Question
A poker hand consists of five cards from a standard 52-card deck (without jokers). The ranks, in order of increasing value, are 2, 3, 4,
A poker hand consists of five cards from a standard 52-card deck (without jokers). The ranks, in order of increasing value, are 2, 3, 4, 5, 6, 7, 8, 9, 10, Jack, Queen, King, and Ace, and the suits are Clubs (), Diamonds (), Hearts (), and Spades (). Each card has a rank and a suit, and all combinations are valid cards.
There are 9 classifications of hands, as follows, in order of decreasing value:
Straight flush
consists of 5 cards of the same suit whose ranks form a sequence, such as 7, 8, 9, 10, J. If two players both have a straight flush, the one with the highest card wins. If both players have the same ranks of cards (but different suits), the players draw. Suits are never used to decide the winner in poker.
Four of a kind
consists of a set of four cards of the same rank, and any other card, such as 3, 3, 3, 3, 7. If two players both have four of a kind, the one with the highest rank of their set of four wins. In some varieties of poker it is possible for two players to both have 4 of the same rank; in this case the one with the higher rank of their fifth card wins. If the fifth cards are also the same rank, it is a draw.
Full house
consists of three cards of one rank and two of another; that is three of a kind plus a pair, for example Q, Q, Q, 7, 7. If two player both have a full house, the one with the higher rank of their set of three wins. If two players to each have three cards of the same rank, then the rank of the pair is used to decide.
Flush
consists of any 5 cards of the same suit, regardless of their rank, for example K, 9, 3, J, 4. If two players both have a flush, then their highest ranking card is used to decide the winner. If their highest ranking cards have the same rank, then their second highest ranking cards, followed by their, third, fourth, and fifth highest ranking cards are used in turn.
Straight
consists of 5 cards whose ranks form a sequence, regardless of their suits, for example, 6, 7, 8, 9, 10. If two players both have a straight, the one with the highest ranking card wins. If two hands have the same highest ranking card, they draw.
Three of a kind
consists of 3 cards of the same rank, and any other 2 cards, for example, 6, 6, 6, 9, J. If two players both have three of a kind, the player whose three of a kind have the highest rank wins. If this is a tie, then the higher ranking other card is used, and if this, too, is a tie, then the rank of last card decides.
Two pair
consists of 2 cards of one rank, 2 cards of another rank, and any other card, such as 5, 5, 9, 9, A. If two players both have two pair, the ranks of their highest ranking pairs decide the winner; if their highest ranking pairs are the same rank, the second ranking pair decides; if they are also the same, the rank of the final cards decides.
One pair
consists of 2 cards of one rank, and any other 3 cards, for example, A, A, 6, J, 2. If two players both have one pair, the rank of the pair decides, with the ranks of their other cards, from high to low, used if their pairs are of the same rank.
High card
consists of any 5 cards. If two players both have a high card hand, the ranks of the cards, from high to low, are used to decide the winner.
For this project, you will write a program that will correctly characterise a poker hand according to the list of hands above. For students undertaking the more advanced project, if more than one hand is given as input, the program will also decide which hand wins.
In either case, your main program should be called Poker.java, and should expect some multiple of 5 cards on the command line (at least 5 cards). Cards should be entered on the command line as two-character strings, the first being an A for Ace, K for King, Q for Queen, J for Jack, T for Ten, or digit between 2 and 9 for ranks 29. The second character should be a C for Clubs (), D for Diamonds (), H for Hearts (), or S for Spades (). Your program should handle both upper and lower case characters (or even a mixture).
We make one small change to the rules of poker to make the project easier: we always take Aces to be the highest rank. Most versions of the rules of poker would consider Ace, 2, 3, 4, 5 to be a straight (or straight flush), but we do not.
The output generated by your program should include, for each hand specified on the command line, one line of the form:
Player_n:_description of hand
where n is 1 when describing the first 5 cards, 2 for the next 5, and so on, and space is shown as _. The description of hand gives both the hand category and some indication of how to decide ties using standard Poker lingo as follows:
In all of the above, ranks (r, r1 and r2 above) should be shown as numerals, or full capitalised rank names for face cards: 2, 3, 4, 5, 6, 7, 8, 9, 10, Jack, Queen, King, or Ace. Note that this is different from the way ranks are specified on the command line. Also note that the information conveyed in these hand descriptions is not always enough to decide the winner; for example, in deciding the winner between two Queen-high hands, the second-highest ranked cards in the two hands must be consulted, but these are not shown in the hand descriptions. Nevertheless, you must correctly decide the winner between two such hands, if you undertake the more challenging project.
For students choosing to complete the simpler version of the project, if more than 5 command line arguments are supplied, the program should output only NOT_UNDERTAKEN as a single line, and then exit. They will not be assessed on the tests for more than one hand; however, it is important that they output exactly the line NOT_UNDERTAKEN, so that the testing script does not take the output to be an erroneous attempt to handle the harder version of the project.
For students choosing to complete the more challenging assignment, if more than one hand is specified on the command line, the lines indicating each hands description must be followed by a single line sentence indicating the winner. When player number n wins, this should have the form Player_n_wins. When two players draw, the sentence should have the form Players_n1_and_n2_draw. where n1 and n2 are the player numbers of the tied winning players, in order of ascending player number. If three or more players draw, the sentence should have the form Players_n1,_n2_and_nk_draw. where n1nk are the winning player numbers in ascending order. In all of this, Player_1 corresponds to the first 5 cards, Player_2 to the next 5, and so on.
If the number of command line arguments is not greater than zero or not a multiple of five, your program should print out
Error:_wrong_number_of_arguments;_must_be_a_multiple_of_5
and exit. If any of the command line arguments is not a valid card name, as specified above, the program should print out
Error:_invalid_card_name_'c'
and exit, where c is the (first) invalid card entered on the command line.
Note well: Since some forms of poker allow the same card to be considered to be part of many players hands, you should not assume that no cards appear in more than one hand. If it is useful to you, you may assume that no cards are repeated in a single hand, but you do not need to check for this.
Examples
For example, this input:
java Poker 2H TH AS AD TC
should produce this output:
Player_1:_Aces_over_10s
This input:
java Poker 2H TH 1S 1D TC
Should produce this output:
Error:_invalid_card_name_'1S'Category Straight flush r-high straight_flushr is highest rank in hand Four of a kind Four rs Full house rs ful Flush Straight Three of a kindThree rs Two pair One paiir High card Description Detail r is rank of 4 cards is rank of 3 cards; r2 is rank of 2 r is highest rank in hand r is highest rank in hand r is rank of 3 cards ri is rank of higher pair; r2 is rank of l r is rank of pair r is highest rank in hand ris_full_of_r2s r-high flush r-high straight air; is_over_r2s Pair of rs r-high
Step by Step Solution
There are 3 Steps involved in it
Step: 1
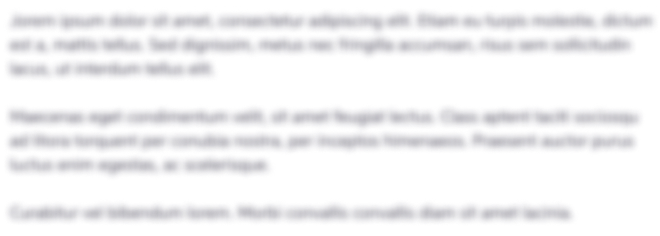
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started