Question
A Python Air (PA) Customer has several attributes associated to them, the most important is their customer identifier (a 6-digit integer) that provides us
"
A Python Air (PA) Customer has several attributes associated to them, the most important is their customer identifier (a 6-digit integer) that provides us with a means to uniquely identify them. Among other attributes, customers all have an _ff_status (frequent flyer status), _miles (PA's qualifying miles), and all_flight_costs.
-
_ff_status is a customer's frequent flyer status, which is an achievement of loyalty through taking trips with PA. The statuses are identified in a static variable coined FREQUENT_FLYER_STATUS in customer.py. Once a status is achieved, it is never lost.
-
_miles is a customer's qualifying miles on PA, which is the sum of all flight segments' length multiplied by their respective multiplier (i.e. see FREQUENT_FLYER_MULTIPLIER in customer.py for reference) that a particular customer has taken over the course of time. The number of miles will determine what _ff_status a customer is given (you may visit FREQUENT_FLYER_STATUS in customer.py for reference to this association). _miles are accumulated over the course of one's life, which means they are never lost. Note: even though the variable is called _miles, the variable's unit of measure is actually kilometres (so there is no need to convert between miles and kilomteres).
-
all_flight_costs is the total cost of all flight segments that a customer has taken. This is computed by multiplying the flight segment's base fare cost with their seat class (i.e. utilizing the CLASS_MULTIPLIER), as well as, finding and applying their discount (see FREQUENT_FLYER_STATUS in customer.py). The values of the tuples are (kilometres, percent), where a negative percent indicates a discount (e.g. a tuple (15000, -10) would be interpreted as 15,000KMs and -10% discount).
An Airport is a data structure which represents international airports, specifically their name, a unique IATA (International Air Transport Association) location identifier _airport_id, and a (longitude, latitude) tuple _map_location which stores that airport's geographical coordinate.
A FlightSegment is a PA flight that tracks everything from its departure airport location to its arrival airport location, the manifest, seat availability and capacity, base fare cost, etc. Some of this data requires computation through the use of private (helper) methods. Unlike airport and customer, the unique identifier of a FlightSegment is the combination of its flight id and departure time.
The flight id represents an alpha-numeric string uniquely distinguishing all flights that depart from the same location and arrive at the same location (i.e. IATA location identifiers).
Thus, the combination of both the flight identifier and time of departure will allow us to uniquely identify that flight. No two flights will have the same flight identifier and departure time.
A Trip is composed of several, or one, flight segments which makes up a customer's itinerary (i.e. the series of flights getting the customer from their original departure airport to their end arrival airport). Every trip has a unique identifier reservation_id which allows us to identify the customer customer_idwho made the booking, but it also stores an alias of every flight segment _flights that is part of this customer's itinerary. It should be noted that for a trip to be created, it must be composed of all the flight segments of that customer's itinerary. If one of the flight segments cannot be booked in any day within the timeframe of the dataset, then the whole trip does not get stored, as the trip cannot be booked.
A Filter represents a specific criterion to filter the flight segments so that the user will be able to visually see their final refined result. There are several types of filters, as described in the starter code.
The Visualizer is a class that does all the graphical work for this assignment. You do not need to code anything here, just know that it exists and is handling this for you.
Finally, we have the Application which does the data processing (reading, parsing, and cleaning), instantiation and storage of all PA's objects, and launches the graphical user interface.
Data
To aid in the rapid progression of software development, Python Air has provided you with both smalland normal data. The following data files have been given:
- airports.csv
- customers.csv
- segments.csv
- trips.csv
- segments_small.csv
- trips_small.csv
The small datasets include data from January 1st, 2019 through January 31st, 2019, while the regular sized versions include data from January 1st, 2019 through June 30th, 2019. Further, both airports.csv and customers.csv are files which are used when loading either the small or regular sized datasets.
NOTE: data is assumed to be correct and complete. This means that there will never be errors in the CSV files. CSV files will have exactly one blank line at the end of them.
airports.csv
This CSV-file contains the data of all international airports that Python Air has deals with. The data is provided in the following order: unique airport IATA location identifier, airport name, longitude, and latitude.
For example, let's take this airport:
YYZ,Lester B. Pearson International Airport,-79.63059998,43.67720032
the data can be parsed as follows:
airport_id = "YYZ" name = "Lester B. Pearson International Airport" map_location = (-79.63059998, 43.67720032)
customers.csv
This CSV-file contains the data of all customers that Python Air currently has stored in their company database. The data is provided in the following order: unique customer identifier, customer name, customer age, and customer nationality.
For example, let's take this customer:
605311,Minnie Van Ryder,65,German
the data can be parsed as follows:
customer_id = "605311" name = "Minnie Van Ryder" age = "65" nationality = "German"
segments.csv
This CSV-file contains the data of all flight segments that Python Air operated in 2019. The data is provided in the following order: flight identifier, departure IATA location identifier, arrival IATA location identifier, date of flight segment departure, time of flight segment departure, time of flight segment arrival, and flight segment length (in KMs).
