Question
Add a Java class file named Message to Lab_Project_5. Design a class Message that models an e-mail message. A message has a recipient, a sender,
Add a Java class file named Message to Lab_Project_5.
Design a class Message that models an e-mail message. A message has a recipient, a sender, and a message text. Support the following methods: A constructor that is given and sets the sender and recipient; the message body is initialized to the empty string. A method append that appends a line of text to the message body A method toString that returns the message as one long string like this: "From: Harry Morgan To: Rudolf Reindeer . . ."
Copy the highlighted code below to your main method to test your Message class:
public static void main(String[] args) { Message msg1 = new Message("Harry Morgan", "Rudolf Reindeer"); msg1.append("Hello, how are you?"); msg1.append("Are you working on the assignment yet?"); msg1.append("If so, can we meet this weekend to discuss the assignment?"); msg1.append("Thanks for the help"); System.out.println(msg1.toString()); /* Expected output: From: Harry Morgan To: Rudolf Reindeer Hello, how are you? Are you working on the assignment yet? If so, can we meet this weekend to discuss the assignment? Thanks for the help */ } // end main method
Add a Java class file named Mailbox to Lab_Project_5.
Design a class Mailbox that stores e-mail messages, using the Message class of Lab 5.1. Implement the following methods:
public void addMessage(Message m) public int getNumberOfMessages() public Message getMessage(int position) public void removeMessage(int position)
Copy the highlighted code below to your main method (replace code from Lab 7.1) to test your Mailbox class:
public static void main(String[] args) { Mailbox box = new Mailbox(); // empty mailbox Message msg = new Message("Harry Morgan", "Rudolf Reindeer"); msg.append("Hello, how are you?"); msg.append("Are you working on the assignment yet?"); msg.append("If so, can we meet this weekend to discuss the assignment?"); msg.append("Thanks for the help"); box.addMessage(msg); msg = new Message("Harry Morgan", "Stella Clause"); msg.append("It's getting closer to the holidays"); msg.append("Want to do some shopping next weekend?"); msg.append("Let me know"); box.addMessage(msg); msg = new Message("Harry Morgan", "Esther Bunny"); msg.append("I'm out of eggs, and the stores are closed"); msg.append("Do you have any I can borrow?"); msg.append("Thanks a lot!"); box.addMessage(msg); int m = box.getNumberOfMessages(); for (int count=1; count<=m; count++) { Message M = box.getMessage(count); System.out.println(M.toString()); } /* Expected output: From: Harry Morgan To: Rudolf Reindeer Hello, how are you? Are you working on the assignment yet? If so, can we meet this weekend to discuss the assignment? Thanks for the help From: Harry Morgan To: Stella Clause It's getting closer to the holidays Want to do some shopping next weekend? Let me know From: Harry Morgan To: Esther Bunny I'm out of eggs, and the stores are closed Do you have any I can borrow? Thanks a lot! */ box.removeMessage(2); // remove second message added m = box.getNumberOfMessages(); for (int count=1; count<=m; count++) { Message M = box.getMessage(count); System.out.println(M.toString()); } /* Expected output: From: Harry Morgan To: Rudolf Reindeer Hello, how are you? Are you working on the assignment yet? If so, can we meet this weekend to discuss the assignment? Thanks for the help From: Harry Morgan To: Esther Bunny I'm out of eggs, and the stores are closed Do you have any I can borrow? Thanks a lot! */ } // end main method
Step by Step Solution
There are 3 Steps involved in it
Step: 1
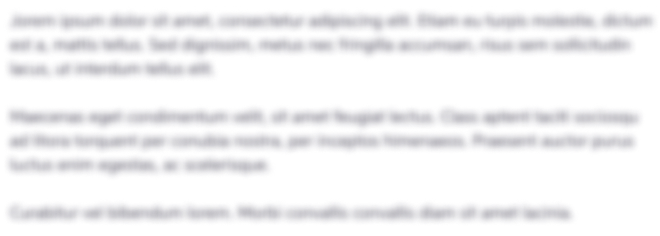
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started