Question
Add a do while loop around your initial menu and switch statement that you created for the Chapter 4 Assignment. Have your do while loop
Add a do while loop around your initial menu and switch statement that you created for the Chapter 4 Assignment. Have your do while loop run until the user selects the menu option to quit. Remove the explanations of the necessity to run the program again from your program options, since we know how to loop now.
For your case 1 and case 2 options, use a while loop to repeat the action until the user enters N or n. E. g.: Print out the menu for case 1 and prompt for the weekday; after this, prompt the user to see it again with a while loop; if the user does not select Y, go back to the main menu in the do while loop. For case 2, calculate the bill, write it to a file, and prompt the user to calculate another bill. Make sure you reset everything to 0 so your total doesnt reflect a previously calculated bill.
#include
#include
#include
#include
#include
using namespace std;
int main()
{
//declairing the values for the math
float billing_amount;
const double tax_rate = .09;
float tip_rate;
float tax_amount;
float tip_amount;
float total;
//random table values
const unsigned MAX = 20;
const unsigned MIN = 1;
unsigned seed = time(0), table;
srand(seed);
//store name
string const store = "John Foods";
string name;
cout << right;
cout << store << endl << endl;
//Main menu
int choice;
cout << " __________________________ ";
cout << "| Main Menu | ";
cout << "|--------------------------| ";
cout << "|1) Display Food Menu | ";
cout << "|2) Calculate Bill | ";
cout << "|3) Exit | ";
cout << "|--------------------------| ";
cout << "Enter your choice (1 - 3): ";
cin >> choice;
//switch 1 start
switch (choice)
{
case 1:
cout << " You selected the food menu! ";
cout << endl;
cout << "If you dont want the food menu, You will"< cout << "have to re-run the program!" << endl; //Discounts for the week //chosing a day of the week cout << " What day of the week is it? "; cout << " __________________________ "; cout << "| What Day is it? | "; cout << "|--------------------------| "; cout << "|1) Monday | "; cout << "|2) Tuesday | "; cout << "|3) Wednsday | "; cout << "|4) Thursday | "; cout << "|5) Friday | "; cout << "|6) Saturday | "; cout << "|7) Sunday | "; cout << "|--------------------------| "; cout << "Enter your choice (1 - 7): "; cin >> choice; //switch 2 start switch (choice) { case 1: cout << " Half price chicken on Monday "; break; case 2: cout << " $.50 off beans on Tuesday "; break; case 3: cout << " All you can eat salad on Wednsday "; break; case 4: cout << " Buy one get one side for free on Thursday "; break; case 5: cout << " Regular Prices on Friday "; break; case 6: cout << " Regular Prices on Saturday "; break; case 7: cout << " Regular Prices on Sunday "; break; default: cout << " You did not select a valid choice! "; break; } cout << endl; cout << "If you chose the wrong day," << endl; cout << "you have to re-run the program!" << endl; //switch 2 stop //food menu cout << " __________________________ "; cout << "| Food Menu | "; cout << "|--------------------------| "; cout << "|1) Fried Chicken | "; cout << "|2) Beans | "; cout << "|3) Potatos | "; cout << "|4) Corn | "; cout << "|5) Stake | "; cout << "|6) Ribs | "; cout << "|7) Salad | "; cout << "|--------------------------| "; cout << "Enter your choice (1 - 7): "; cin >> choice; //switch 3 start switch (choice) { case 1: cout << " You selected the Fried Chicken: $5.00 "; cout << endl; cout << "If you want to calculate the bill," << endl; cout << "you have to re-run the program!" << endl; cout << endl; break; case 2: cout << " You selected Beans: $2.00 "; cout << endl; cout << "If you want to calculate the bill," << endl; cout << "you have to re-run the program!" << endl; cout << endl; break; case 3: cout << " You selected Potatos: :$2.00 "; cout << endl; cout << "If you