Question
/** A Temperature object models temperature by storing its value (magnitude) * and scale. */ public class Temperature{ /** Initializes a Temperature object with given
/** A Temperature
object models temperature by storing its value (magnitude) * and scale. */ public class Temperature{
/** Initializes a Temperature
object with given value in Celsius *
* If the initial temperature is less than absolute zero (-273.15C) * then the temperature object will be initialized with -273.15C. * * @param value is the initial temperature in Celsius. */ public Temperature(double value){ }
/** Initializes a Temperature
object with given * value using the specified scale *
* If the temperature is lower than absolute zero, in the given scale, * then set the temperature to absolute zero (in that scale). *
* If the scale is not one of the three specified in the Scale class, * then create the object with value 0.0 and scale Scale.NONE
*
* Example: new Temperature(12.3, Scale.KELVIN)
will create * a Temperature
object with value 12.3 using the * Kelvin scale. * * @param value is the initial temperature * @param scale is the scale of initial temperature and must a constant * defined in the Scale enum type. */ public Temperature(double value, String scale){ }
/** A simple getter for the scale of this object. *
* The output will always be one of the static attributes * from the Scale
class. * * @return the current scale of this object. */ public String getScale(){ return Scale.Q; }
/** A simple getter for the value of this object. *
* The value will correspond to the current scale. That is, if the current * scale is Scale.CELSIUS
, then the value returned will be the * value in Celsius. * * @return the temperature of the object using the current scale */ public double getValue(){ return -Math.random(); }
/** A simple setter for the current scale of this object. *
* Changes the current scale of this object. Subsequent calls to * getValue()
and toString()
will output values * in this new scale. If the provided scale is not one of the three specified in the * Scale
class, then set the to scale Scale.NONE
* and leave the current value unchanged. *
* Example: Temperature t = new Temperature(0.0);
* t.setScale(Scale.FAHRENHEIT);
* A call to t.getValue()
should now return 32.0
. * * * @param scale is the new scale of the temperature and should be * one of Scale.CELSIUS
, Scale.FAHRENHEIT
, or * Scale.KELVIN
. If any other input is given then the the * behaviour of the method is described as above. */ public void setScale(String scale){ }
/** A simple setter for value of this object. *
* It is assumed that this value is in this object's current scale. *
* For example, if the current scale is Scale.KELVIN
, * then setValue(12.4)
sets current temperature to be 12.4K. *
* If the value specified is less than absolute zero, in the current scale, * the object's temperature is set to absolute zero in the current scale. * * @param value is the new value of the temperature. */ public void setValue(double value){ }
/** A setter for both the value and scale of this object. *
* If the temperature value is lower than absolute zero, in the given scale, * then sets the temperature to absolute zero (in that scale). *
* If the scale is not one of Scale.CELSIUS
, Scale.FAHRENHEIT
, * or Scale.KELVIN
, then set the object's value to be 0.0
and * scale to be Scale.NONE
. * * @param value is the new temperature value * @param scale is the new temperature scale. * It should be one of the three valid scales from the Scale
class */ public void setTemp(double value, String scale){ }
/* ------------------------------------------------- */ /* ------------------------------------------------- */ /* do not change anything below this */ /* ------------------------------------------------- */ /* ------------------------------------------------- */
/** Create a String representation of this temperature object. The value * is always given with three (3) decimal places (rounded). * * @return a String representation of this object. */ @Override public String toString(){ return "" + String.format("%.3f", this.getValue()) + this.getScale().charAt(0); }
}
Part One Temperature Complete the provided Temperature class. Add any attributes and helper methods as needed but keep in mind that testing will involve only the methods you are asked to write/complete. You must complete the constructors and methods in the provided class (without changing any signatures, return types, or modifiers). A lemperalure consists of a value (magnitude) and a scale. For example, if the temperature is 17.4C, then its value is 17.4 and its scale is Celsius. The valid scales that we will consider for our Temperature objects will be Celsius, Fahrenheit or Kelvin. Once a scale has been set, a Temperature object will always display its temperature in that scale until the scale is changed. The default scale is Celsius if not specified. In this problem you will need to be able to convert temperatures between Celsius, Fahrenheit and Kelvin. For help, see https://en.wikipedia.org/wiki/Conversion_of_units_of_temperature The three scales are represented by Strings in the provided Scale class (class attributes). For this assignment, the purpose of the Scale class is to provide a consistent naming scheme for the different scales. Essentially, we assign fixed names for the three scales and use these everywhere in the code (so that a simple spelling mistake in your code does not, result in failing every text, case). Some examples of using a Temperature object: Note: You must provide the state (instance atiributes) for the Temperature class. You must decide what state to store for this problem. But, you must only use instance attributes. You should have no static attributes in your class. Note: You must use information hiding in your class. Note: Temperature values are floating point numbers. If an object's value is expected to be 0.1 and your output (from getValue() is 0.09999999999998 ), that is OK. You are not asked to perform any rounding in the getValue() method. Note: The provided toString() method will always display your Temperature objects using three (3) decimal places. Rounding will happen automatically here in the String output. Note: A program called SimpleTemperatureProgram is provided with the code shown above that you can use as a starting point for your own testing if you wish. 1) You should have no static attributes in any of your classes. 2) Read the specifications in the skeleton files carefully if provided. 3) Be sure to use INFORMATION HIDING. Part One: Do not use Strings that look like the attributes from Scale. Use the actual static constants provided. ** The Scale class provides class attributes (constants) to be * used for temperature scales. This is used to ensure * with the scale names. */ ublic class Scale\{ public static final String CELSIUS = new String(original: "CELSIUS"); public static final String FAHRENHEIT = new String(original: "FAHRENHEIT"); public static final String KELVIN = new String(original: "KELVIN"); public static final String NONE = new String(original: "NONE"); // do not use the Q string! // it is here to simply have "some" scale provided // in the Temperature.java skeleton code so that it compile // without using any of the above strings public static final String Q= new String(original: "Q"); // this private constructor prevents the creation // of scale objects. There is no use for a Scale object. private Scale()\{\}Step by Step Solution
There are 3 Steps involved in it
Step: 1
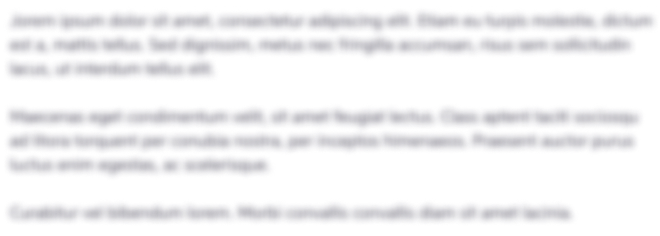
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started