Question
a. Write an abstract class Animal that represents the concept of a generic animal. Your class must meet the following requirements. The class must have
a. Write an abstract class Animal that represents the concept of a generic animal. Your class must meet the following requirements.
The class must have the private field String name, which should be used to hold the name (not type) of the animal (e.g. Fido).
The class must have a constructor Animal(String aName) that initializes the value of name.
The class must have a public abstract method void makeNoise() that is declared without implementation (since we dont know what kind of noise a generic animal makes).
The class must have a public getter function String getName() that returns name.
The class must provide an override to the method public String toString(), which returns a string that reads [name], who is a generic Animal where [name] should be replaced by name.
b. Write two classes Dog and Cat, which should both be declared to be subclasses of Animal and satisfy the following requirements.
Both classes must have constructors that call their superclass constructor in order to set the name of the animal that is being create (whether a dog or a cat).
Both classes must provide implementations of the makeNoise() method declared in Animal. The implementation for the Dog class should print out Woof Woof! and the implementation for the Cat class should print out Meow!
The Dog class should introduce a new method public void playCatch() that prints out the statement Oh Boy, I love playing fetch!
Both classes should override the public String toString() method so that they return the strings [name], who is dog and [name], who is a cat respectively.
c. Write a class Chihuahua that is a subclass of Dog. This class should satisfy the following requirement.
The class should have a constructor that calls its superclass constructor (which in turn calls the superclass superclass constructor) in order to set the value of name.
The class should override the method makeNoise() so that it prints out Squeak! Squeak!
d. Use your classes above to write a sample program to help evaluate the output of the following instructions. Please keep in mind that some of these statements will lead to compile and/or runtime errors.
i. Dog A = new Dog(Fido); System.out.println(A);
ii. Animal B = new Cat(Garfield); B.makeNoise();
iii. Animal C = new Animal(Tweetie); System.out.println(C);
iv. Animal D = new Animal(Leo) {
@Override public void makeNoise() {
System.out.println(Roar!);
}
}; D.makeNoise();
v. Animal E = new Dog(Rover); E.playFetch();
vi. Animal F = new Dog(Lady); ((Dog) F).playFetch();
vii. Animal G = new Cat(Heathcliff); ((Dog) G).playFetch();
viii. Dog H = new Chihuahua(Tiny); H.makeNoise();
ix. Animal I = new Chihuaua(Tinier); ((Dog) I).makeNoise();
e. Based on this exercise, given a reference type that does not match the constructed object type (i.e., the reference class is a superclass of the class to which the object belongs), describe in your own words how can one determine if a class method call is legal, and assuming that it is, which implementation will be used
Step by Step Solution
There are 3 Steps involved in it
Step: 1
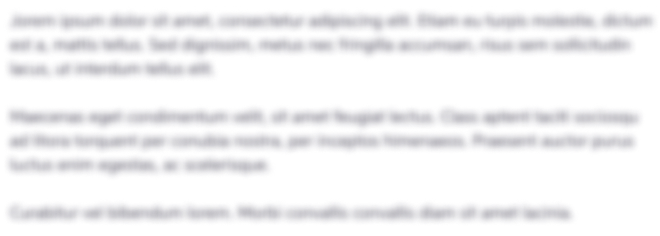
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started