Question
A thief robbing a store can carry a maximum weight of W in their knapsack. There are n items and ith item weighs wi and
A thief robbing a store can carry a maximum weight of W in their knapsack. There are n items and ith item weighs wi and is worth vi dollars. What items should the thief take to maximize the value of what is stolen?
The thief must adhere to the 0-1 binary rule which states that only whole items can be taken. The thief is not allowed to take a fraction of an item (such as of a necklace or of a diamond ring). The thief must decide to either take or leave each item.
Develop an algorithm using Java and developed in the Cloud9 environment (or your own Java IDE) environment to solve the knapsack problem.
Your algorithms should use the following data as input.
Maximum weight (W) that can be carried by the thief is 20 pounds
There are 16 items in the store that the thief can take (n = 16). Their values and corresponding weights are defined by the following two lists.
Item Values: 10, 5, 30, 8, 12, 30, 50, 10, 2, 10, 40, 80, 100, 25, 10, 5
Item Weights: 1, 4, 6, 2, 5, 10, 8, 3, 9, 1, 4, 2, 5, 8, 9, 1
Your solution should be based upon dynamic programming principles as opposed to brute force.
The brute force approach would be to look at every possible combination of items that is less than or equal to 20 pounds. We know that the brute force approach will need to consider every possible combination of items which is 2n items or 65536.
The optimal solution is one that is less than or equal to 20 pounds of weight and one that has the highest value. The following algorithm is a brute force solution to the knapsack problem. This approach would certainly work but would potentially be very expensive in terms of processing time because it requires 2n (65536) iterations
The following is a brute force algorithm for solving this problem. It is based upon the idea that if you view the 16 items as digits in a binary number that can either be 1 (selected) or 0 (not selected) than there are 65,536 possible combinations. The algorithm will count from 0 to 65,535, convert this number into a binary representation and every digit that has a 1 will be an item selected for the knapsack. Keep in mind that not ALL combinations will be valid because only those that meet the other rule of a maximum weight of 20 pounds can be considered. The algorithm will then look at each valid knapsack and select the one with the greatest value.
import java.lang.*; import java.io.*; public class Main { /** * @param args the command line arguments */ public static void main(String[] args) { int a, i, k, n, b, Capacity, tempWeight, tempValue, bestValue, bestWeight; int remainder, nDigits; int Weights[] = {1, 4, 6, 2, 5, 10, 8, 3, 9, 1, 4, 2, 5, 8, 9, 1}; int Values[] = { 10, 5, 30, 8, 12, 30, 50, 10, 2, 10, 40, 80, 100, 25, 10, 5 }; int A[]; A = new int[16]; Capacity = 20; // Max pounds that can be carried n = 16; // number of items in the store b=0; tempWeight = 0; tempValue = 0; bestWeight = 0; bestValue = 0; for ( i=0; i<65536; i++) { remainder = i; // Initialize array to all 0's for ( a=0; a<16; a++) { A[a] = 0; } // Populate binary representation of counter i //nDigits = Math.ceil(Math.log(i+0.0)); nDigits = 16; for ( a=0; a
Some of these algorithms may take a long time to execute. If you have access to a java compiler on your local computer or the Virtual Computing Lab, you may want to test your code by running it and executing it with java directly as it can speed up the process of getting to a result. You should still execute your code within Java to get an understanding of how it executes. (To compile with java use the javac command. To run a compiled class file, use the java command) Grading Rubric
Was a java algorithm solution for the knapsack problem provided
Is the code documented to give the reader an idea of what the author is trying to do within the code?
Does the java algorithm execute in the Java IDE environment
When executed does the algorithm produce the appropriate output as detailed above
Does the assignment include an asymptotic analysis describing the complexity of the algorithm in terms of Big-O (Big-, or Big- as appropriate)?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
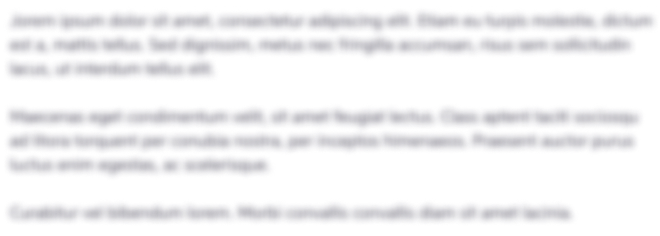
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started