Question
# A. reorder_strings. Given two strings, a and b, write a function that returns the result of putting them together in the order abba, e.g.
# A. reorder_strings. Given two strings, a and b, write a function that returns the
result of putting them together in the order abba, e.g. "Hi" and "Bye" returns
HiByeByeHi".
# remember to check the test cases below for examples
def reorder_strings(a, b):
""" This function must return a new string that is the result of concatenating
the arguments in the order abba.
Parameters:
a (str): A string of any composition.
b (str): A string of any composition.
Returns:
abba (str): A new string.
"""
abba = ""
return abba
# B. find_divisors. Given 4 integers, write a function that returns a string
holding all the numbers between start and end (inclusive of both), that are
divisible by one number and also multiples of another. Separate these numbers with
commas; it's fine that there's an extra comma at the end of the string.
def find_divisors(divisible_by, multiple_of, start, end):
""" This function must return a new string that is the result of concatenating
the arguments in the order abba.
Parameters:
divisible_by (int): A positive integer
multiple_of (int): A positive integer
start (int): A positive integer
end (int): A positive integer greater than start.
Returns:
all_nums (str): A new string.
"""
all_nums=""
return all_nums
# C. typeof_triangle. Given three values representing the lengths of the sides of a
triangle, determine whether the triangle is regular (all 3 sides equal), symmetric
(two sides equal), or irregular (no two sides are equal)
def typeof_triangle(x, y, z):
""" This function must return a string with the word "Regular", "Symmetric", or
"Irregular".
Parameters:
x (int): A positive integer.
y (int): A positive integer.
z (int): A positive integer.
Returns:
triangle_type (str): A new string.
"""
triangle_type = ""
return triangle_type
# D. shared_letters_check. Given two strings, determine which letters are present
at the same position in them, regardless of case.
def shared_letters_check(s1, s2):
""" This function must return a string with the shared letters, in the same order
as they were originally present in the string.
Parameters:
s1 (str): A non-empty string of letters.
s2 (str): A non-empty string of letters.
Returns:
s3 (str): An empty or non-empty string of letters.
"""
s3 = ""
return s3
# Provided simple test() function used in main() to print
# what each function returns vs. what it's supposed to return.
def test(got, expected):
if got == expected:
prefix = ' OK '
else:
prefix = ' X '
print('%s got: %s; expected: %s' % (prefix, repr(got), repr(expected)))
# Provided main() calls the above functions with interesting inputs,
# using test() to check if each result is correct or not.
def main():
print("reorder_strings test")
test(reorder_strings("First", "Second"), "FirstSecondSecondFirst")
test(reorder_strings("", ""), "")
test(reorder_strings("This", "That"), "ThisThatThatThis")
print("find_divisors test")
test(find_divisors(5, 10, 1, 100), "10,20,30,40,50,60,70,80,90,100,")
test(find_divisors(3, 7, 2001, 3001),
"2016,2037,2058,2079,2100,2121,2142,2163,2184,2205,2226,2247,2268,2289,2310,2331,23
52,2373,2394,2415,2436,2457,2478,2499,2520,2541,2562,2583,2604,2625,2646,2667,2688,
2709,2730,2751,2772,2793,2814,2835,2856,2877,2898,2919,2940,2961,2982,")
test(find_divisors(3, 5, 1, 100), "15,30,45,60,75,90,")
print("type of triangle test")
test(typeof_triangle(5, 6, 7), "Irregular")
test(typeof_triangle(1, 1, 1), "Regular")
test(typeof_triangle(7, 7, 5), "Symmetric")
print("check shared letters test")
test(shared_letters_check("Foo", "Bar"), "")
test(shared_letters_check("Foo", "far"), "f")
test(shared_letters_check("Fabulous", "fabtastiC"), "fab")
test(shared_letters_check("FUN", "funicular"), "fun")
test(shared_letters_check("oblivious", "Obviate"), "ob")
test(shared_letters_check("trauma", "polytrauma"), "")
# Standard boilerplate to call the main() function.
if __name__ == '__main__':
main()
answer a through d in python 3
Step by Step Solution
There are 3 Steps involved in it
Step: 1
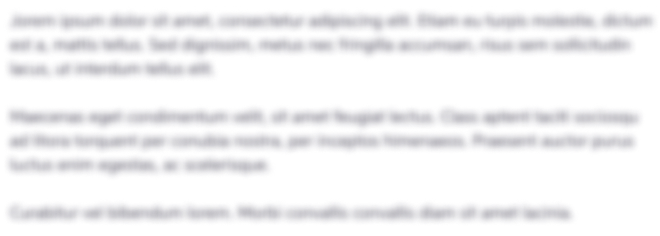
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started