Question
Activity Euchre project Euchre class constants Constants class Add the following constants int ONE = 0 int TWO = 1 int NUM_PLAYERS = 4 int
Activity | |
Euchre project | |
Euchre class | |
constants | |
Constants class | Add the following constants int ONE = 0 int TWO = 1 int NUM_PLAYERS = 4 int DEAL_ONE = 2 int DEAL_TWO = 3 |
core package | |
AiPlayer class | |
Card class | |
Deck class | Update class to If not already included, generate getter/setter for member variable of data type Set that represents the deck of cards for the game |
Game class | Update class to Add member variablesModify member variable named trump to be of data type class Card instead of enumeration Suit Add member variable of data type class ArrayList specifically to only allow for instances of class Player that represents the game table layout (i.e. players of the game Euchre are set up so that team members sit across from each other, therefore the deal would be similar to TeamOne.PlayerOne, TeamTwo.PlayerOne, TeamOne.PlayerTwo, TeamTwo.PlayerTwo depending upon who the dealer is) Add member variable of data type int to represent an integer index of the current dealer based on the table layout Add member variable of data type int to represent an integer index of the lead player based on the table layout Update custom constructor to call methods AFTER existing method callssetTable dealHand displayHands Write method setTable to do the following:Return type void Empty parameter list Instantiate the member variable that represents the player table that is of data type ArrayList Instantiate two instances of class Team set equal to each instance of class Team stored in the member variable of data type class ArrayList specifically of data type class Team Instantiate four instances of class Player set equal to each instance of class Player stored in the member variable in class Team of data type class ArrayList of data type class Player (i.e. players of the game Euchre are set up so that team members sit across from each other, therefore the deal would be similar to TeamOne.PlayerOne, TeamTwo.PlayerTwo, TeamOne.PlayerTwo, TeamTwo.PlayerTwo depending upon who the dealer is) There should be an instance of class Player that represents TeamOne, PlayerOne There should be an instance of class Player that represents TeamOne, PlayerTwo There should be an instance of class Player that represents TeamTwo, PlayerOne There should be an instance of class Player that represents TeamTwo, PlayerTwo Explicitly add each player to the member variable that represents the game table, from above in an alternating pattern so that a player from TeamOne is added, followed by a player from TeamTwo, followed by a player from TeamOne, followed by a player from TeamTwo; this requires using the method signature of class ArrayList.add that requires two arguments; the first argument is the position in the ArrayList (i.e. 0, 1, 2, 3), the second argument is the object of data type class Player (e.g. table.add(0, teamOnePlayerOne) Write method setDealerAndLead to do the followingInstantiate an instance of class Random Set the member variable of primitive data type int that will represent a random integer of four players to determine who the initial dealer of the game is set equal to the method call .nextInt passing as an argument the value of the number of players in the game (i.e. four) Set the member variable that represents the current dealer equal to the randomly selected value from item f. above using the .get method in class ArrayList on the member variable that represents the Euchre table Write method dealHand to do the followingReturn type void Empty parameter list Call method setDealerAndLead Create a local variable of data type int to represent the current player index initialized to the leadIdx member variable Create a local variable of data type class Iterator explicitly only allowing for members of class Card set equal to member variable deck, calling method getDeck followed by method getIterator For the first deal of two cards each, loop through the number of playersCall method dealOne passing as arguments the variables player index and the iterator If the current player value is greater than 3, reset the variable to the value of 0; otherwise increment the current player For the second deal of three cards each, loop through the number of playersCall method dealTwo passing as arguments the variables player index and the iterator If the current player value is greater than 3, reset the variable to the value of 0; otherwise increment the current player Set the member variable trump equal to the next card on the deck Write method dealOne so it does the followingReturn type void Receives two parametersData type int representing the current player index Data type Iterator representing the iterator Loops twiceChecks if the iterator has a next CardInstantiate an instance of class Card set equal to method call next on the parameter iterator Outputs to the console the dealt card and the player it was dealt to Adds the card to the players hand by calling method getHand followed by method add, on the player instance stored at the parameter index location of member variable table Remove the current card from the HashSet using method call remove Write method dealTwo so it does the followingReturn type void Receives two parametersData type int representing the current player index Data type Iterator representing the iterator Loops thriceChecks if the iterator has a next CardInstantiate an instance of class Card set equal to method call next on the parameter iterator Outputs to the console the dealt card and the player it was dealt to Adds the card to the players hand by calling method getHand followed by method add, on the player instance stored at the parameter index location of member variable table Remove the current card from the HashSet using method call remove Write method displayHands to do the followingLoops through the game teamsCall method outputHands in class Team |
HumanPlayer class | |
IPlayer interface | |
