Question
Add a due date to the Invoice application 1. Import the project named ch15 exLInvoice that's in the ex starts Then, review the code in
Add a due date to the Invoice application
1. Import the project named ch15 exLInvoice that's in the ex starts Then, review the code in the Invoice and InvoiceApp classes.
This is the invoice code
package murach.business;
import java.text.NumberFormat; import java.time.LocalDateTime; import java.time.format.DateTimeFormatter; import java.time.format.FormatStyle; import java.util.ArrayList;
public class Invoice {
// the instance variables private ArrayList lineItems; private LocalDateTime invoiceDate; // the constructor public Invoice() { lineItems = new ArrayList<>(); invoiceDate = LocalDateTime.now(); }
public void addItem(LineItem lineItem) { lineItems.add(lineItem); }
public ArrayList getLineItems() { return lineItems; }
public double getTotal() { double invoiceTotal = 0; for (LineItem lineItem : lineItems) { invoiceTotal += lineItem.getTotal(); } return invoiceTotal; }
public String getTotalFormatted() { NumberFormat currency = NumberFormat.getCurrencyInstance(); return currency.format(getTotal()); } public void setInvoiceDate(LocalDateTime invoiceDate) { this.invoiceDate = invoiceDate; }
2. In the Invoice class, modify the getlnvoiceDateFormatted method so it returns the due date using the MEDIUM format style 3. In the Invoice class, add a method named getDueDate. This method should calculate and retum a LocalDate Time object that's 30 days after the invoice 4. In the Invoice class, add a method named getDueDateFormatted. This method should retum the due date using the MEDIUM format style. 5. In the Invoice App class, modify the displaylnvoice method so it displays the invoice date and the due date before it displays the line items like this Invoice date july 1, 2015 Invoice due datet: Jul 31, 2015
Description Price QTY Total Murach's Java Programming $57.50 2 $115.00 Invoice total $115.00
this is the steps i Need .!! what you mean be productive
public LocalDateTime getInvoiceDate() { return invoiceDate; }
public String getInvoiceDateFormatted() { DateTimeFormatter dtf = DateTimeFormatter.ofLocalizedDate( FormatStyle.LONG); return dtf.format(invoiceDate); } }
and this is is the invoiceApp code
package murach.ui;
import murach.db.ProductDB; import murach.business.Invoice; import murach.business.LineItem; import murach.business.Product;
public class InvoiceApp {
public static Invoice invoice = new Invoice();
public static void main(String args[]) { System.out.println("Welcome to the Invoice application "); getLineItems(); displayInvoice(); }
public static void getLineItems() { String choice = "y"; while (choice.equalsIgnoreCase("y")) { String productCode = Console.getString("Enter product code: "); int quantity = Console.getInt("Enter quantity: ");
Product product = ProductDB.getProduct(productCode); invoice.addItem(new LineItem(product, quantity));
choice = Console.getString("Another line item? (y/n): "); System.out.println(); } }
public static void displayInvoice() { StringBuilder sb = new StringBuilder(); sb.append("Invoice date: "); sb.append(invoice.getInvoiceDateFormatted()); sb.append(" "); sb.append(StringUtil.pad("Description", 34)); sb.append(StringUtil.pad("Price", 10)); sb.append(StringUtil.pad("Qty", 5)); sb.append(StringUtil.pad("Total", 10)); sb.append(" ");
for (LineItem lineItem : invoice.getLineItems()) { Product product = lineItem.getProduct(); sb.append(StringUtil.pad(product.getDescription(), 34)); sb.append(StringUtil.pad(product.getPriceFormatted(), 10)); sb.append(StringUtil.pad(lineItem.getQuantityFormatted(), 5)); sb.append(StringUtil.pad(lineItem.getTotalFormatted(), 10)); sb.append(" "); } sb.append(" Invoice total: "); sb.append(invoice.getTotalFormatted()); sb.append(" "); System.out.println(sb); } }
2. In the Invoice class, modify the getlnvoiceDateFormatted method so it returns the due date using the MEDIUM format style 3. In the Invoice class, add a method named getDueDate. This method should calculate and retum a LocalDate Time object that's 30 days after the invoice 4. In the Invoice class, add a method named getDueDateFormatted. This method should retum the due date using the MEDIUM format style. 5. In the Invoice App class, modify the displaylnvoice method so it displays the invoice date and the due date before it displays the line items like this Invoice date july 1, 2015 Invoice due datet: Jul 31, 2015
