Question
a. Using just the stub code for next() and __iter__() fooled most of our tests for iterable. Which test correctly reports that out Stack object
a. Using just the stub code for next() and __iter__() fooled most of our tests for iterable. Which test correctly reports that out Stack object is not yet Iterable?
i. Method that uses hasattr()
ii. Method that uses isinstance() and collections module
iii. Method that uses duck typing in a try/except/else block
b. After next() and __iter__() have been defined, your stacks should now be iterable. Hooray! Paste in your output for demo5() now that your stacks are iterable. Show the countdown to Blast-Off! No need to include the green duck.
The test object <__main__.stack object at> has type: Stack # Add the rest here
STEPS:
Start CODE:
__author__ = 'robincarr' import time import collections # added to test if a Stack is Iterable # Last item in the list named "items" is the TOP of the stack. # First item (index 0) in the list "items" is the bottom of the stack. # Top of the stack is the end of its list of items. # LIST IMPLEMENTATION OF A STACK IN PYTHON class Stack(object): def __init__(self, initial_list=[]): # example of an optional argument self.stack_list = initial_list # the list of items in the Stack def isEmpty(self): return self.stack_list == [] # Returns True if the stack is empty, otherwise False. def push(self, item): self.stack_list.append(item) # Append the new item to the top of the stack's list. def pop(self): # Implements the pop() method for stacks, using the pop() method for its list. if not self.isEmpty(): return self.stack_list.pop() def peek(self): return self.stack_list[len(self.stack_list) - 1] # These next methods are not core stack methods, but are still useful. def size(self): return len(self.stack_list) def __str__(self): return str(self.stack_list) + "# The arrow here represents the pointer to the top of the stack. def __eq__(self, other): # i. two stacks are equal iff their stack_list attributes are equal. Be sure to return the result. return self.stack_list == other.stack_list pass # Learn more about ANSI escape codes here: https://en.wikipedia.org/wiki/ANSI_escape_code # American National Standards Institute class ANSI: MAGENTA = '\033[95m'; CYAN = '\033[96m'; DARKCYAN = '\033[36m' BLUE = '\033[94m'; GREEN = '\033[92m' ; YELLOW = '\033[93m' RED = '\033[91m'; GREY = '\033[90m' BOLD = '\033[1m'; UNDERLINE = '\033[4m'; END = '\033[0m' def demo1(): print ANSI.BLUE + ANSI.BOLD + " * * * * * STACKS: DEMO # 1 * * * * * " + ANSI.END stack1 = Stack([1, 2, 3, [4, 5]]) print "Here is out initial stack", stack1 print "Next, we will pop it, until it is empty. " while not stack1.isEmpty(): stack1.pop() print "\tAfter popping: ", stack1 print "It's OK to pop an empty stack. We added code so that does nothing and does not throw an error either." stack1.pop() # no problem, does not throw error. def demo2(): print ANSI.BLUE + ANSI.BOLD + " * * * * * STACKS: DEMO # 2: PALINDROME * * * * * " + ANSI.END stack1 = Stack([]); stack2 = Stack([]); temp = Stack([]) # a temporary stack, soon to be consumed. test_phrase = "TacoCat".upper() test_phrase = "Aerate pet area.".upper() # ii. Add a for loop and an if statement to push each character in the test_phrase onto both stack1 and temp, # if that character (ch) isalpha(). for ch in test_phrase: if ch.isalpha(): stack1.push(ch); temp.push(ch) # iii. pop every item from temp and push it onto stack2. This will create a reversed stack. while not temp.isEmpty(): stack2.push(temp.pop()) # iv. test if stack1 and stack2 are equal using ==. Print an appropriate message. # Must fix the dunder method __eq__ first! # Use an if/else structure to print yes or no messages that also mprint out the test_phrase. if stack1 == stack2: print ANSI.GREEN + "Yes, %s is a palindrome!" % test_phrase + ANSI.END else: print ANSI.RED + "No, %s is NOT a palindrome!" % test_phrase + ANSI.END def demo3(): """Demo3 shows a stack object is not Iterable