Answered step by step
Verified Expert Solution
Question
1 Approved Answer
/** * Abstract Class: Employee * * Represents an employee for payroll calculations. All calculations are based on an * hourly wage. Therefore, for salaried
/** * Abstract Class: Employee *
* Represents an employee for payroll calculations. All calculations are based on an * hourly wage. Therefore, for salaried employees, their annual salary must be * converted to an equivalent hourly wage. *
* @author Debra M. Duke * @version 2.0, updated 8/30/2012 * */ public abstract class Employee { /** * The employee's first name */ private String firstName; /** * The employee's last name */ private String lastName; /** * The hourly wage. For salaried employees, their annual salary must be * converted to an equivalent hourly wage. */ private double wage; /** * default contructor */ public Employee() { firstName = ""; lastName = ""; wage = 0.0; } /** * parameterized contructor * * @param first * @param last * @param hourlyWage */ public Employee(String first, String last, double hourlyWage) { firstName = first; lastName = last; wage = hourlyWage; } /** * calculates the weekly pay for this employee * * * @param hours the number of hours worked for the week * @return the pay amount based onhours
*/ public abstract double computePay(double hours); /** * @return the first name */ public String getFirstName(){ return firstName; } /** * @return the last name */ public String getLastName(){ return lastName; } /** * @return the full name */ public String getName(){ return lastName + ", " + firstName; } /** * @return the wage */ public double getWage(){ return wage; } /** * @param name the first name to set */ public void setFirstName(String name){ firstName = name; } /** * @param name the last name to set */ public void setLastName(String name){ lastName = name; } /** * The wage must be in the form of the hourly wage. Convert all * other forms of salary compensation into an hourly equivalent before setting this * value. * @param wage the wage to set */ public void setWage(double wage){ this.wage = wage; } /** * sets the wage to the new amount calculated * by increasing the existing wage by the percent * increase * * @param percent the percent increase */ public void raiseWages(double percent){ wage = wage * ((100 + percent)/100); } }
Project 2 - Personnel (1) converted (Protected View) - Word Sign in File Home Insert Design Layout References Mailings Review View Help Grammarly Foxit Reader PDF Tell me what you want to do PROTECTED VIEW Be careful--files from the Internet can contain viruses. Unless you need to edit, it's safer to stay in Protected View Enable Editing CSC 202 Computer Science II - Project 2 CSC 202 Computer Science II - Project 2 CSC ZOZ Computer Science II - Project 2 Programming Assignment z $20 $2 125 . / 63/ / wed e-po e goed h de et them the power en ha rwaltherubahan words W adhamiration the com weathe r edemple . Le tre of the can Honda hellery and they had y es Yound the war m thadi hetype the e Here are a fe w more Ar c imelise them The treet serta te your mot cake foo d the and Updated world wherwisem when a ted TON, Lisa et a bfoluen a wide www . Endo sh cathew was e 3 of 3 1083 words Type here to search BE ^E o de Q ES - E 7:12 PM ( 640) ENG 2/10/200 CSC 202 Computer Science II - Project 2 Programming Assignment 2 Note: When you turn in on assignment to be graded in this class, you are making the claim that you neither gave nor received assistance on the work you turned in (except, of course, assistance from the instructor). Program: Points: Personnel 100 Write a program named Project that maintains wage information for the employees of a company. The company maintains a text file of all of the current employees called Employeesin.dat. Each line of the file contains the employee's name (last name, first name), a character code indicating their wage status (h for hourly or s for salary), and their wage (pay/hour for hourly employees and the annual salary for salary employees). The name, wage status and wage are separated by varying amounts of white space (at minimum, there are two spaces). For example, Harris, Joanh 5.00 Baird, Joey h 7.50 Kinsey, Paul h 8.00 Olson, Margaret S 15000 Campbell, Peter S 20000 Draper, Donalds 40000 Sterling, Rogers 45000 Cooper, Bertram S 50000 Once a week (generally on Friday), updates to the file (Employeesin.dat) are made and the weekly paycheck amount is calculated for each employee based on the hours worked that week. The update information is read from a second text file called Updates.dat. Updates can be any of the following: adding a new employee (n), raising the rate of pay for all employees by a given percentage (r), or dismissal of an existing