Question
Add a constructor to your Clock class that takes a char representing a time zone code and sets the clock to the current time in
Add a constructor to your Clock class that takes a char representing a time zone code and sets the clock to the current time in that time zone.
Support codes 'p', 'm', 'c', 'e', 'a', 'h' for pacific, mountain, central, eastern, atlantic or Hawaii time zones.
Use time(0) (or time(NULL)) to get the current number of seconds since Jan 1 1970 . That's UTC by the way. Pacific time is currently minus eight hours from that.
Then extract the current time of day by modding by the number of seconds in a day.
ONLY USE time(0), not other library function or function calls, to get the time.
To determine whether or not it's daylight savings time you would need to figure out the year and current day or the year. Here are the start and end dates for the next four years:
2023 March 12 to November 5
2024March 10 to November 3
2025March 9 to November 2
2026 March 8 to November 1
Here's my code so far: // DRIVER
#include
int main() {
int hour;
Clock c1;
c1.setHour((time(0) / 3600) % 24 + 16);
if (hour = (time(0) - 8) < 0) hour += 16; else hour -= 8;
c1.setMinute((time(0) % 3600) / 60); c1.setSecond((time(0)) % 60);
for (int i = 0; i < 1000; i++) { c1.tick(); c1.printTime(false); cout << endl; c1.printTime(true); Sleep(1000); system("cls"); }
return 0; }
// IMPLEMENTATION
#include
Clock::Clock(int hour, int minute, int second, bool isMorning) : hour(hour), minute(minute), second(second), isMorning(isMorning) {}
int Clock::getHour() const { return hour; }
int Clock::getMinute() const { return minute; }
int Clock::getSecond() const { return second; }
bool Clock::getIsMorning() const { return isMorning; }
void Clock::setHour(int hour) {
if (hour <= 0) hour = 1; if (hour >= 24) hour = 23; this->hour = hour; }
void Clock::setMinute(int minute) {
if (minute >= 60) minute = 0; if (minute <= 0) minute = 0; this->minute = minute; }
void Clock::setSecond(int second) {
if (second >= 60) second = 0; if (second <= 0) second = 0; this->second = second; }
void Clock::setIsMorning(bool isMorning) { this->isMorning = isMorning; }
void Clock::tick() { second++;
if (second >= 60) { second = 0; minute++;
if (minute >= 60) { minute = 0; hour++;
if (hour >= 13) { hour = 1; isMorning = !isMorning; } } } }
void Clock::printTime(bool twelveHourFormat) const { int hourToPrint = hour; if (twelveHourFormat) { if (hour == 0) { hourToPrint = 12; } else if (hour > 12) { hourToPrint = hour - 12; } }
std::cout << hourToPrint << ":";
if (minute < 10) { std::cout << "0"; }
std::cout << minute << ":";
if (second < 10) { std::cout << "0"; }
std::cout << second;
if (isMorning) { if (hour >= 12) { cout << " PM"; } else if (hour <= 11) { cout << " AM";
} } }
// HEADER
#pragma once #ifndef CLOCK_H #define CLOCK_H
class Clock {
private: int hour; int minute; int second; bool isMorning;
public: Clock(int hour = 12, int minute = 0, int second = 0, bool isMorning = true); int getHour() const; int getMinute() const; int getSecond() const; bool getIsMorning() const;
void setHour(int hour); void setMinute(int minute); void setSecond(int second); void setIsMorning(bool isMorning);
void tick(); void printTime(bool twelveHourFormat) const; };
#endif CLOCK_H
Please provide constructor code.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
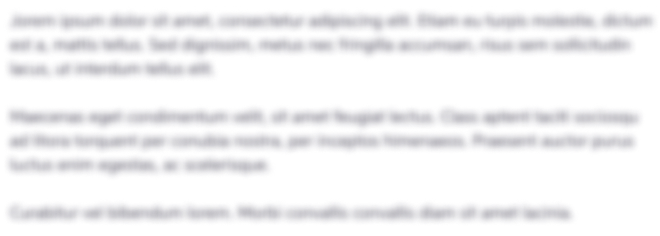
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started