Description Price QTY Total Murach's Java Programming $57.50 2 $115.00 Invoice total $115.00
6 Run the application to make sure it work correctly
here is the both classes codes
This is the invoice code
package murach.business;
import java.text.NumberFormat; import java.time.LocalDateTime; import java.time.format.DateTimeFormatter; import java.time.format.FormatStyle; import java.util.ArrayList;
public class Invoice {
// the instance variables private ArrayList lineItems; private LocalDateTime invoiceDate; // the constructor public Invoice() { lineItems = new ArrayList<>(); invoiceDate = LocalDateTime.now(); }
public void addItem(LineItem lineItem) { lineItems.add(lineItem); }
public ArrayList getLineItems() { return lineItems; }
public double getTotal() { double invoiceTotal = 0; for (LineItem lineItem : lineItems) { invoiceTotal += lineItem.getTotal(); } return invoiceTotal; }
public String getTotalFormatted() { NumberFormat currency = NumberFormat.getCurrencyInstance(); return currency.format(getTotal()); } public void setInvoiceDate(LocalDateTime invoiceDate) { this.invoiceDate = invoiceDate; }
public LocalDateTime getInvoiceDate() { return invoiceDate; }
public String getInvoiceDateFormatted() { DateTimeFormatter dtf = DateTimeFormatter.ofLocalizedDate( FormatStyle.LONG); return dtf.format(invoiceDate); } }
and this is is the invoiceApp code
package murach.ui;
import murach.db.ProductDB; import murach.business.Invoice; import murach.business.LineItem; import murach.business.Product;
public class InvoiceApp {
public static Invoice invoice = new Invoice();
public static void main(String args[]) { System.out.println("Welcome to the Invoice application "); getLineItems(); displayInvoice(); }
public static void getLineItems() { String choice = "y"; while (choice.equalsIgnoreCase("y")) { String productCode = Console.getString("Enter product code: "); int quantity = Console.getInt("Enter quantity: ");
Product product = ProductDB.getProduct(productCode); invoice.addItem(new LineItem(product, quantity));
choice = Console.getString("Another line item? (y/n): "); System.out.println(); } }
public static void displayInvoice() { StringBuilder sb = new StringBuilder(); sb.append("Invoice date: "); sb.append(invoice.getInvoiceDateFormatted()); sb.append(" "); sb.append(StringUtil.pad("Description", 34)); sb.append(StringUtil.pad("Price", 10)); sb.append(StringUtil.pad("Qty", 5)); sb.append(StringUtil.pad("Total", 10)); sb.append(" ");
for (LineItem lineItem : invoice.getLineItems()) { Product product = lineItem.getProduct(); sb.append(StringUtil.pad(product.getDescription(), 34)); sb.append(StringUtil.pad(product.getPriceFormatted(), 10)); sb.append(StringUtil.pad(lineItem.getQuantityFormatted(), 5)); sb.append(StringUtil.pad(lineItem.getTotalFormatted(), 10)); sb.append(" "); } sb.append(" Invoice total: "); sb.append(invoice.getTotalFormatted()); sb.append(" "); System.out.println(sb); } }
Here is the linetime class code
package murach.business;
import java.text.NumberFormat;
public class LineItem {
private Product product; private int quantity; private double total;
public LineItem() { this.product = new Product(); this.quantity = 0; this.total = 0; }
public LineItem(Product product, int quantity) { this.product = product; this.quantity = quantity; this.calculateTotal(); }
public void setProduct(Product product) { this.product = product; }
public Product getProduct() { return product; }
public void setQuantity(int quantity) { this.quantity = quantity; }
public int getQuantity() { return quantity; }
public String getQuantityFormatted() { NumberFormat number = NumberFormat.getNumberInstance(); return number.format(quantity); } public void setTotal(double total) { this.total = total; }
public double getTotal() { return total; }
public void calculateTotal() { total = quantity * product.getPrice(); }
public String getTotalFormatted() { NumberFormat currency = NumberFormat.getCurrencyInstance(); return currency.format(total); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
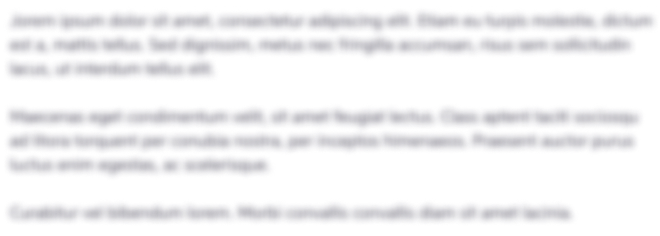
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started