Question
A. Write a PHP function 'bassAckwards' that will take a comma delimited string such as: Php,Cookies,Arrays,Mysql,LAMP And will return a string with the individual elements
A.
Write a PHP function 'bassAckwards' that will take a comma delimited string such as: Php,Cookies,Arrays,Mysql,LAMP
And will return a string with the individual elements reversed, but in the same order: phP,seikooC,syarrA,lqsyM,PMAL
B.
Write a PHP function 'getTLD' that accepts a domain name (such as www.google.com) as a single string argument and returns the top-level domain, the right-most portion, of that domain (com).
Note that fully qualified domains actually end in a period (www.google.com.). It is optional when displayed and often not included. Your fuction, however, should account for this as shown in the third example.
getTLD("iastate.edu"); // returns "edu" getTLD("www.bob.smith.info"); // returns "info" (even though it is not just three characters) getTLD("w3.org."); // returns "org" (without the trailing period)
C.
Given a birthdate in the form mm/dd/yyyy, write a function that returns the person's age and true or false if today is the person's birthday.
For example, since today is 04/19/2017, your function would return the following:
getBirthDate('05/22/1977'); // returns array('age' => 39, 'birthday' => false); getBirthDate('04/19/1996'); // returns array('age' => 21, 'birthday' => true);
D.
Write a function called bubbleSort which takes an array of numbers and returns an array with the numbers sorted from lowest to highest.
You should implement this function without using any of PHP's built-in sort functions. Check out Wikipedia's "Bubble Sort" page for more information on the algorithm. You may use any sorting algorithm you choose, it doesn't have to be Bubble Sort, though the function name must still be called bubbleSort for you to submit your code.
E.
Given the following MySQL database table.
+------------------------------------------------------------+ | interview_student | +------------+-----------+------------+---------+------+-----+ | Student_ID | Last_Name | First_Name | College | GPA | ... | +------------+-----------+------------+---------+------+-----+ | 463127376 | Willemsen | Lucas | A | 2.37 | ... | | 338288531 | Hansen | Jocelyn | D | 2.56 | ... | | 357921012 | Looysen | William | E | 3.90 | ... | | 265307238 | Hester | Susan | E | 1.25 | ... | | 152900535 | Gaddis | Robert | S | 2.45 | ... | +------------+-----------+------------+---------+------+-----+
Construct a SELECT statement that returns: Student_ID, Last_Name, First_Name, GPA for all of the students with a GPA of $min or better. Where $min will be the argument passed to your function.
Write a function 'getStudent' which takes one argument ($min) and returns the query string.
The function need only return a string which contains the MySQL statement. You do not need to handle the connection, submitting the query, parsing the results, etc. Just return a string that contains the MySQL statement.
Be sure the columns are returned in the specified order: Student_ID, Last_Name, First_Name, GPA
function getStudent($min) { // replace the query statement below with the correct statement $query = "SELECT * FROM interview_student"; return $query; } ?>
F.
Assuming access to the interview_student table from the previous question...
+------------------------------------------------------+ | interview_student | +------------+-----------+------------+---------+------+ | Student_ID | Last_Name | First_Name | College | GPA | +------------+-----------+------------+---------+------+ | 463127376 | Willemsen | Lucas | A | 2.37 | +------------+-----------+------------+---------+------+
The accounting office has provided us a list of students who currently have holds on their accounts because of unpaid tuition. They are only listed by ID and amount due; and they are only listed if they have an amount due. Students who have no amount due are not listed.
+-------------------------+ | interview_account_hold | +-------------------------+ | Student_ID | Amount_Due | +------------+------------+ | 416996983 | 3,403.20 | | 287856417 | 2,318.13 | | 537713123 | 3,678.06 | | 187296716 | 2,202.83 | | 145768187 | 2,379.32 | +------------+------------+
Write a function called hasHold that takes one argument ($college) and returns a string (a SELECT statement).
The argument passed to the function will be a college code (such as 'A' for Agriculture).
The string returned should be a SELECT statement that will return all the students who are in the given college that have an account hold in place. The resulting query should return the following fields in the following order: Student ID, Last Name, First Name, College, Amount Due.
Once again, the function should return the SELECT statement as a string. You do not need to handle the actual connection, submitting the query, parsing the results, etc. Just return a string.
return $query; } ?>
Write a PHP function "checkPass" that takes two arguments: $user and $password and returns the UserID if the password matches the MD5 encoding of the appropriate password in the interview_user_info table below and -1 otherwise.
The $user variable contains the NetID of the individual; the $password variable contains a clear-text version of the password as submitted.
+---------------------------------------------------------------------------------+ | interview_user_info | +-----------+-------------+-----------+--------+----------------------------------+ | UserID | LastName | FirstName | NetID | Passwd | +-----------+-------------+-----------+--------+----------------------------------+ | 469562029 | Gonzalez | Shauntee | sgonz | 530b4a0ae65148d112537bc0eafba9c9 | | 702930431 | Bates | Austin | abates | 9cdfb439c7876e703e307864c9167a15 | +-----------+-------------+-----------+--------+----------------------------------+
The connection and database selection are taken care of. Write the query to pull data from the table "interview_user_info", and return the result. The connection will be closed for you.
Hint: You will likely need to make use of the following functions and possibly others: md5, mysql_query, mysql_fetch_assoc
function checkPass($user, $password) { // You will likely want to use mysql_query($query) AND mysql_fetch_assoc($result); // YOUR CODE HERE
return $UserID;
} ?>
Step by Step Solution
There are 3 Steps involved in it
Step: 1
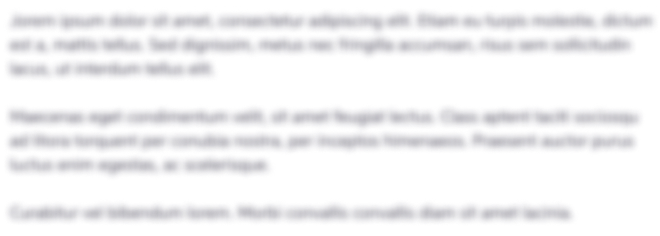
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started