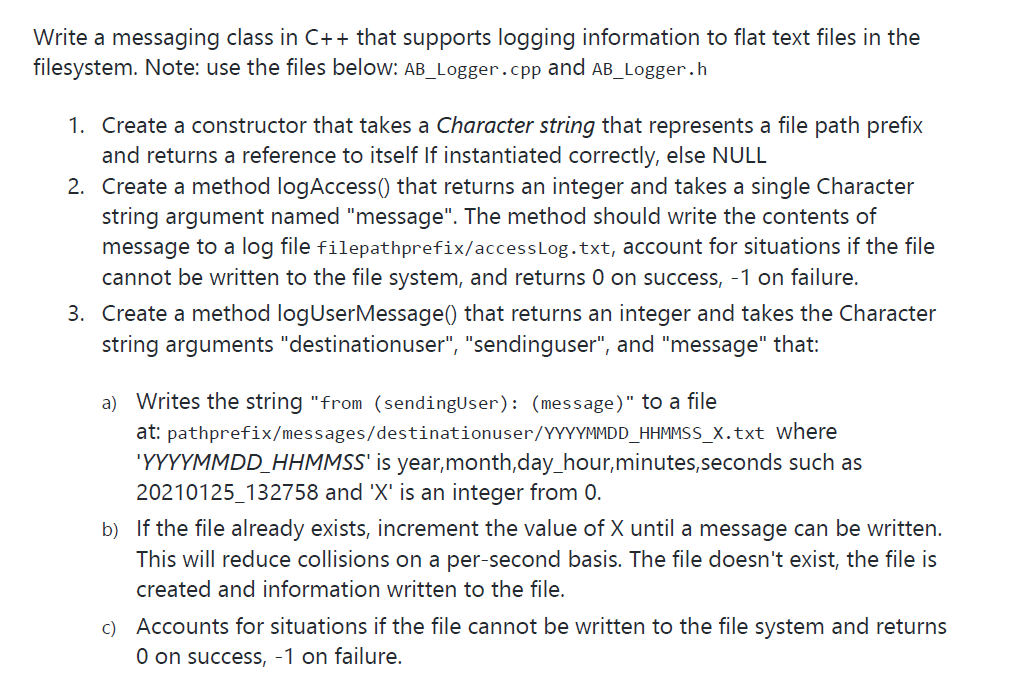
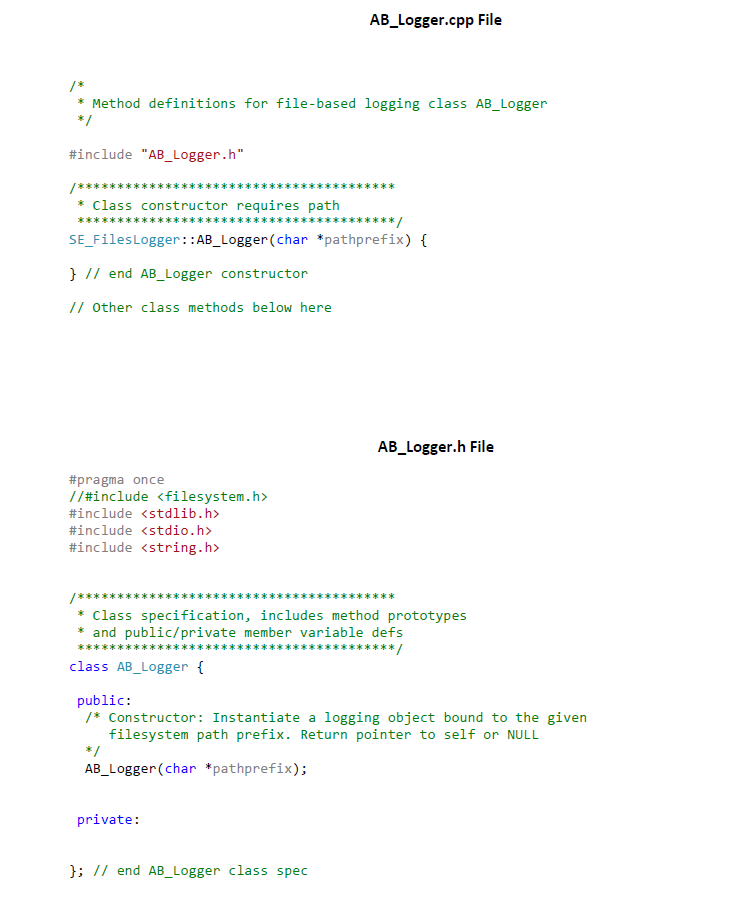
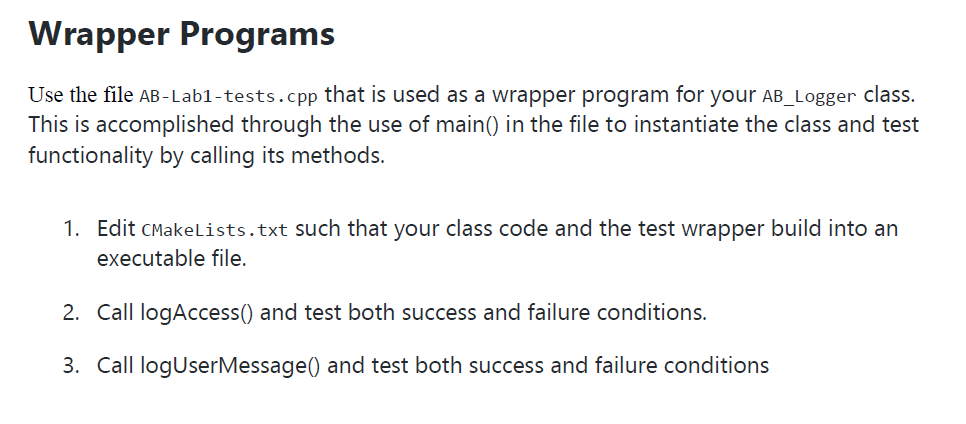
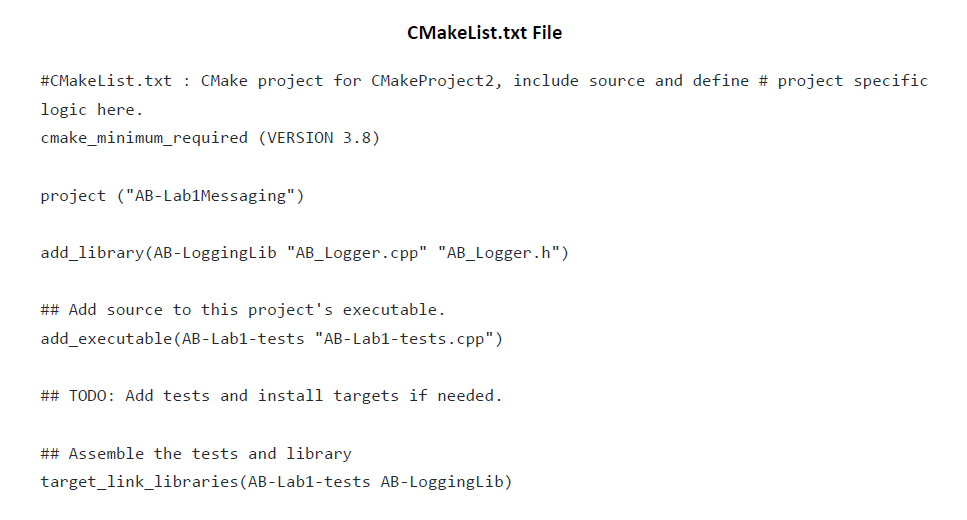
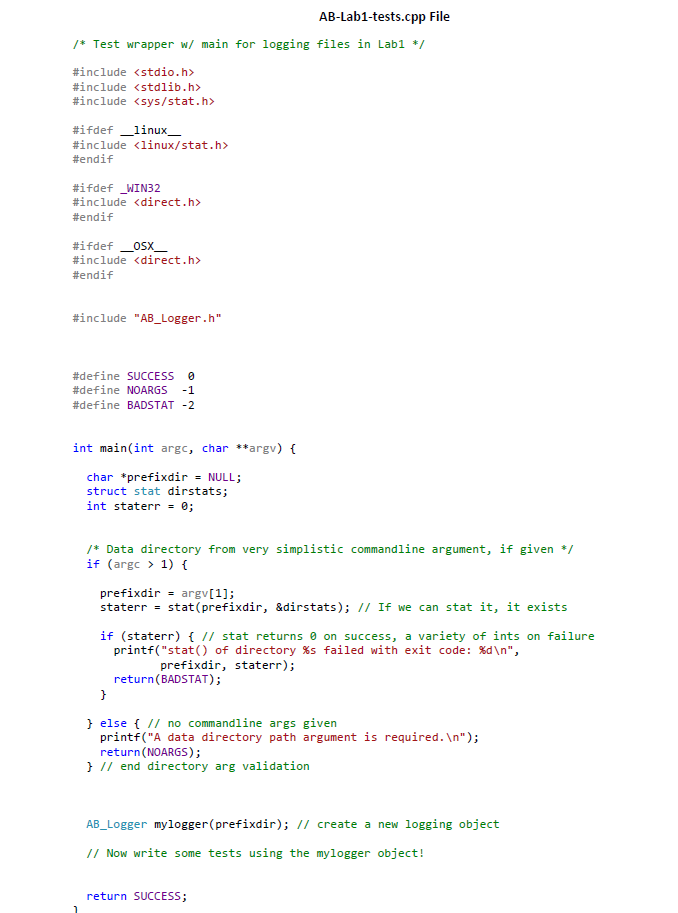
AB-Lab1-tests.cpp File
/* Test wrapper w/ main for logging files in Lab1 */
#include
#include
#include
#ifdef __linux__
#include
#endif
#ifdef _WIN32
#include
#endif
#ifdef __OSX__
#include
#endif
#include "AB_Logger.h"
#define SUCCESS 0
#define NOARGS -1
#define BADSTAT -2
int main(int argc, char **argv) {
char *prefixdir = NULL;
struct stat dirstats;
int staterr = 0;
/* Data directory from very simplistic commandline argument, if given */
if (argc > 1) {
prefixdir = argv[1];
staterr = stat(prefixdir, &dirstats); // If we can stat it, it exists
if (staterr) { // stat returns 0 on success, a variety of ints on failure
printf("stat() of directory %s failed with exit code: %d ",
prefixdir, staterr);
return(BADSTAT);
}
} else { // no commandline args given
printf("A data directory path argument is required. ");
return(NOARGS);
} // end directory arg validation
AB_Logger mylogger(prefixdir); // create a new logging object
// Now write some tests using the mylogger object!
return SUCCESS;
}
Write a messaging class in C++ that supports logging information to flat text files in the filesystem. Note: use the files below: AB_Logger.cpp and AB_Logger.h 1. Create a constructor that takes a Character string that represents a file path prefix and returns a reference to itself If instantiated correctly, else NULL 2. Create a method logAccess() that returns an integer and takes a single Character string argument named "message". The method should write the contents of message to a log file filepathprefix/accessLog.txt, account for situations if the file cannot be written to the file system, and returns 0 on success, -1 on failure. 3. Create a method logUserMessage() that returns an integer and takes the Character string arguments "destinationuser", "sendinguser", and "message" that: a) Writes the string "from (sendingUser): (message)" to a file at: pathprefix/messages/destinationuser/YYYYMMDD_HHMMSS_X.txt where 'YYYYMMDD_HHMMSS' is year,month,day_hour,minutes, seconds such as 20210125_132758 and 'X' is an integer from 0. b) If the file already exists, increment the