Question
A playing card is a piece of specially prepared paper that is marked with distinguishing motifs. The 52-card deck is the most popular deck and
A playing card is a piece of specially prepared paper that is marked with distinguishing motifs. The 52-card deck is the most popular deck and includes 13 ranks of 4 suits. The ranks are Ace, 2, 3, 4, 5, 6, 7, 8, 9, 10, Jack, Queen, King. The suits are composed of clubs (), diamonds (), hearts () and spades (). An example of a 52 card deck is shown in Figure 3: Typical 52 Card Deck Suits and Ranks. For this problem, we will model a 52 card deck using three classes: clsCard to represent an individual card, clsCardDeck to represent a 52 card deck, and clsGame to manipulate a clsCardDeck.
1. Create a Java file called clsCard.java. Do not add a main() method to clsCard.java
2. Make comments
3. Create two instance variables of type String named strsuit and strvalue.
4. Create a constructor of clsCard with two parameters of type String named suit and value.
a. In the body of the constructor, use the this reference to assign the instance variables to the corresponding parameter values
b. Add a JavaDoc comment before the constructor signature with an explanation of your choice. The JavaDoc must be a complete sentence and explain what the method does. The parameters must be explained.
5. Create a method with the signature public String getSuit(). This method does not take any parameters and returns the suit as a String.
a. In the body of the method, return the instance variable strsuit.
b. Add a JavaDoc comment before the method signature with an explanation of your choice. The JavaDoc must be a complete sentence and explain what the method does. The return value must be explained.
6. Create a method with the signature public String getValue(). This method does not take any parameters and returns the value as a String.
a. In the body of the method, return the instance variable strvalue.
b. Add a JavaDoc comment before the method signature with an explanation of your choice. The JavaDoc must be a complete sentence and explain what the method does. The return value must be explained.
7. Create a method with the signature public String toString(). This method does not take any parameters and returns a String formatted as " of ".
a. In the body of the method, return a String consisting of the strvalue, concatenated with the string literal " of " concatenated with the strsuit.
b. Add a JavaDoc comment before the method signature with an explanation of your choice. The JavaDoc must be a complete sentence and explain what the method does. The return value must be explained.
8. Create a Java file called clsCardDeck.java in the same project directory as clsCard.java. Do not add a main() method to clsCardDeck.java.
9. Import the java.util.ArrayList library.
10. Make some comments
11. Create a constant String array named SUITS. Initialize the array with the values "Clubs", "Diamonds", "Hearts", "Spades".
12. Create an instance variable of an ArrayList of type clsCard named deck.
13. Create a constructor of the clsCardDeck with no parameters. a. In the body of the constructor, do the following: i. Use a nested loop structure to iterate over the suits and values. In the outer loop iterate over the integer values 0 to 3 inclusive. We will use these values as the positions in the SUITS array when we are instantiating the cards.
HINT: THE VALUES 0 TO 3 WILL BE USED TO INDEX THE SUITS ARRAY. 1. In the inner loop, iterate over the values 1 to 13 inclusive. We will use the iteration number as the value of the card. However, iteration 1 value is ace and iterations 11, 12, and 13 are jack, queen, king respectively.
a. In the body of the inner loop, we will use a nested decision structure to create a new clsCard object of the SUIT and value. HINT: EACH TIME YOU CREATE A CLSCARD, YOU NEED TO USE THE NEW KEYWORD. The decision structure should implement the following conditions:
b. If the inner loop iteration is 1, the value should be "Ace". Create a new object of type clsCard with SUIT indexed by the value of the outer loop iteration and value "Ace".
c. If the inner loop iteration is 2 to 10 inclusive, the value should be a string representation of the iteration number. Create a new object of type clsCard with SUIT indexed by the value of the outer loop iteration and use the .toString() method of the Integer class to convert the iteration number to a String representing the value.
d. If the inner loop iteration is 11, the value should be "Jack". Create a new object of type clsCard with SUIT indexed by the value of the outer loop iteration and value "Jack". e. If the inner loop iteration is 12, the value should be "Queen". Create a new object of type clsCard with SUIT indexed by the value of the outer loop iteration and value "Queen". f. If the inner loop iteration is 13, the value should be "King". Create a new object of type clsCard with SUIT indexed by the value of the outer loop iteration and value "King". b. Add a JavaDoc comment before the constructor signature with an explanation of your choice. The JavaDoc must be a complete sentence and explain what the method does.
