Question
Student Edu My Drive Zoom Options Automatic Actual Size Fit Page Full Width 50% 75% 100% 125% 150% 200% 300% 400% Turn In ZS Select
Student
Edu
My Drive
Zoom Options Automatic Actual Size Fit Page Full Width 50% 75% 100% 125% 150% 200% 300% 400%
Turn In
ZS
Select
select_all
Dictionary
Text to Speech
Markup
Comment
Text Box
Equation
Drawing
Shapes
Eraser
Add Media
Signature
AP
Computer Science A
Basic Object-Oriented Programming
Student Handout
2019-2020 EDITION
Your name:
Presenter name:
Basic Object-Oriented Programming
A class is a blueprint from which objects are created. A class encapsulates an
objects state and behaviors. Defining a class creates a new data type.
The main parts of a class: class header, private instance variables, constructors, and
methods.
Private instance variables store data that describe the state of an object.
The job of the constructor is to initialize the instance variables. The constructor
is public and has the same name as the class. Unlike methods, a constructor
has no return type and is not void.
Methods represent behaviors of an object.
A class may contain constants which can be either public or private, depending
on whether or not a client needs access to the constant.
Only static methods or constants may be called using the class name.
Math.sqrt(16) is legal since sqrt is a static method in the Math class. Math.PI
is how a programmer would access the Math class constant PI.
Use an object name to call a method that is NOT static.
Dog frank = new Dog("Sparky", 0);
frank.makeOlder();
A class may have multiple constructors. When a class has more than one
constructor, the constructors are said to be overloaded. To overload a constructor,
the parameters in each constructor heading must vary in number, type, or order
from other constructors' headings.
A class can have multiple methods with the same name. When a class has more
than one method with the same name, these methods are said to be overloaded.
Overloaded methods, like overloaded constructors, are differentiated by their
parameters.
In a class, an accessor method is a method that does not change the state of the
object. Accessor methods generally return the value of a private instance variable.
In a class, a mutator method is a method that could or does change the state of the
object.
Every class inherits a toString method from its parent class, ultimately the Object
class. The toString method may be overridden to return pertinent information
about the object.
Copyright 2019 National Math + Science Initiative, Dallas, Texas. All rights reserved. Visit us online at www.nms.org. AP* is a trademark of the College Entrance
Examination Board. The College Entrance Examination Board was not involved in the production of this material. Used with permission.
AP
Computer Science A
Exercises.
1. Label these parts of the Dog class
accessor method(s)
class heading
constant(s)
constructor(s)
instance variable(s)
mutator method(s)
overloaded method(s)
overridden method(s)
public class Dog
{
private String name;
private int age;
public Dog(String initName, int initAge)
{
name = initName;
age = initAge;
}
public String getName()
{
return name;
}
public int getAge()
{
return age;
}
public void makeOlder()
{
age++;
}
public String toString()
{
return name + " is " + age + " years old.";
}
}
Copyright 2019 National Math + Science Initiative, Dallas, Texas. All rights reserved. Visit us online at www.nms.org. AP* is a trademark of the College Entrance
Examination Board. The College Entrance Examination Board was not involved in the production of this material. Used with permission.
AP
Computer Science A
2. Consider the CandyBar class and answer the questions that follow:
public class CandyBar {
private String name;
private double price;
private boolean kingSize;
public CandyBar() {
name = "Snickers";
price = 0.99;
kingSize = false;
}
public CandyBar(String n, double p, boolean k) {
name = n;
price = p;
kingSize = k;
}
public double getPrice()
{
return price; }
public String getName()
{
return name; }
public void changePrice(double newPrice)
{
price = newPrice; }
public double makeKing() {
kingSize = true;
price = price + 0.50;
return price;
}
}
a. How many private instance variables are there in the class? ________
b. How many constructors are there in the class?
________
c. How many accessor methods are there in the class?
________
d. How many mutator methods are there in the class?
________
e. When looking at a class, what distinguishes a constructor from ________
the methods of the class?
f. Look at all the methods in the CandyBar class and list all the ________
different return types.
g. What is the default price of a CandyBar object?
________
Copyright 2019 National Math + Science Initiative, Dallas, Texas. All rights reserved. Visit us online at www.nms.org. AP* is a trademark of the College Entrance
Examination Board. The College Entrance Examination Board was not involved in the production of this material. Used with permission.
loading...
AP
Computer Science A
Given the following incomplete class:
public class Binky
{
//private instance variables not shown
public Binky() {...}
public Binky(String name) {...}
public Binky(String name, int age) {...}
public String getName() {...}
public void setName(String name) {...}
public int getAge() {...}
public void setAge(int age) {...}
public String toString() {...}
//other methods not shown
}
5. Which one of the following statements would create a new Binky object using the
one parameter constructor?
a. Binky baby = Binky("Sally");
b. Binky baby = new Binky("Sally");
c. Binky = new Binky("Sally");
d. baby = Binky("Sally");
e. none of these
6. Which of the following statements would instantiate a new Binky object with a
given name Linus and an age of 0?
a. Binky baby = new Binky();
b. Binky baby = new Binky();
baby.setName("Linus");
baby.setAge(0);
c. Binky baby = new Binky("Linus");
baby.setAge(0);
d. Binky baby = new Binky("Linus",0);
e. none of these
Copyright 2019 National Math + Science Initiative, Dallas, Texas. All rights reserved. Visit us online at www.nms.org. AP* is a trademark of the College Entrance
Examination Board. The College Entrance Examination Board was not involved in the production of this material. Used with permission.