Player class | Update class to Add member variable of data type class ArrayList specifically only allowing for instances of class Card that represents the players hand Generate getter/setter for the member variable above representing the players hand Write a customer constructor thatReceives no parameters Instantiates the member variable of type ArrayList representing the players hand Write method displayHandReturn type void Empty parameter list Using an enhanced for loop, loop through the hand of the playerOutput to the console the face and suit of each card in the players hand |
Team class | Update class to If it isnt already included, add member variable of data type class String to represent the teams name Generate getter/setter for member variable representing the teams name Write method outputHandsReturn type void Empty parameter list Using an enhanced for loop, loop through the collection of class TeamCall method outputHands for each player |
userinterface package |
Existing code::
/*
Constant class:
*/ package constants;
public class Constants { public static final int NUM_AI_PLAYERS = 3; public static final int NUM_CARDS = 24; public static final int CARDS_EACH = 5; public static final int MAX_ROUNDS = 5;
public enum Color { RED, BLACK } public enum Suit { CLUBS, DIAMONDS, HEARTS, SPADES } public enum Face { NINE, TEN, JACK, QUEEN, KING, ACE } }
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
/*
AiPlayer class :
*/
package core;
public class AiPlayer extends Player {
@Override public Card playCard() { throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public void makeTrump() { throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
}
/////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
/*
Card class:
*/
package core;
import constants.Constants.Color; import constants.Constants.Face; import constants.Constants.Suit;
public class Card { private Face face; private Suit suit; private Color color;
public int hashCode() { int hashcode = 0; hashcode += face.hashCode(); hashcode += suit.hashCode(); hashcode += color.hashCode(); return hashcode; } public boolean equals(Object obj) { if (obj instanceof Card) { Card card = (Card) obj; return (card.face.equals(this.face) && card.color.equals(this.color) && card.suit.equals(this.suit)); } else { return false; } } public Face getFace() { return face; }
public void setFace(Face face) { this.face = face; }
public Suit getSuit() { return suit; }
public void setSuit(Suit suit) { this.suit = suit; }
public Color getColor() { return color; }
public void setColor(Color color) { this.color = color; } }
///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
/*
Deck class
*/
package core;
import constants.Constants; import java.util.ArrayList; import java.util.Collections; import java.util.HashSet; import java.util.List; import java.util.Set;
public class Deck { private Set
if(suit == Constants.Suit.DIAMONDS || suit == Constants.Suit.HEARTS) card.setColor(Constants.Color.RED); else card.setColor(Constants.Color.BLACK);
if(!deck.contains(card)) deck.add(card); } } } private void displayDeck() { System.out.println("Deck size:" + deck.size() + " cards"); System.out.println("Deck includes:"); for(Card card : deck) { System.out.println("Card: " + card.getFace() + " of " + card.getSuit() + " is color " + card.getColor()); } } private void shuffleDeck() { List
public Set
public void setDeck(Set
}
//////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
/*
Game class
*/
package core;
import constants.Constants; import constants.Constants.Suit; import java.util.ArrayList; import java.util.Scanner;
public class Game { private Suit trump; private Player leadPlayer; private Player dealer; private Player wonTrick; private int round; private ArrayList
private void outputTeams() { for(Team team : teams) { team.outputTeam(); } } private void createDeck() { deck = new Deck(); } public Player getWonTrick() { return wonTrick; }
public void setWonTrick(Player wonTrick) { this.wonTrick = wonTrick; }
public int getRound() { return round; }
public void setRound(int round) { this.round = round; }
public Player getLeadPlayer() { return leadPlayer; }
public void setLeadPlayer(Player leadPlayer) { this.leadPlayer = leadPlayer; }
public Player getDealer() { return dealer; }
public void setDealer(Player dealer) { this.dealer = dealer; } }
//////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
/*
Human Player class
*/
package core;
/** * * @author kwhiting */ public class HumanPlayer extends Player { @Override public Card playCard() { throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
@Override public void makeTrump() { throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. } }
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
/*
IP player class
*/
package core;
/** * * @author kwhiting */ public interface IPlayer { public Card playCard(); public void makeTrump(); }
///////////////////////////////////////////////////////////////////////////////////
/*
Player class
*/
package core;
public abstract class Player implements IPlayer { private String name; private int tricks; private int bid; private int score;
public abstract Card playCard(); public abstract void makeTrump();
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public int getTricks() { return tricks; }
public void setTricks(int tricks) { this.tricks = tricks; }
public int getBid() { return bid; }
public void setBid(int bid) { this.bid = bid; }
public int getScore() { return score; }
public void setScore(int score) { this.score = score; } }
////////////////////////////////////////////////////////////////////////////
/*
Team Class
*/
package core;
import java.util.ArrayList;
public class Team { private ArrayList
public void outputTeam() { System.out.println(teamName + " includes players: "); for(Player player : team) { System.out.println(player.getName()); } }
public int getTeamScore() { return teamScore; }
public void setTeamScore(int teamScore) { this.teamScore = teamScore; }
public int getTeamTricks() { return teamTricks; }
public void setTeamTricks(int teamTricks) { this.teamTricks = teamTricks; }
public ArrayList
public void setTeam(ArrayList
public String getTeamName() { return teamName; }
public void setTeamName(String teamName) { this.teamName = teamName; } }
////////////////////////////////////////////////////////////
Step by Step Solution
There are 3 Steps involved in it
Step: 1
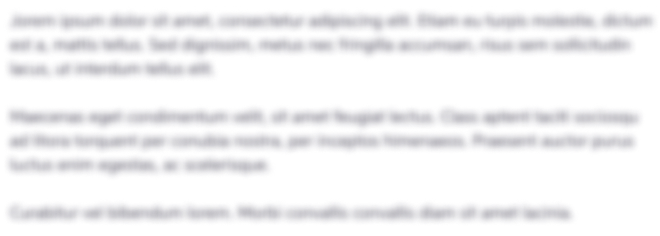
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started