Question
Above is my instructions, below is my code. Can someone just double check me and ensure that if I 100000 in the coding to Adjust
Above is my instructions, below is my code. Can someone just double check me and ensure that if I "100000" in the coding to "Adjust your array sizes to hold only 1,000 elements and run the 9 tests again" that it will in fact meet what I the instructions are asking. I'd like the outputs to automatically line up, but I think the way I coded it, it wont ever truly line up. Is it an easy fix?
import java.util.Arrays;
import java.util.Random;
public class SelectionSort
{
static Random r=new Random();
public static void main(String args[])
{
System.out.println("sort\t\t\t best case\t\t average case\t\t worst case");
SelectionSortTest();
InsertionSortTest();
MergeSortTest();
}
public static void SelectionSortTest()
{
long starttime;
long endtime;
starttime=System.nanoTime();
selectionSort(getSequentialArray());
endtime=System.nanoTime();
long best=endtime-starttime;
starttime=System.nanoTime();
selectionSort(getRandomArray());
endtime=System.nanoTime();
long average=endtime-starttime;
starttime=System.nanoTime();
selectionSort(getDescendingArray());
endtime=System.nanoTime();
long worst=endtime-starttime;
System.out.println(String.format("selection sort\t\t%d\t\t%d\t\t%d",best,average,worst));
}
public static void InsertionSortTest()
{
long starttime;
long endtime;
starttime=System.nanoTime();
insertionSort(getSequentialArray());
endtime=System.nanoTime();
long best=endtime-starttime;
starttime=System.nanoTime();
insertionSort(getRandomArray());
endtime=System.nanoTime();
long average=endtime-starttime;
starttime=System.nanoTime();
insertionSort(getDescendingArray());
endtime=System.nanoTime();
long worst=endtime-starttime;
System.out.println(String.format("Insertion sort\t\t%d\t\t\t%d\t\t%d",best,average,worst));
}
public static void MergeSortTest()
{
long starttime;
long endtime;
starttime=System.nanoTime();
MergeSort(getSequentialArray());
endtime=System.nanoTime();
long best=endtime-starttime;
starttime=System.nanoTime();
MergeSort(getRandomArray());
endtime=System.nanoTime();
long average=endtime-starttime;
starttime=System.nanoTime();
MergeSort(getDescendingArray());
endtime=System.nanoTime();
long worst=endtime-starttime;
System.out.println(String.format("Merge sort\t\t%d\t\t\t%d\t\t%d",best,average,worst));
}
public static int[] getSequentialArray()
{
int[] a=new int[100000];
for(int i=0;i { a[i]=i+1; } return a; } public static int[] getRandomArray() { int[] a=new int[100000]; for(int i=0;i { a[i]=r.nextInt(100000 + 1 - 1) + 1; } return a; } public static int[] getDescendingArray() { int[] a=new int[100000]; int j=1000; for(int i=0;i { a[i]=j; j--; } return a; } public static void insertionSort(int[] data) { // loop over data.length - 1 elements for (int next = 1; next { int insert = data[next]; // value to insert int moveItem = next; // location to place element // search for place to put current element while (moveItem > 0 && data[moveItem - 1] > insert) { // shift element right one slot data[moveItem] = data[moveItem - 1]; moveItem--; } data[moveItem] = insert; // place inserted element } } public static void selectionSort(int[] data) { // loop over data.length - 1 elements for (int i = 0; i { int smallest = i; // first index of remaining array // loop to find index of smallest element for (int index = i + 1; index if (data[index] smallest = index; swap(data, i, smallest); // swap smallest element into position } } // end method selectionSort // helper method to swap values in two elements private static void swap(int[] data, int first, int second) { int temporary = data[first]; // store first in temporary data[first] = data[second]; // replace first with second data[second] = temporary; // put temporary in second } private static int[] numbers; private static int[] helper; private static int number; public static void MergeSort(int[] values) { numbers = values; number = values.length; helper = new int[number]; mergesort(0, number - 1); } private static void mergesort(int low, int high) { // check if low is smaller than high, if not then the array is sorted if (low { // Get the index of the element which is in the middle int middle = low + (high - low) / 2; // Sort the left side of the array mergesort(low, middle); // Sort the right side of the array mergesort(middle + 1, high); // Combine them both merge(low, middle, high); } } private static void merge(int low, int middle, int high) { // Copy both parts into the helper array for (int i = low; i { helper[i] = numbers[i]; } int i = low; int j = middle + 1; int k = low; // Copy the smallest values from either the left or the right side back // to the original array while (i { if (helper[i] { numbers[k] = helper[i]; i++; } else { numbers[k] = helper[j]; j++; } k++; } // Copy the rest of the left side of the array into the target array while (i { numbers[k] = helper[i]; k++; i++; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
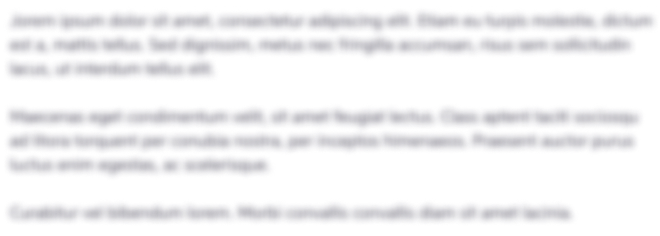
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started