Chili Peppers,17.0 2019-02-20,Calle 13,86.0Step by Step Solution
There are 3 Steps involved in it
Step: 1
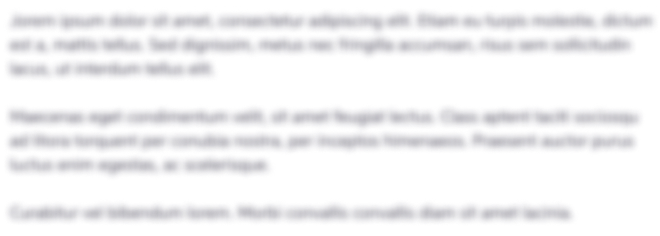
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started