using the hasattr method""" print ANSI.BLUE + ANSI.BOLD + " * * * * * STACKS: DEMO # 3: Iterable Test using hasattr() method * * * * * " + ANSI.END # First let's build a stack that can be used to countdown to zero and launch a rocket. my_list = ["Blast-Off!!"] + range(0, 11) + ["Commencing countdown!"] # list with items needed for the countdown my_stack = Stack() # an empty stack for item in my_list: my_stack.push(item) # push all the items onto the Stack result = hasattr(my_stack, "__iter__") if result: print ANSI.GREEN + "Yes, the object %s of type '%s' IS iterable!" % (my_stack, type(my_stack).__name__) + ANSI.END else: print ANSI.RED + "Sorry, the object %s of type '%s' is NOT iterable!" % (my_stack, type(my_stack).__name__) + ANSI.END while not my_stack.isEmpty(): print my_stack.pop(), time.sleep(0.1) # time accelerated 10X so we don't get bored print "" def demo4(): """Demo4: Show a stack object is not Iterable using isinstance and the collections module""" # Add ANSI tags so this header prints in blue and bold. print " * * * * * STACKS: DEMO # 4: Iterable Test using isinstance & collections module * * * * * " # First let's build a stack that can be used to countdown to zero and launch a rocket. my_list = ["Blast-Off!!"] + range(0, 11) + ["Commencing countdown!"] # list with items needed for the countdown my_stack = Stack() # an empty stack for item in my_list: my_stack.push(item) # push all the items onto the Stack # Add code here to test is my_stack is iterable using the collections module. Use an if/else block # so your code does not break when we make our stack class iterable # print yes answers in green, no answers in red. while not my_stack.isEmpty(): print my_stack.pop(), time.sleep(0.1) # time accelerated 10X so we don't get bored print "" # It's not yet iterable, so this attempt to use a for loop fails! try: for item in my_stack: print item except TypeError as e: print "Sorry, you cannot use a for loop! That's why we used a while loop to pop the stack." def show_duck(): # adjust code so the duck prints to the console in green. # just prepend ANSI.GREEN to the duck and postpend ANSI.END inside the print statement duck = """ ,;MMMM.. ,;:MM"MMMMM. ,;.MM::M.MMMMMM: ""::.;'MMMMMMMMM "'""MMMMM; ':MMMM. 'MMMM; :MMMM;. MMMMMM;... MMMMMMMMMMMMM;.;.. MMMMMMMMMMMMMMMMMMM... MMMMMM:MMMMMMMMMMMMMMM;... ..: MMMMMM;MMMMMMMMMMMMM:MMMMMMM:MMMM:M :MMMMMM:MMMMMMMMMMMMMMM.:::;:::;;:' ':MMMMMMM:MMMM:;MM:M;.MMM:';::M:' ':MMMMMM;M;;MM;::M;;::::;MM:"" 'MMMMMMMM;M;:::MMMMMMMMMM" ''MMMMMMMMMMMMMMMMMMMMM" ':MMMMMMMMMMMMMMMM"' '':MMMMMMMMMMM"' -hrr- ':MMMMMM""' . : :: ,..;.M' ,;;MM:' '"' """ # Add ANSI tags so this duck prints in green. print duck def demo5(): """Demo5: Show a stack object is not Iterable using duck typing in a try/except/else block""" print " * * * * * STACKS: DEMO # 5: Iterable Test using DUCK TYPING * * * * * " show_duck() # First let's build a stack that can be used to countdown to zero and launch a rocket. my_list = ["Blast-Off!!"] + range(0, 11) + ["Commencing countdown!"] # list with items needed for the countdown my_stack = Stack() # an empty stack for item in my_list: my_stack.push(item) # push all the items onto the Stack # Add code here to test is my_stack is iterable using duck typing in a try/except block # also use a for loop to step through the Stack if it passes the Iterable test print "The test object %s has type: %s" % (repr(my_stack), type(my_stack).__name__) try: iterator = iter(my_stack) # duck-type test? Can it quack?? (i.e. call __iter__) except TypeError as e: pass # add code here else: print ANSI.GREEN + "Yes, the object %s of type '%s' IS iterable!" % (my_stack, type(my_stack).__name__) + ANSI.END print "Let's iterate through its items in a for loop." # add for loop here if __name__ == "__main__": demo1() demo2() demo3() demo4() demo5()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
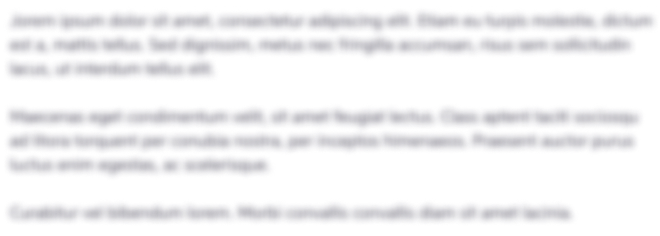
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started