Question
Add a difference method then demonstrate it in a main program, as specified in below book question. Included is Bag interface and implementation ArrayBag. The
Add a difference method then demonstrate it in a main program, as specified in below book question. Included is Bag interface and implementation ArrayBag.
The difference of two collections is a new collection of the entries that would be left in one collection after removing those that also occur in the second. Add a method difference to the interface BagInterface for the ADT bag that returns as a new bag the difference of the bag receiving the call to the method and the bag that is the methods one argument. Include sufficient comments to fully specify the method.
Note that the difference of two bags might contain duplicate items. For example, if object x occurs five times in one bag and twice in another, the difference of these bags contains x three times. Specifically, suppose that bag1 and bag2 are Bag objects, where Bag (or ArrayBag) implements BagInterface; bag1 contains the String objects a, b, and c; and bag2 contains the String objects b, b, d, and e. After the statement
BagInterface leftOver1 = bag1.difference(bag2);
executes, the bag leftOver1 contains the strings a and c. After the statement BagInterface leftOver2 = bag2.difference(bag1); executes, the bag leftOver2 contains the strings b, d, and e. Note that difference does not affect the contents of bag1 and bag2.
-----------------------------------------------
public interface BagInterface
{
public int getCurrentSize();
public boolean isEmpty();
public boolean add(T newEntry);
public T remove();
public boolean remove(T anEntry);
public void clear();
public int getFrequencyOf(T anEntry);
public boolean contains(T anEntry);
public void display();
}
-----------------
public final class ArrayBag
{
private final T[] bag;
private int numberOfEntries;
private boolean initialized = false;
private static final int DEFAULT_CAPACITY = 25;
private static final int MAX_CAPACITY = 10000;
/** Creates an empty bag whose initial capacity is 25. */
public ArrayBag()
{
this(DEFAULT_CAPACITY);
} // end default constructor
/** Creates an empty bag having a given capacity.
@param desiredCapacity The integer capacity desired. */
public ArrayBag(int desiredCapacity)
{
if (desiredCapacity <= MAX_CAPACITY)
{
// The cast is safe because the new array contains null entries
@SuppressWarnings("unchecked")
T[] tempBag = (T[])new Object[desiredCapacity]; // Unchecked cast
bag = tempBag;
numberOfEntries = 0;
initialized = true;
}
else
throw new IllegalStateException("Attempt to create a bag " +
"whose capacity exceeds " +
"allowed maximum.");
} // end constructor
/** Adds a new entry to this bag.
@param newEntry The object to be added as a new entry.
@return True if the addition is successful, or false if not. */
public boolean add(T newEntry)
{
checkInitialization();
boolean result = true;
if (isArrayFull())
{
result = false;
}
else
{ // Assertion: result is true here
bag[numberOfEntries] = newEntry;
numberOfEntries++;
} // end if
return result;
} // end add
/** Retrieves all entries that are in this bag.
@return A newly allocated array of all the entries in this bag. */
public T[] toArray()
{
checkInitialization();
// The cast is safe because the new array contains null entries.
@SuppressWarnings("unchecked")
T[] result = (T[])new Object[numberOfEntries]; // Unchecked cast
for (int index = 0; index < numberOfEntries; index++)
{
result[index] = bag[index];
} // end for
return result;
} // end toArray
public boolean isEmpty()
{
return numberOfEntries == 0;
} // end isEmpty
public int getCurrentSize()
{
return numberOfEntries;
}
public int getFrequencyOf(T anEntry)
{
checkInitialization();
int counter = 0;
for (int index = 0; index < numberOfEntries; index++)
{
if (anEntry.equals(bag[index]))
{
counter++;
} // end if
} // end for
return counter;
}
public boolean contains(T anEntry)
{
checkInitialization();
return getIndexOf(anEntry) > -1; // or >= 0
} // end contains
/** Removes all entries from this bag. */
public void clear()
{
while (!isEmpty())
remove();
} // end clear
/** Removes one unspecified entry from this bag, if possible.
@return Either the removed entry, if the removal
was successful, or null. */
public T remove()
{
checkInitialization();
T result = removeEntry(numberOfEntries - 1);
return result;
}
public boolean remove(T anEntry)
{
checkInitialization();
int index = getIndexOf(anEntry);
T result = removeEntry(index);
return anEntry.equals(result);
} // end remove
// Returns true if the array bag is full, or false if not.
private boolean isArrayFull()
{
return numberOfEntries >= bag.length;
} // end isArrayFull
// Locates a given entry within the array bag.
// Returns the index of the entry, if located,
// or -1 otherwise.
// Precondition: checkInitialization has been called.
private int getIndexOf(T anEntry)
{
int where = -1;
boolean found = false;
int index = 0;
while (!found && (index < numberOfEntries))
{
if (anEntry.equals(bag[index]))
{
found = true;
where = index;
} // end if
index++;
} // end while
// Assertion: If where > -1, anEntry is in the array bag, and it
// equals bag[where]; otherwise, anEntry is not in the array.
return where;
} // end getIndexOf
// Removes and returns the entry at a given index within the array.
// If no such entry exists, returns null.
// Precondition: 0 <= givenIndex < numberOfEntries.
// Precondition: checkInitialization has been called.
private T removeEntry(int givenIndex)
{
T result = null;
if (!isEmpty() && (givenIndex >= 0))
{
result = bag[givenIndex]; // Entry to remove
int lastIndex = numberOfEntries - 1;
bag[givenIndex] = bag[lastIndex];
bag[lastIndex] = null; // Remove reference to last
numberOfEntries--;
} // end if
return result;
} // end removeEntry
public void display()
{
for(int i=0; i< numberOfEntries; i++)
System.out.print(bag[i] + " ");
System.out.println();
}
// Throws an exception if this object is not initialized.
private void checkInitialization()
{
if (!initialized)
throw new SecurityException("ArrayBag object is not initialized properly.");
} // end checkInitialization
} // end ArrayBag
Step by Step Solution
There are 3 Steps involved in it
Step: 1
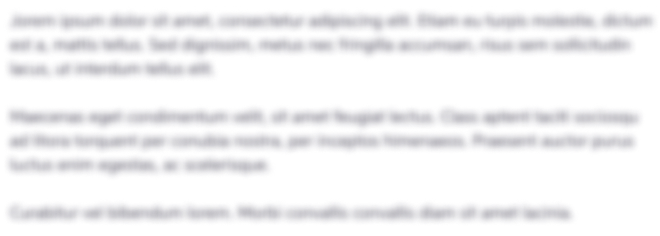
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started