employee (d). For example, the file could contain the following lines: Pryce, Lane S 40000 Kinsey After performing the updates, the revised information is written to a text file named EmployeesOut.dat a summary report is displayed on the console in the following format, New Employee added: Pryce, Lane New Wages: Harris, Joan $5.25/hour Baird, Joey $7.88/hour Olson, Margaret $15750.00/year Campbell, Peter $21000.00/year CSC 202 Computer Science II - Project 2 Draper, Donald $42000.00/year Sterling, Roger $47250.00/year Cooper, Bertram $52500.00/year Pryce, Lane $42000.00/year Deleted Employee: Kinsey, Paul Paycheck amounts are calculated based on each employee's number of hours worked. This information is included in the file Hours Worked.dat. This file simply lists each employee's last name and the number of hours worked that week, separated by white space. For example, Harris 65 Baird 40 Olson 70 Campbell 40 Draper 60 Sterling 40 Cooper 35 Pryce 45 After calculating each employee's pay for the week, a report is written to an output file called WeeklyPayroll.txt in the following format, Paycheck amount: Harris, Joan $406.88 Baird, Joey $315.00 Olson, Margaret $302.88 Campbell, Peter $403.85 Draper, Donald $807.69 Sterling, Roger $908.65 Cooper, Bertram $1009.62 Pryce, Lane $807.69 Total $4962.26 (Please note that all the examples shown are for formatting purposes only; do not expect that the numeric values reflect correct calculations in any way.) Write two classes that store information about an employee: HourlyEmployee, which extends the abstract class Employee. The constructor will take an employee's name (first name and last name are two separate data fields) and hourly wage as its parameters. Methods will include computePay and toString. To determine the employee's pay computePay multiplies the first 40 hours (or fewer) by the employee's hourly wage. Hours worked beyond 40 are paid at time-and-a-half (1.5 times the hourly wage) toString() returns a string containing the employee's name and hourly wage, formatted as shown in the example output of the r command. Note that spaces are added between the names and wage so that the entire string is 40 characters long. CSC 202 Computer Science II - Project 2 SalariedEmployee, which extends the abstract class Employee. The constructor will take the employee's name (first name and last name are two separate data fields) and annual salary as its parameters. Methods will include a getter and a setter for the annual salary, along with computePay and toString(). (Note that the annual salary must be converted to an hourly wage, because that's what the Employee class requires. To do this conversion, assume that a salaried employee works 40 hours a week for 52 weeks.) computePay always returns 1/52 of the annual salary, regardless of the number of hours worked, toString() returns a string containing the employee's name and annual salary, formatted as shown in the example output for the r command. (Do not add a separate instance variable for the annual salary. Follow the program specifications in order to receive full credit for this project.) Use an array to store employee records of data type Employee. The array should be managed in a class called Personnel Manager. Each element of the array will store a reference to either an HourlyEmployee object or a SalariedEmployee object. The array used to store employee objects must contain only one element initially. When the array becomes full, it must be doubled in size by calling a method written for this purpose in the Personnel Manager class. Your main method is located in the Project2 class, which is responsible for calling its own methods to handle reading and writing the data files. It instantiates a Personnel Manager object in order to process the employee updates and generate the weekly payroll. Here are a few other requirements for the program: . After updating the employee records and calculating the weekly payroll, the revised employee information is to be stored in the same format as the original Employeesin.dat file. However, name this output file EmployeesOut.dat. Dollar amounts must be displayed correctly, with two digits after the decimal point. For example, make sure that your program displays $100.00 not $100.0 All input may be preceded or followed by spaces. Character command codes may be uppercase or lowercase letters. If the user enters a character code other than n, d, h, s, or r, the program must display the following message along with the line containing the invalid character: Command was not recognized;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
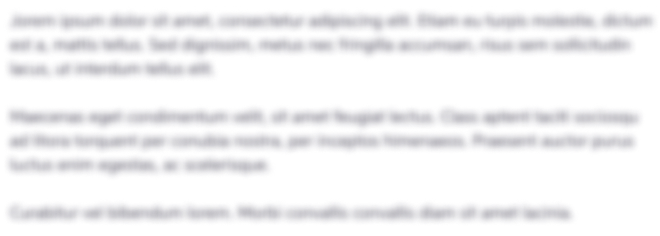
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started