value of X until a message can be written. This will reduce collisions on a per-second basis. The file doesn't exist, the file is created and information written to the file. c) Accounts for situations if the file cannot be written to the file system and returns O on success, -1 on failure. AB_Logger.cpp File * Method definitions for file-based logging class AB_Logger */ #include "AB_Logger.h" *** Class constructor requires path ********/ SE_Files Logger::AB_Logger(char *pathprefix) { } // end AB_Logger constructor // Other class methods below here AB_Logger.h File #pragma once //#include
#include #include #include *** /**** * Class specification, includes method prototypes * and public/private member variable defs *******/ class AB_Logger { *** public: /* Constructor: Instantiate a logging object bound to the given filesystem path prefix. Return pointer to self or NULL AB_Logger(char *pathprefix); private: }; // end AB_Logger class spec Wrapper Programs Use the file AB-Lab1-tests.cpp that is used as a wrapper program for your AB_Logger class. This is accomplished through the use of main() in the file to instantiate the class and test functionality by calling its methods. 1. Edit CMakelists.txt such that your class code and the test wrapper build into an executable file. 2. Call logAccess() and test both success and failure conditions. 3. Call logUserMessage() and test both success and failure conditions CMakeList.txt File #CMakelist.txt : CMake project for CMakeProject2, include source and define # project specific logic here. cmake_minimum required (VERSION 3.8) project ("AB-Lab1Messaging") add_library(AB-LoggingLib "AB_Logger.cpp" "AB_Logger.h") ## Add source to this project's executable. add_executable (AB-Lab1-tests "AB-Lab1-tests.cpp") ## TODO: Add tests and install targets if needed. ## Assemble the tests and library target_link_libraries (AB-Lab1-tests AB-LoggingLib) AB-Lab1-tests.cpp File /* Test wrapper w/ main for logging files in Labi */ #include #include #include #ifdef _linux_ #include #endif #ifdef _WIN32 #include #endir #ifdef _0SX_ #include #endif #include "AB_Logger.h" #define SUCCESS 0 #define NOARGS -1 #define BADSTAT -2 int main(int args, char **argv) { char *prefixdir = NULL; struct stat dirstats; int staterr = 0; /* Data directory from