Question
A standard deck of playing cards consists of 52 cards. Each card has a rank (2, 3, . . . , 9, 10, Jack, Queen,
A standard deck of playing cards consists of 52 cards. Each card has a rank (2, 3, . . . , 9, 10, Jack, Queen, King, or Ace) and a suit (spades, hearts, clubs, or diamonds).
You will create a class called Card that will simulate cards from a standard deck of cards. Your class will extend the AbstractCard class (provided).
The ordering of the cards in a standard deck (as defined for this assignment) is first specified by the suit and then by rank if the suits are the same. The suits and ranks are ordered as follows:
suits: The suits will be ordered diamonds < clubs < hearts < spades
ranks: The ranks will be ordered 2 < 3 < < 9 < 10 < Jack < Queen < King < Ace
A Joker card is a special card that is greater than any other card in the deck (any two jokers are equal to each other). A joker has no suit (None from AbstractCard.SUITS).
Again, the overall ordering for non-joker cards is specified by suit first and then rank; for example, all club cards are less than all heart cards. Two cards with the same rank and suit are considered equal.
Write a Java class called Card that extends the provided Card class. Your class must have two constructors:
public Card(String rank, String suit) // purpose: creates a card with given rank and suit // preconditions: suit must be a string found in Cards.SUITS // rank must be a string found in Cards.RANKS // Note: If the rank is AbstractCard.RANKS[15] then any
// valid suit from AbstractCard.SUITS can be given
// but the cards suit will be set to AbstractCard.SUITS[4]
public Card(int rank, String suit) // purpose: creates a card with the given rank and suit // preconditions: suit must be a string found in Cards.SUITS
// // // // Note: as with the other constructor, if a joker is created, any valid suit can be passed // but the cards suit will be set to AbstractCard.SUITS[4]
Note that the case of strings is important here. The input strings must be exactly the same as those found in AbstractCard.SUITS or AbstractCard.RANKS.
The specification for the three abstract methods in the AbstractCard class are given by:
public int getRank() // Purpose: Get the current cards rank as an integer // Output: the rank of the card
// joker -> 1, 2 -> 2, 3 -> 3, ..., 10 -> 10
// jack -> 11, queen -> 12, king -> 13, ace -> 14
public String getRankString() // Purpose: Get the current cards rank as a string // Returns the cardss rank as one of the strings in Card.RANKS
// (whichever corresponds to the card)
public String getSuit() // Purpose: Get the current cards suit // Returns the cards suit as one of the strings in Card.SUITS // (whichever corresponds to the card)
Do not change the abstract AbstractCard class. You can add any (non-static) attributes and helper methods that you need for your Card class.
ABSTRACT CLASS:
public abstract class AbstractCard implements Comparable
/** the numerical representation of the rank of the current card *
* ranks have the numerical values * 2 = 2, 3 = 3, ..., 10 = 10 * Jack = 11, Queen = 12, King = 13, Ace = 14 * Joker = 1 * @return the numerical rank of this card */ public abstract int getRank();
/** the string representation of the rank of the current card * * @return the string representation of the rank of this card * (must be a string from Card.RANKS) */ public abstract String getRankString();
/** the suit of the current card * * @return the suit of this card (must be a string from Card.SUITS) */ public abstract String getSuit(); @Override public final String toString(){ // outputs a string representation of a card object int r = getRank(); if( r >= 2 && r <= 14 ){ return r + getSuit().substring(0,1); }else if (r == 1){ return "J"; } return "invalid card"; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
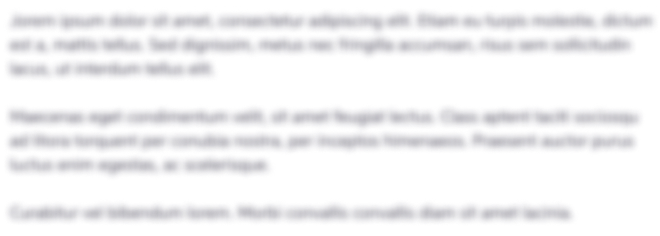
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started