want to calculate the bill," << endl; cout << "you have to re-run the program!" << endl; cout << endl; break; case 4: cout << " You selected Corn: $1.50 "; cout << endl; cout << "If you want to calculate the bill," << endl; cout << "you have to re-run the program!" << endl; cout << endl; break; case 5: cout << " You selected Stake: $10.00 "; cout << endl; cout << "If you want to calculate the bill," << endl; cout << "you have to re-run the program!" << endl; cout << endl; break; case 6: cout << " You selected Ribs: $8.00 "; cout << endl; cout << "If you want to calculate the bill," << endl; cout << "you have to re-run the program!" << endl; cout << endl; break; case 7: cout << " You selected Salad: $3.00 "; cout << endl; cout << "If you want to calculate the bill," << endl; cout << "you have to re-run the program!" << endl; cout << endl; break; default: cout << " You did not select a valid choice! "; break; } //switch 3 stop break; case 2: cout << " You selected the bill calculator! "; cout< cout << "If you dont want the bill calculator, You will" << endl; cout << "have to re-run the program!" << endl; cout << endl; cout << endl; cout << "Bill Calculator" << endl; cout << endl; cout << "Enter servers name:" ""; cin.ignore(); getline(cin, name); cout << "Enter billing amount in dollars:" ""; cin >> billing_amount; cout << endl; cout << endl; cout << "Chose a tip rate amount" << endl; cout << endl; //Tip menu switch cout << " __________________________ "; cout << "| Tip Rates | "; cout << "|--------------------------| "; cout << "|1) 10% | "; cout << "|2) 20% | "; cout << "|3) 30% | "; cout << "|4) 40% | "; cout << "|5) 50% | "; cout << "|6) Other | "; cout << "|--------------------------| "; cout << "Enter your choice (1 - 6): "; cin >> choice; //switch 4 start switch (choice) { case 1: cout << " You selected The 10% Tip Rate "; tip_rate = 10; break; case 2: cout << " You selected The 20% Tip Rate "; tip_rate = 20; break; case 3: cout << " You selected The 30% Tip Rate "; tip_rate = 30; break; case 4: cout << " You selected The 40% Tip Rate "; tip_rate = 40; break; case 5: cout << " You selected The 50% Tip Rate "; tip_rate = 50; break; case 6: cout << " You selected The Custom Tip Rate "; cout << "Enter tip rate in percent:" ""; cin >> tip_rate; break; default: cout << " You did not select a valid choice! "; break; } //switch 4 stop //if statement for billing discount if (billing_amount >= 40 && tip_rate > 12) { cout << "You get a a 5 % discount on your meal" << endl; billing_amount*= .95; cout << endl; } else { billing_amount *= 1; } cout << endl; tax_amount = billing_amount * tax_rate; total = billing_amount + tax_amount; tip_amount = total * tip_rate / 100; total += tip_amount; cout << setprecision(2) << fixed; cout << "You entered the following information: " << endl; cout << "Billing amount= $" << setw(10) << billing_amount << endl; cout << "Tip rate= " << setw(10) << static_cast cout << endl; cout << "The tax rate is:" "" << setw(10) << static_cast cout << endl; cout << "Tip= $" << setw(10) << tip_amount << endl; cout << "Tax= $" << setw(10) << tax_amount << endl; cout << endl; cout << "Total amount due= $" << setw(10) << total << endl; cout << endl; cout << "Thanks for dinning at:" << endl; cout << store << endl; cout << endl; //random table code table = (rand() % (MAX - MIN + 1)) + MIN; cout << "Your table number is: " << table << endl; cout << endl; cout << "You were served by:" << " " << name << endl; cout << endl; cout << "Come back to see us soon!" "" << endl; cout << endl; char ch; cout << "Press any Key to continue:" "" << endl; cin.ignore(); cin.get(ch); cout< break; case 3: cout << " You selected exit! "; break; default: cout << " You did not select a valid choice! "; break; } //switch 1 stop return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
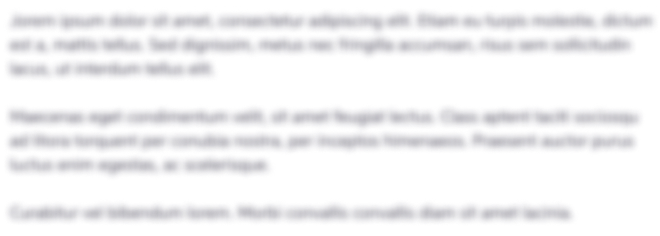
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started