14. Create a method with the signature public void shuffle(). The method does not take any parameters and does not return anything. The purpose of this method is the simulate a shuffle of a 52 card deck.
a. In the body of the method use a loop to iterate over the values from 0 to the size of the deck.
b. In the body of the loop, do the following:
i. Create a local variable of type int named intrandomPosition. Assign the integer casting of the product of a pseudo random number using the .random() method of the Math class multiplied by the size of the deck using the .size() method.
ii. Create a local variable of type clsCard named card and assign the result of calling the .remove() method with the parameter intrandomPosition on the object deck to card.
iii. Overwrite the value in intrandomPosition by assigning the integer casting of the product of a pseudo random number using the .random() method of the Math class multiplied by the size of the deck using the .size() method.
HINT: WE HAVE TO OVERWRITE INTRANDOMPOSITION TO CREATE A NEW PSEUDO RANDOM NUMBER. iv. Use the .add() method on the object deck with the parameters intrandomposition and card. HINT: WE ARE ADDING THE CARD BACK TO THE DECK IN A RANDOM POSITION.
c. Add a JavaDoc comment before the method signature with an explanation of your choice. The JavaDoc must be a complete sentence and explain what the method does.
15. Create a method with the signature public int deckSize(). The method does not take any parameters and returns a value of type int representing the number of cards in the deck object.
a. In the body of the method return the size of the deck object using the .size() method.
b. Add a JavaDoc comment before the method signature with an explanation of your choice. The JavaDoc must be a complete sentence and explain what the method does. The return value must be explained.
16. Create a method with the signature public String viewCard(int positionInDeck). The method takes one parameter of type int named positionInDeck and returns the String representation of a clsCards value and suit using the clsCards .toString() method. a. In the body of the method return the String representation of the clsCard object in the deck object using a nested function call composed of the .get() method of the deck object with parameter positionInDeck and the .toString() method of the clsCard class. b. Add a JavaDoc comment before the method signature with an explanation of your choice. The JavaDoc must be a complete sentence and explain what the method does. The parameter and return value must be explained.
17. Create a Java file called clsGame.java in the same project directory as clsCard.java and clsCardDeck.java. Add a main() method to clsGame.java.
18. Create a method after the main method with the signature public static void printDeck(clsCardDeck deck). The method takes 1 parameter of type clsCardDeck and does not return anything. The purpose of this method is to print the suit and value of each card in the deck.
a. In the body of the method, use a loop to iterate over each position in deck and print the cards value and suit using the the .viewCard() method on the deck object to the console.
19. In the main method, do the following:
a. Create a new object of type clsCardDeck named deck.
b. Print the string literal "Before shuffling: " to the console.
c. Use the printDeck() method with deck as the argument.
d. Print the string literal "After shuffling: " to the console.
e. Use the .shuffle() method on the deck object.
f. Use the printDeck() method with deck as the argument. The result of the deck after instantiation and after shuffling are shown in Figure 4: Example Output Before Shuffling Deck and Figure 5: Example Output After Shuffling Deck respectively.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
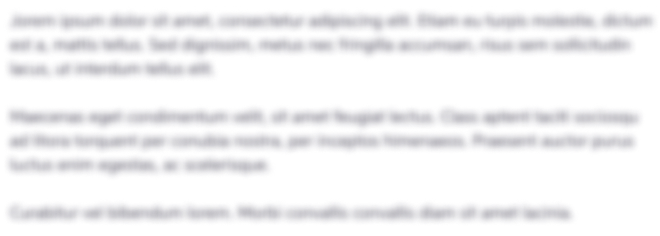
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started