very simplistic commandline argument, if given */ if (argc > 1) { prefixdir = argv[1]; staterr = stat(prefixdir, &dirstats); // If we can stat it, it exists if (staterr) { // stat returns on success, a variety of ints on failure printf("stat() of directory %s failed with exit code: %d ", prefixdir, staterr); return(BADSTAT); } } else { // no commandline args given printf("A data directory path argument is required. "); return(NOARGS); } // end directory arg validation AB_Logger mylogger(prefixdir); // create a new logging object // Now write some tests using the mylogger object! return SUCCESS; Write a messaging class in C++ that supports logging information to flat text files in the filesystem. Note: use the files below: AB_Logger.cpp and AB_Logger.h 1. Create a constructor that takes a Character string that represents a file path prefix and returns a reference to itself If instantiated correctly, else NULL 2. Create a method logAccess() that returns an integer and takes a single Character string argument named "message". The method should write the contents of message to a log file filepathprefix/accessLog.txt, account for situations if the file cannot be written to the file system, and returns 0 on success, -1 on failure. 3. Create a method logUserMessage() that returns an integer and takes the Character string arguments "destinationuser", "sendinguser", and "message" that: a) Writes the string "from (sendingUser): (message)" to a file at: pathprefix/messages/destinationuser/YYYYMMDD_HHMMSS_X.txt where 'YYYYMMDD_HHMMSS' is year,month,day_hour,minutes, seconds such as 20210125_132758 and 'X' is an integer from 0. b) If the file already exists, increment the value of X until a message can be written. This will reduce collisions on a per-second basis. The file doesn't exist, the file is created and information written to the file. c) Accounts for situations if the file cannot be written to the file system and returns O on success, -1 on failure. AB_Logger.cpp File * Method definitions for file-based logging class AB_Logger */ #include "AB_Logger.h" *** Class constructor requires path ********/ SE_Files Logger::AB_Logger(char *pathprefix) { } // end AB_Logger constructor // Other class methods below here AB_Logger.h File #pragma once //#include #include #include #include *** /**** * Class specification, includes method prototypes * and public/private member variable defs *******/ class AB_Logger { *** public: /* Constructor: Instantiate a logging object bound to the given filesystem path prefix. Return pointer to self or NULL AB_Logger(char *pathprefix); private: }; // end AB_Logger class spec Wrapper Programs Use the file AB-Lab1-tests.cpp that is used as a wrapper program for your AB_Logger class. This is accomplished through the use of main() in the file to instantiate the class and test functionality by calling its methods. 1. Edit CMakelists.txt such that your class code and the test wrapper build into an executable file. 2. Call logAccess() and test both success and failure conditions. 3. Call logUserMessage() and test both success and failure conditions CMakeList.txt File #CMakelist.txt : CMake project for CMakeProject2, include source and define # project specific logic here. cmake_minimum required (VERSION 3.8) project ("AB-Lab1Messaging") add_library(AB-LoggingLib "AB_Logger.cpp" "AB_Logger.h") ## Add source to this project's executable. add_executable (AB-Lab1-tests "AB-Lab1-tests.cpp") ## TODO: Add tests and install targets if needed. ## Assemble the tests and library target_link_libraries (AB-Lab1-tests AB-LoggingLib) AB-Lab1-tests.cpp File /* Test wrapper w/ main for logging files in Labi */ #include #include #include #ifdef _linux_ #include #endif #ifdef _WIN32 #include #endir #ifdef _0SX_ #include #endif #include "AB_Logger.h" #define SUCCESS 0 #define NOARGS -1 #define BADSTAT -2 int main(int args, char **argv) { char *prefixdir = NULL; struct stat dirstats; int staterr = 0; /* Data directory from very simplistic commandline argument, if given */ if (argc > 1) { prefixdir = argv[1]; staterr = stat(prefixdir, &dirstats); // If we can stat it, it exists if (staterr) { // stat returns on success, a variety of ints on failure printf("stat() of directory %s failed with exit code: %d ", prefixdir, staterr); return(BADSTAT); } } else { // no commandline args given printf("A data directory path argument is required. "); return(NOARGS); } // end directory arg validation AB_Logger mylogger(prefixdir); // create a new logging object // Now write some tests using the mylogger object! return SUCCESS