For example, let's take this trip:
PA-001,YYZ,CDG,2019:01:01,12:59,22:54,9143
the data can be parsed as follows:
_flight_id = "PA-001" _dep_loc = "YYZ" _arr_loc = "CDG" _time = ("2019:01:01,12:59", "2019:01:01,22:54") # note that I have duplicated the date _flight_length = "9143"
trips.csv
This CSV-file contains the data of all customers' trips that Python Air operated in 2019. The data is provided in the following order: unique reservation identifier, unique customer identifier, date of departure, itinerary list.
For example, let's take this trip:
YKJRP,108620,2019-01-01,[('SVO','Economy'),('FCO','Economy'), ('YYZ','Economy'),('CAI','Business'),('HKG','Business'), ('DXB','')]
the data can be parsed as follows:
reservation_id = "YKJRP" customer_id = "108620" trip_departure = "2019-01-01" itinerary = "[('SVO','Economy'),('FCO','Economy'),('YYZ','Economy'),('CAI','Business'), ('HKG','Business'),('DXB','')]""
and, for clarity, the itinerary can be read as follows flight segments:
- SVO (Moscow) --> FCO (Rome) in Economy class
- FCO (Rome) --> YYZ (Toronto) in Economy class
- YYZ (Toronto) --> CAI (Cairo) in Economy class
- CAI (Cairo) --> HKG (Hong Kong) in Business class
- HKG (Hong Kong) --> DXB (Dubai) in Business class
Trips will always be well formed. This means a trip in the dataset will always have a departure and arrival location. You will never have partial/missing data.
NOTE: any sample parsing provided in the examples above, does not show the conversion into the correct types from the type contracts. It is solely showing you how the string maps to the variable of the associated class. The variable may need to be private!
Tasks
You are now ready to begin writing code! A note about doctests: we have omitted doctests from most of the starter code, and you are not required to write doctests for this assignment. Instead, you will write your tests in pytest because, in this assignment, initializing objects for testing is more involved than usual, resulting in messy docstrings. However, you will be required to follow all other aspects of the class design recipe.
Before starting to code, review your four datasets. I would suggest taking a piece of paper and recording the 'headers' of each of the data files. They are shown above. Additionally, if you are not familiar with the datetime module yet, read the datetime documentation.
Do not duplicate code! If you are given a method, use it! If you need to write a helper function because a piece of code is utilized over and over, do it. But only if it is necessary. Remember to make helpers private, and to follow data encapsulation principles.
Task 1: Complete the Initializers and Getters
Your first code-writing task is to complete the initializers (this includes initializing the specified default parameters) and getter methods only of the following classes (according to the docstring and type contract) in the order stated:
-
Airport: this means you will complete the entire class.
-
FlightSegment: ensure that you set any necessary attributes appropriately.
Note: The base fare cost of the flight segment is calculated at a DEFAULT_BASE_COST of $0.1225/km. The actual cost of a flight will change depending on the booking class (i.e. utilizing the CLASS_MULTIPLIER).
Hint: helper functions may come in handy here! "
from typing import Tuple class Airport: """ An ADT representing international airports and their locations on a map === Public Attributes === name: this is the name of the airport. >>> toronto = Airport("YYZ", "Lester B. Pearson International Airport", \ (-79.63059998, 43.67720032)) >>> toronto.get_airport_id() == "YYZ" True >>> toronto.get_location() == (-79.63059998, 43.67720032) True """ # === Private Attributes === # _airport_id: # this is a unique 3-character airport identifier (i.e. the IATA # location identifier; e.g. Toronto Pearson is "YYZ"). # # _map_location: # this is a tuple containing the longitude and latitude of the # the airport's coordinates on the world map. name: str _airport_id: str _map_location: Tuple[float, float] def __init__(self, aid: str, name: str, location: Tuple[float, float]) -> None: """ Initialize a new Airport object with the given parameters. """ # TODO def get_airport_id(self) -> str: """ Returns the unique IATA location identifier for this airport. """ # TODO def get_name(self) -> str: """ Returns the airport's name. """ # TODO def get_location(self) -> Tuple[float, float]: """ Returns the airport's location. """ # TODO
Step by Step Solution
There are 3 Steps involved in it
Step: 1
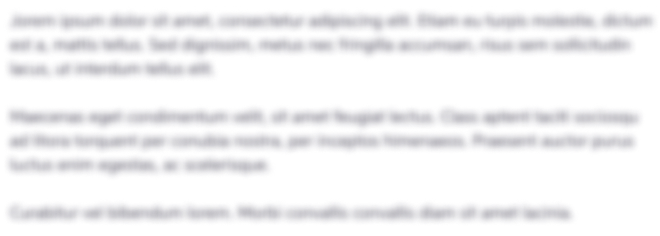
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started