Question
a) Write a C++ class called Clock for dealing with the daytime represented by hours, minutes, and seconds. Your class must have the following features:
a) Write a C++ class called Clock for dealing with the daytime represented by hours, minutes, and seconds. Your class must have the following features: 1. Three instance variables for the hours (range 0 - 23), minutes (range 0 - 59), and seconds (range 0 - 59). 2. Two constructors: i. default (with no parameters passed; should initialize the represented time to 12:0:0) ii. a constructor with three parameters: hours, minutes, and seconds. 3. Instance functions: i. Functions getHours(), getMinutes(), getSeconds() with no parameters that return the corresponding values. ii. Functions setHours(), setMinutes(), setSeconds() with one parameter each that set up the corresponding instance variables. iii. Function tick() with no parameters that increments the time stored in a Clock object by one second. iv. A function printClock() that would print the time stored a Clock object using the standard time. For example, if a clock object time has an hours = 18, minutes = 35, seconds = 50 then this function should print the following: Time: 06:35:50 PM v. Function addClocks() that would accept two objects of type Clock as parameters. This function should return a new created Clock object that has the result of the addition of the two times in these two received parameters. 2 b) Write a C++ main ( ) function that should do the following: 1. Create a Clock object firstClock using the default constructor defined above. 2. Print the time in firstClock using the function printClock(). 3. Tick the clock in the firstClock object ten times by applying its tick() function and printout the time after each tick using the function printClock(). 4. Create another Clock object secondClock using the second constructor defined above with the three integers (hours, minutes, seconds) that should be read from the keyboard. 5. Print the time in secondClock object using the function printClock(). 6. Tick the clock of the secondClock object ten times, printing the time after each tick using the function printClock(). 7. Call the function addClocks() to add the time in the firstClock object to the time in the secondClock object. Name the return object from this function call thirdClock. Then printout the time in thirdClock object using the function printClock(). 8. Change the time in the firstClock object to be (hours = 7, minutes = 23, seconds = 15) using the set functions defined above, then printout the time in this object. 9. Check if the two time objects firstClock and secondClock have the same time using the get functions defined above to check if they have the same hours, same minutes, and the same seconds. If so, printout the following message: firstClock and secondClock have the same time Otherwise, printout the following message: firstClock and secondClock do not have the same time 3 Sample Run: Creating firstClock object using the default constructor Time: 12:00:00 AM Ticking firstClock object Tick 1: Time: 12:00:01 AM Tick 2: Time: 12:00:02 AM Tick 3: Time: 12:00:03 AM Tick 4: Time: 12:00:04 AM Tick 5: Time: 12:00:05 AM Tick 6: Time: 12:00:06 AM Tick 7: Time: 12:00:07 AM Tick 8: Time: 12:00:08 AM Tick 9: Time: 12:00:09 AM Tick 10: Time: 12:00:10 AM Creating secondClock object using the second constructor Enter Value for hour: 15 Enter value for minutes: 59 Enter value for seconds: 57 Time: 03:59:57 PM Ticking secondClock object Tick 1: Time: 03:59:58 PM Tick 2: Time: 03:59:59 PM Tick 3: Time: 04:00:00 PM Tick 4: Time: 04:00:01 PM Tick 5: Time: 04:00:02 PM Tick 6: Time: 04:00:03 PM Tick 7: Time: 04:00:04 PM Input these values from the keyboard 4 Tick 8: Time: 04:00:05 PM Tick 9: Time: 04:00:06 PM Tick 10: Time: 04:00:07 PM Adding the time in the firstClock object to the time in the secondClock object to produce the thirdClock object: Time in thirdClock is: Time: 04:00:17 AM Changing the time in firstClock object to be: Time: 07:23:15 AM firstClock and secondClock do not have the same time ----------------------------------------------------------------------------------------------------- Notice: if firstClock object has the following time values: hours = 12 minutes = 0 seconds = 10 and the secondClock object has the following time values: hours = 16 minutes = 0 seconds = 7 adding the times in these two objects to produce a new Clock object with time values as follows: hours = 12 + 16 = 28 % 24 = 4 minutes = 0 + 0 = 0 % 60 = 0 seconds = 10 + 7 = 17 % 60 = 17 The following is not part of the output This is to explain how the addition of two times objects is done
Step by Step Solution
There are 3 Steps involved in it
Step: 1
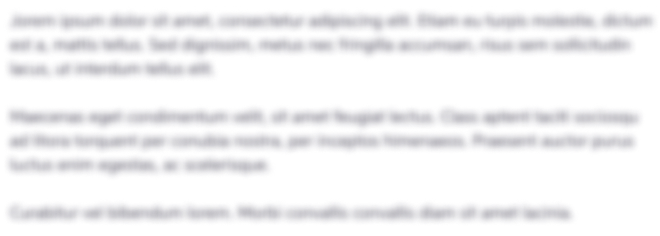
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started