AP
Computer Science A
7. Given that baby has been instantiated as a Binky object, which of the following
statements would be an appropriate call to the getName method?
a. Binky.getName();
b. baby.getName();
c. baby.getName("Lucy");
d. System.out.println(baby.getName());
e. baby = getName();
8. Given that baby has been instantiated as a Binky object, which of the following
statements would be an appropriate call to the setName method?
a. Binky.setName("Lucy");
b. baby.setName();
c. baby.setName("Lucy");
d. System.out.println(baby.setName("Lucy"));
e. baby = setName("Lucy");
9. Assume that babyBoy and babyGirl have been instantiated as Binky objects.
Which of the following code segments would calculate the total of their ages?
I. int ageTotal = babyBoy + babyGirl;
II. int ageTotal = babyBoy.getAge();
ageTotal += babyGirl.getAge();
III. int ageTotal;
ageTotal = babyBoy.getAge() + babyGirl.getAge();
a. I only
b. II only
c. III only
d. I and II
e. II and III
Copyright 2019 National Math + Science Initiative, Dallas, Texas. All rights reserved. Visit us online at www.nms.org. AP* is a trademark of the College Entrance
Examination Board. The College Entrance Examination Board was not involved in the production of this material. Used with permission.
AP
Computer Science A
10. Consider the following classes that will be used to represent points in the
xy-coordinate plane.
public class Point {
private int x; // coordinates
private int y;
public Point() {
x = 0;
y = 0;
}
public Point(int a, int b){
x = a;
y = b;
}
// ... other methods not shown
}
public class NamedPoint extends Point {
private String name;
// constructors go here
// ... other methods not shown
}
Consider the following proposed constructors for the NamedPoint class.
I. public NamedPoint()
{
name = "";
}
II. public NamedPoint(int d1, int d2, String pointName)
{
x = d1; y = d2;
name = pointName;
}
III. public NamedPoint(int d1, int d2, String pointName)
{
super(d1, d2); name = pointName;
}
Which of these constructors would be legal for the NamedPoint class?
a. I only
b. II only
c. III only
d. I and III
e. II and III
Copyright 2019 National Math + Science Initiative, Dallas, Texas. All rights reserved. Visit us online at www.nms.org. AP* is a trademark of the College Entrance
Examination Board. The College Entrance Examination Board was not involved in the production of this material. Used with permission.
AP
Computer Science A
Free Response
An APLine is a line defined by the equation ax + by + c = 0, where a is not equal to zero, b is
not equal to zero, and a, b, and c are all integers. The slope of an APLine is defined to be the
double value -a / b. A point (represented by integers x and y) is on an APLine if the equation
of the APLine is satisfied when those x and y values are substituted into the equation. That
is, a point represented by x and y is on the line if ax + by + c is equal to 0.
Examples of two APLine equations are shown in the following table.
Equation
Slope (-a/b)
Is point (5, 2) on the line?
5x + 4y - 17 = 0
-5 / 4 = -1.25
Yes, because 5(5) + 4(-2) + (-17) = 0
-25x + 40y + 30 = 0 25 / 40 = 0.625
No, because -25(5) + 40(-2) + 30 0
Assume that the following code segment appears in a class other than APLine. The code
segment shows an example of using the APLine class to represent the two equations
shown in the table.
APLine line1 = new APLine(5, 4, -17);
double slope1 = line1.getSlope(); // slope1 is assigned -1.25
boolean onLine1 = line1.isOnLine(5, -2); // true because 5(5) + 4(-2) + (-17) = 0
APLine line2 = new APLine(-25, 40, 30);
double slope2 = line2.getSlope(); // slope2 is assigned 0.625
boolean onLine2 = line2.isOnLine(5, -2); // false because -25(5) + 40(-2) + 30 0
Write the APLine class. Your implementation must include a constructor that has three
integer parameters that represent a, b, and c, in that order. You may assume that the
values of the parameters representing a and b are not zero. It must also include a method
getSlope that calculates and returns the slope of the line, and a method isOnLine that
returns true if the point represented by its two parameters (x and y, in that order) is on
the APLine and returns false otherwise. Your class must produce the indicated results
when invoked by the code segment given above. You may ignore any issues related to
integer overflow.
Copyright 2019 National Math + Science Initiative, Dallas, Texas. All rights reserved. Visit us online at www.nms.org. AP* is a trademark of the College Entrance
Examination Board. The College Entrance Examination Board was not involved in the production of this material. Used with permission.
.
+
Page / 8
Step by Step Solution
There are 3 Steps involved in it
Step: 1
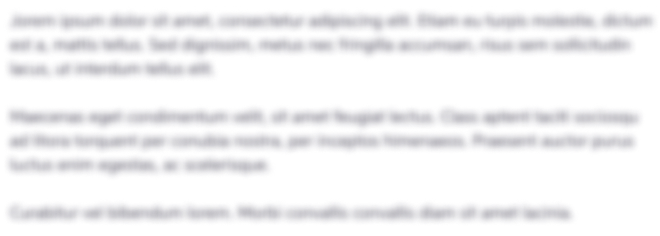
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started